應用啟動的時候自動啟動mock server,使整個應用都是在一個程序中維護。
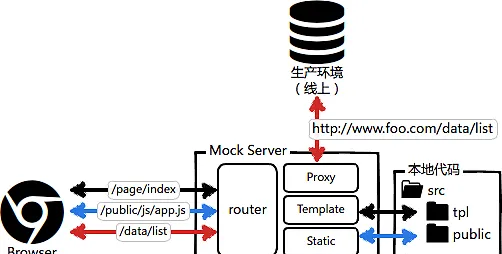
導入maven依賴。
<!-- mockserver -->
<dependency>
<groupId>org.mock-server</groupId>
<artifactId>mockserver-netty</artifactId>
<version>5.11.1</version>
</dependency>
在spring boot 應用啟動的時候自動加載并且啟動應用,端口可以根據自己的需要設定。
@Component
public class ServerControl {
private Logger logger = LoggerFactory.getLogger(getClass());
private ClientAndServer mockServer;
@PostConstruct
public void startMockServer() {
mockServer = startClientAndServer(1080);
logger.info("mock server【" + mockServer + "】 start...");
}
}
啟動應用并且先使用postman進行測試。
啟動應用并且能夠看到列印的日志相關的資訊。
在postman中使用put的方式送出期望資訊,并且測試期望傳回的内容。
發送位址
http://localhost:1080/mockserver/expectation
建立期望資訊,以post方式通路位址/hello/mock,傳回的内容可以自己随意改動。
{"httpRequest":{"method":"post","path":"/hello/mock"},"httpResponse":{"body":{"result":"W期望結果資料","status":200}},"id":"test_mock","priority":10}
發送成功後會傳回如下頁面
傳回内容如下:
[
{
"id": "test_mock",
"priority": 10,
"httpRequest": {
"method": "post",
"path": "/hello/mock"
},
"httpResponse": {
"body": {
"result": "W期望結果資料",
"status": 200
}
},
"times": {
"unlimited": true
},
"timeToLive": {
"unlimited": true
}
}
]
然後postman中重新打開一個測試的sheet。通路
http://localhost:1080/hello/mock
可以得到期望的傳回内容。
{
"result": "W期望結果資料",
"status": 200
}
以上是使用postman的方式建構,下面使用程式來模拟上面的操作。因為送出的是json内容,是以根據以上的結建構立期望建構類。
httpRequest,httpResponse,其它屬性可以根據自身需求二次擴充。
建立工具類
import org.apache.http.HttpEntity;
import org.apache.http.client.ClientProtocolException;
import org.apache.http.client.ResponseHandler;
import org.apache.http.client.methods.HttpPut;
import org.apache.http.entity.StringEntity;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.util.EntityUtils;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.IOException;
import java.nio.charset.Charset;
/**
* http操作工具類
*/
public class HttpClientUtil {
private static Logger logger = LoggerFactory.getLogger(HttpClientUtil.class);
/**
* 模拟發送httpput請求
* @param url
* @param data
* @throws IOException
*/
public static void doPut(String url, String data) throws IOException {
try (CloseableHttpClient httpclient = HttpClients.createDefault()) {
HttpPut httpPut = new HttpPut(url);
StringEntity s = new StringEntity(data.toString(),"UTF-8");
s.setContentEncoding("UTF-8");
s.setContentType("application/json");
httpPut.setEntity(s);
httpPut.addHeader("Content-type","application/json; charset=utf-8");
httpPut.setHeader("Accept", "application/json");
httpPut.setEntity(new StringEntity(data, Charset.forName("UTF-8")));
// Create a custom response handler
ResponseHandler<String> responseHandler = response -> {
int status = response.getStatusLine().getStatusCode();
if (status >= 200 && status < 300) {
HttpEntity entity = response.getEntity();
return entity != null ? EntityUtils.toString(entity,"UTF-8") : null;
} else {
throw new ClientProtocolException("Unexpected response status: " + status);
}
};
String responseBody = httpclient.execute(httpPut, responseHandler);
logger.info("-------------------請求體-----------------------");
logger.info(responseBody);
}
}
}
測試類的内容:
import com.alibaba.fastjson.JSON;
import com.alibaba.fastjson.JSONObject;
import com.primeton.api.console.mock.server.Body;
import com.primeton.api.console.mock.server.Expectations;
