1.概述
1.1.分布式系统面临的问题
复杂分布式体系结构中的应用程序 有数10个依赖关系,每个依赖关系在某些时候将不可避免地失败
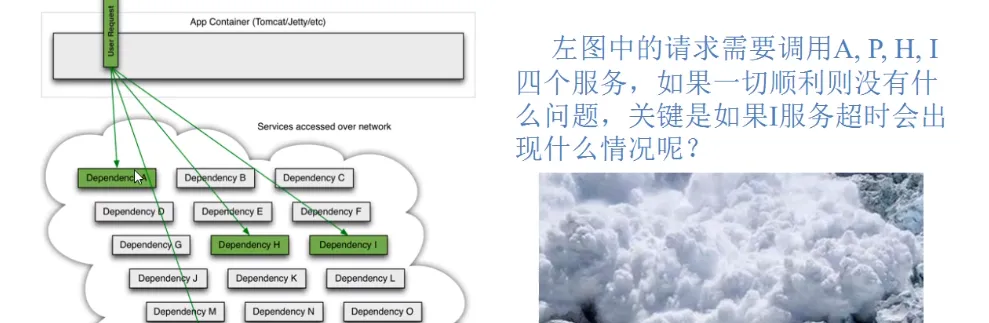
1.2.是什么
1.3.能干嘛
服务降级
服务熔断
接近实时的监控
1.4.官网资料
https://github.com/Netflix/hystrix/wiki
1.5.官宣,停更进维
https://github.com/Netflix/hystrix
被动修复bugs
不再接受合并请求
不再发布新版本
2.HyStrix重要概念
2.1.服务降级
服务器忙,请稍后再试,不让客户端等待并立刻返回一个友好提示,fallback
2.2.哪些情况会发出降级
程序运行异常
超时
服务熔断触发服务降级
线程池/信号量也会导致服务降级
2.3.服务熔断
类比保险丝达到最大服务访问后,直接拒绝访问,拉闸限电,然后调用服务降级的方法并返回友好提示
就是保险丝
服务的降级->进而熔断->恢复调用链路
2.4.服务限流
秒杀高并发等操作,严禁一窝蜂的过来拥挤,大家排队,一秒钟N个,有序进行
3.hystrix案例
3.1.构建
新建cloud-provider-hystrix-payment8001
POM
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<parent>
<artifactId>springcloud2020</artifactId>
<groupId>com.itxiongmao.springcloud</groupId>
<version>1.0-SNAPSHOT</version>
</parent>
<modelVersion>4.0.0</modelVersion>
<artifactId>cloud-provider-hystrix-payment8001</artifactId>
<dependencies>
<!-- hystrix -->
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-netflix-hystrix</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-netflix-eureka-client</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<dependency>
<groupId>com.itxiongmao.springcloud</groupId>
<artifactId>cloud-api-commons</artifactId>
<version>1.0-SNAPSHOT</version>
<scope>compile</scope>
</dependency>
</dependencies>
</project>
YML
server:
port: 8001
spring:
application:
name: cloud-provider-hystrix-payment
eureka:
client:
register-with-eureka: true
fetch-registry: true
service-url:
defaultZone: http://eureka7001.com:7001/eureka,http://eureka7002.com:7002/eureka
主启动
package com.itxiongmao;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cloud.netflix.eureka.EnableEurekaClient;
/**
* @BelongsProject: springcloud2020
* @BelongsPackage: com.itxiongmao
* @CreateTime: 2020-11-22 12:37
* @Description: TODO
*/
@SpringBootApplication
@EnableEurekaClient
public class PaymentHystrixMain8001 {
public static void main(String[] args) {
SpringApplication.run(PaymentHystrixMain8001.class, args);
}
}
业务类
service
package com.itxiongmao.service;
/**
* @BelongsProject: springcloud2020
* @BelongsPackage: com.itxiongmao.service
* @CreateTime: 2020-11-22 12:39
* @Description: TODO
*/
public interface PaymentService {
String getPaymentInfo_OK(Integer id);
String paymentInfo_TimeOut(Integer id);
}
package com.itxiongmao.service.impl;
import com.itxiongmao.service.PaymentService;
import org.springframework.stereotype.Service;
import java.util.concurrent.TimeUnit;
/**
* @BelongsProject: springcloud2020
* @BelongsPackage: com.itxiongmao.service.impl
* @CreateTime: 2020-11-22 12:39
* @Description: TODO
*/
@Service
public class PaymentServiceImpl implements PaymentService {
/*
* 正常访问
* @param id
* @return
*/
@Override
public String getPaymentInfo_OK(Integer id) {
return "线程池:" + Thread.currentThread().getName() + " paymentInfo_OK,id:" + id + "\t" + "O(∩_∩)O哈哈~";
}
/**
* 超时访问
*
* @param id
* @return
*/
@Override
public String paymentInfo_TimeOut(Integer id) {
int timeNumber = 3;
try {
// 暂停3秒钟
TimeUnit.SECONDS.sleep(timeNumber);
} catch (InterruptedException e) {
e.printStackTrace();
}
return "线程池:" + Thread.currentThread().getName() + " paymentInfo_TimeOut,id:" + id + "\t" +
"O(∩_∩)O哈哈~ 耗时(秒)" + timeNumber;
}
}
PaymentController
package com.itxiongmao.controller;
import com.itxiongmao.service.PaymentService;
import lombok.extern.slf4j.Slf4j;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RestController;
import javax.annotation.Resource;
import java.util.concurrent.TimeUnit;
@RestController
@Slf4j
public class PaymentController {
@Resource
private PaymentService paymentService;
@Value("${server.port}")
private String servicePort;
/**
* 正常访问
*
* @param id
* @return
*/
@GetMapping("/payment/hystrix/ok/{id}")
public String paymentInfo_OK(@PathVariable("id") Integer id) {
String result = paymentService.getPaymentInfo_OK(id);
log.info("*****result:" + result);
return result;
}
/**
* 超时访问
*
* @param id
* @return
*/
@GetMapping("/payment/hystrix/timeout/{id}")
public String paymentInfo_TimeOut(@PathVariable("id") Integer id) {
String result = paymentService.paymentInfo_TimeOut(id);
log.info("*****result:" + result);
return result;
}
}
3.2.正常测试
启动eureka7001
启动eureka-provider-hystrix-payment8001
访问
success的方法
http://localhost:8001/payment/hystrix/ok/31
每次调用耗费5秒钟
http://localhost:8001/payment/hystrix/timeout/31