For every
Web applicationa
ServletContextobject is created by the web container. ServletContext object is used to get configuration information from
Deployment Descriptor(web.xml) which will be available to any servlet or JSPs that are part of the web app.
对于每个
Web应用程序,Web容器都会创建一个
ServletContext对象。 ServletContext对象用于从
部署描述符(web.xml)获取配置信息,该信息对于Web应用程序中的任何Servlet或JSP都可用。
ServletContext的一些重要方法 (Some Important method of ServletContext)
Methods | Description |
---|---|
Object | returns the container attribute with the given name, or NULL if there is no attribute by that name. |
String | returns parameter value for the specified parameter name, or NULL if the parameter does not exist |
Enumeration | returns the names of the context's initialization parameters as an Enumeration of String objects |
void | set an object with the given attribute name in the application scope |
void | removes the attribute with the specified name from the application context |
方法 | 描述 |
---|---|
对象 | 返回具有给定名称的容器属性,如果该名称没有属性,则返回NULL。 |
字符串 | 返回指定参数名称的参数值;如果参数不存在,则返回NULL |
枚举 | 以字符串对象的枚举形式返回上下文的初始化参数的名称 |
无效 | 在应用程序范围内设置具有给定属性名称的对象 |
void | 从应用程序上下文中删除具有指定名称的属性 |
如何在web.xml中初始化上下文参数 (How Context Parameter is Initialized inside web.xml)
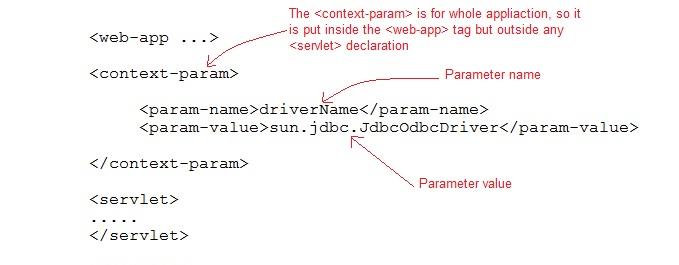
如何获取ServletContext的对象 (How to get the Object of ServletContext)
ServletContext app = getServletContext();OR
ServletContext app = getServletConfig().getServletContext();
ServletContext的优点 (Advantages of ServletContext)
-
Provides communication between servlets
提供Servlet之间的通信
-
Available to all servlets and JSPs that are part of the web app
适用于Web应用程序中的所有Servlet和JSP
-
Used to get configuration information from web.xml
用于从web.xml获取配置信息
上下文初始化参数和Servlet初始化参数之间的区别 (Difference between Context Init Parameters and Servlet Init Parameter)
Context Init parameters | Servlet Init parameter |
---|---|
Available to all servlets and JSPs that are part of web | Available to only servlet for which the <init-param> was configured |
Context Init parameters are initialized within the not within a specific elements | Initialized within the for each specific servlet. |
ServletContext object is used to get Context Init parameters | ServletConfig object is used to get Servlet Init parameters |
Only one ServletContext object for entire web app | Each servlet has its own ServletConfig object |
上下文初始化参数 | Servlet初始化参数 |
---|---|
适用于Web的所有Servlet和JSP | 仅对配置了<init-param>的servlet可用 |
上下文初始化参数在 而不是在特定的 元素中初始化 | 在 为每个特定的servlet初始化。 |
ServletContext对象用于获取Context Init参数 | ServletConfig对象用于获取Servlet的Init参数 |
整个Web应用程序只有一个ServletContext对象 | 每个Servlet都有自己的ServletConfig对象 |
演示ServletContext用法的示例 (Example demonstrating usage of ServletContext)
web.xml web.xml<web-app ...>
<context-param>
<param-name>driverName</param-name>
<param-value>sun.jdbc.JdbcOdbcDriver</param-value>
</context-param>
<servlet>
<servlet-name>hello</servlet-name>
<servlet-class>MyServlet</servlet-class>
</servlet>
<servlet-mapping>
<servlet-name>hello</servlet-name>
<url-pattern>/hello</url-pattern>
</servlet-mapping>
</web-app>
MyServlet class : MyServlet类: import java.io.*;
import javax.servlet.*;
import javax.servlet.http.*;
public class MyServlet extends HttpServlet {
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
response.setContentType("text/html;charset=UTF-8");
PrintWriter out = response.getWriter();
ServletContext sc = getServletContext();
out.println(sc.getInitParameter("driverName"));
}
}
翻译自: https://www.studytonight.com/servlet/servlet-context.php