import com.primeton.api.console.mock.server.HttpRequest;
import com.primeton.api.console.mock.server.HttpResponse;
import org.apache.commons.httpclient.HttpStatus;
import org.apache.http.HttpEntity;
import org.apache.http.ParseException;
import org.apache.http.client.ClientProtocolException;
import org.apache.http.client.config.RequestConfig;
import org.apache.http.client.methods.CloseableHttpResponse;
import org.apache.http.client.methods.HttpPut;
import org.apache.http.entity.StringEntity;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.util.EntityUtils;
import java.io.IOException;
import java.net.URLEncoder;
public class TestHttpUtil {
public static void main(String[] args) {
Expectations expectations = new Expectations();
HttpRequest httpRequest = new HttpRequest();
HttpResponse httpResponse = new HttpResponse();
expectations.setId("test_mock");
httpRequest.setPath("/hello/mock");
httpRequest.setMethod("post");
expectations.setHttpRequest(httpRequest);
Body body = new Body();
body.setStatus(200);
body.setResult("test中文内容,中文可以随便輸入了");
httpResponse.setBody(body);
expectations.setPriority(10);
expectations.setHttpResponse(httpResponse);
String json = JSON.toJSONString(expectations);
String url = "http://localhost:1080/mockserver/expectation";
try{
HttpClientUtil.doPut(url,json);
}catch (Exception ex){
ex.printStackTrace();
}
}
}
其它的内容不變,隻是傳回的内容變了一下,我們啟動函數,然後重新用postman執行測試。
列印的日志内容如下:
14:07:52.920 [main] DEBUG org.apache.http.client.protocol.RequestAddCookies - CookieSpec selected: default
14:07:52.951 [main] DEBUG org.apache.http.client.protocol.RequestAuthCache - Auth cache not set in the context
14:07:52.956 [main] DEBUG org.apache.http.impl.conn.PoolingHttpClientConnectionManager - Connection request: [route: {}->http://localhost:1080][total available: 0; route allocated: 0 of 2; total allocated: 0 of 20]
14:07:52.998 [main] DEBUG org.apache.http.impl.conn.PoolingHttpClientConnectionManager - Connection leased: [id: 0][route: {}->http://localhost:1080][total available: 0; route allocated: 1 of 2; total allocated: 1 of 20]
14:07:53.002 [main] DEBUG org.apache.http.impl.execchain.MainClientExec - Opening connection {}->http://localhost:1080
14:07:53.019 [main] DEBUG org.apache.http.impl.conn.DefaultHttpClientConnectionOperator - Connecting to localhost/127.0.0.1:1080
14:07:53.021 [main] DEBUG org.apache.http.impl.conn.DefaultHttpClientConnectionOperator - Connection established 127.0.0.1:54023<->127.0.0.1:1080
14:07:53.022 [main] DEBUG org.apache.http.impl.execchain.MainClientExec - Executing request PUT /mockserver/expectation HTTP/1.1
14:07:53.022 [main] DEBUG org.apache.http.impl.execchain.MainClientExec - Target auth state: UNCHALLENGED
14:07:53.022 [main] DEBUG org.apache.http.impl.execchain.MainClientExec - Proxy auth state: UNCHALLENGED
14:07:53.027 [main] DEBUG org.apache.http.headers - http-outgoing-0 >> PUT /mockserver/expectation HTTP/1.1
14:07:53.027 [main] DEBUG org.apache.http.headers - http-outgoing-0 >> Content-type: application/json; charset=utf-8
14:07:53.027 [main] DEBUG org.apache.http.headers - http-outgoing-0 >> Accept: application/json
14:07:53.027 [main] DEBUG org.apache.http.headers - http-outgoing-0 >> Content-Length: 182
14:07:53.027 [main] DEBUG org.apache.http.headers - http-outgoing-0 >> Host: localhost:1080
14:07:53.027 [main] DEBUG org.apache.http.headers - http-outgoing-0 >> Connection: Keep-Alive
14:07:53.027 [main] DEBUG org.apache.http.headers - http-outgoing-0 >> User-Agent: Apache-HttpClient/4.5.13 (Java/1.8.0_152)
14:07:53.027 [main] DEBUG org.apache.http.headers - http-outgoing-0 >> Accept-Encoding: gzip,deflate
14:07:53.028 [main] DEBUG org.apache.http.wire - http-outgoing-0 >> "PUT /mockserver/expectation HTTP/1.1[\r][\n]"
14:07:53.028 [main] DEBUG org.apache.http.wire - http-outgoing-0 >> "Content-type: application/json; charset=utf-8[\r][\n]"
14:07:53.028 [main] DEBUG org.apache.http.wire - http-outgoing-0 >> "Accept: application/json[\r][\n]"
14:07:53.028 [main] DEBUG org.apache.http.wire - http-outgoing-0 >> "Content-Length: 182[\r][\n]"
14:07:53.028 [main] DEBUG org.apache.http.wire - http-outgoing-0 >> "Host: localhost:1080[\r][\n]"
14:07:53.028 [main] DEBUG org.apache.http.wire - http-outgoing-0 >> "Connection: Keep-Alive[\r][\n]"
14:07:53.028 [main] DEBUG org.apache.http.wire - http-outgoing-0 >> "User-Agent: Apache-HttpClient/4.5.13 (Java/1.8.0_152)[\r][\n]"
14:07:53.028 [main] DEBUG org.apache.http.wire - http-outgoing-0 >> "Accept-Encoding: gzip,deflate[\r][\n]"
14:07:53.028 [main] DEBUG org.apache.http.wire - http-outgoing-0 >> "[\r][\n]"
14:07:53.028 [main] DEBUG org.apache.http.wire - http-outgoing-0 >> "{"httpRequest":{"method":"post","path":"/hello/mock"},"httpResponse":{"body":{"result":"test[0xe4][0xb8][0xad][0xe6][0x96][0x87][0xe5][0x86][0x85][0xe5][0xae][0xb9][0xef][0xbc][0x8c][0xe4][0xb8][0xad][0xe6][0x96][0x87][0xe5][0x8f][0xaf][0xe4][0xbb][0xa5][0xe9][0x9a][0x8f][0xe4][0xbe][0xbf][0xe8][0xbe][0x93][0xe5][0x85][0xa5][0xe4][0xba][0x86]","status":200}},"id":"test_mock","priority":10}"
14:07:53.275 [main] DEBUG org.apache.http.wire - http-outgoing-0 << "HTTP/1.1 201 Created[\r][\n]"
14:07:53.275 [main] DEBUG org.apache.http.wire - http-outgoing-0 << "version: 5.11.1[\r][\n]"
14:07:53.275 [main] DEBUG org.apache.http.wire - http-outgoing-0 << "connection: keep-alive[\r][\n]"
14:07:53.275 [main] DEBUG org.apache.http.wire - http-outgoing-0 << "content-type: application/json; charset=utf-8[\r][\n]"
14:07:53.275 [main] DEBUG org.apache.http.wire - http-outgoing-0 << "content-length: 365[\r][\n]"
14:07:53.275 [main] DEBUG org.apache.http.wire - http-outgoing-0 << "[\r][\n]"
14:07:53.275 [main] DEBUG org.apache.http.wire - http-outgoing-0 << "[ {[\r][\n]"
14:07:53.275 [main] DEBUG org.apache.http.wire - http-outgoing-0 << " "id" : "test_mock",[\r][\n]"
14:07:53.275 [main] DEBUG org.apache.http.wire - http-outgoing-0 << " "priority" : 10,[\r][\n]"
14:07:53.276 [main] DEBUG org.apache.http.wire - http-outgoing-0 << " "httpRequest" : {[\r][\n]"
14:07:53.276 [main] DEBUG org.apache.http.wire - http-outgoing-0 << " "method" : "post",[\r][\n]"
14:07:53.276 [main] DEBUG org.apache.http.wire - http-outgoing-0 << " "path" : "/hello/mock"[\r][\n]"
14:07:53.276 [main] DEBUG org.apache.http.wire - http-outgoing-0 << " },[\r][\n]"
14:07:53.276 [main] DEBUG org.apache.http.wire - http-outgoing-0 << " "httpResponse" : {[\r][\n]"
14:07:53.276 [main] DEBUG org.apache.http.wire - http-outgoing-0 << " "body" : {[\r][\n]"
14:07:53.276 [main] DEBUG org.apache.http.wire - http-outgoing-0 << " "result" : "test[0xe4][0xb8][0xad][0xe6][0x96][0x87][0xe5][0x86][0x85][0xe5][0xae][0xb9][0xef][0xbc][0x8c][0xe4][0xb8][0xad][0xe6][0x96][0x87][0xe5][0x8f][0xaf][0xe4][0xbb][0xa5][0xe9][0x9a][0x8f][0xe4][0xbe][0xbf][0xe8][0xbe][0x93][0xe5][0x85][0xa5][0xe4][0xba][0x86]",[\r][\n]"
14:07:53.276 [main] DEBUG org.apache.http.wire - http-outgoing-0 << " "status" : 200[\r][\n]"
14:07:53.276 [main] DEBUG org.apache.http.wire - http-outgoing-0 << " }[\r][\n]"
14:07:53.276 [main] DEBUG org.apache.http.wire - http-outgoing-0 << " },[\r][\n]"
14:07:53.276 [main] DEBUG org.apache.http.wire - http-outgoing-0 << " "times" : {[\r][\n]"
14:07:53.276 [main] DEBUG org.apache.http.wire - http-outgoing-0 << " "unlimited" : true[\r][\n]"
14:07:53.276 [main] DEBUG org.apache.http.wire - http-outgoing-0 << " },[\r][\n]"
14:07:53.276 [main] DEBUG org.apache.http.wire - http-outgoing-0 << " "timeToLive" : {[\r][\n]"
14:07:53.276 [main] DEBUG org.apache.http.wire - http-outgoing-0 << " "unlimited" : true[\r][\n]"
14:07:53.276 [main] DEBUG org.apache.http.wire - http-outgoing-0 << " }[\r][\n]"
14:07:53.276 [main] DEBUG org.apache.http.wire - http-outgoing-0 << "} ]"
14:07:53.280 [main] DEBUG org.apache.http.headers - http-outgoing-0 << HTTP/1.1 201 Created
14:07:53.280 [main] DEBUG org.apache.http.headers - http-outgoing-0 << version: 5.11.1
14:07:53.280 [main] DEBUG org.apache.http.headers - http-outgoing-0 << connection: keep-alive
14:07:53.280 [main] DEBUG org.apache.http.headers - http-outgoing-0 << content-type: application/json; charset=utf-8
14:07:53.280 [main] DEBUG org.apache.http.headers - http-outgoing-0 << content-length: 365
14:07:53.286 [main] DEBUG org.apache.http.impl.execchain.MainClientExec - Connection can be kept alive indefinitely
14:07:53.294 [main] DEBUG org.apache.http.impl.conn.PoolingHttpClientConnectionManager - Connection [id: 0][route: {}->http://localhost:1080] can be kept alive indefinitely
14:07:53.294 [main] DEBUG org.apache.http.impl.conn.DefaultManagedHttpClientConnection - http-outgoing-0: set socket timeout to 0
14:07:53.294 [main] DEBUG org.apache.http.impl.conn.PoolingHttpClientConnectionManager - Connection released: [id: 0][route: {}->http://localhost:1080][total available: 1; route allocated: 1 of 2; total allocated: 1 of 20]
14:07:53.294 [main] INFO com.primeton.api.console.mock.util.HttpClientUtil - -------------------請求體-----------------------
14:07:53.294 [main] INFO com.primeton.api.console.mock.util.HttpClientUtil - [ {
"id" : "test_mock",
"priority" : 10,
"httpRequest" : {
"method" : "post",
"path" : "/hello/mock"
},
"httpResponse" : {
"body" : {
"result" : "test中文内容,中文可以随便輸入了",
"status" : 200
}
},
"times" : {
"unlimited" : true
},
"timeToLive" : {
"unlimited" : true
}
} ]
14:07:53.294 [main] DEBUG org.apache.http.impl.conn.PoolingHttpClientConnectionManager - Connection manager is shutting down
14:07:53.295 [main] DEBUG org.apache.http.impl.conn.DefaultManagedHttpClientConnection - http-outgoing-0: Close connection
14:07:53.295 [main] DEBUG org.apache.http.impl.conn.PoolingHttpClientConnectionManager - Connection manager shut down
Process finished with exit code 0
重新請求
http://localhost:1080/hello/mock
我們可以看到内容變成我們期望的内容了。