生成二維碼工具類
package com.itheima.hchat.util;
import java.awt.image.BufferedImage;
import java.io.File;
import java.nio.file.Path;
import java.util.HashMap;
import javax.imageio.ImageIO;
import org.springframework.stereotype.Component;
import com.google.zxing.BarcodeFormat;
import com.google.zxing.BinaryBitmap;
import com.google.zxing.EncodeHintType;
import com.google.zxing.MultiFormatReader;
import com.google.zxing.MultiFormatWriter;
import com.google.zxing.Result;
import com.google.zxing.client.j2se.BufferedImageLuminanceSource;
import com.google.zxing.client.j2se.MatrixToImageWriter;
import com.google.zxing.common.BitMatrix;
import com.google.zxing.common.HybridBinarizer;
import com.google.zxing.qrcode.decoder.ErrorCorrectionLevel;
@Component
public class QRCodeUtils {
/**
* 儲存二維碼到指定路徑
* filePath 指定路徑
* content 二維碼内容
*/
public void createQRCode(String filePath, String content) {
int width=300; //圖檔的寬度
int height=300; //圖檔的高度
String format="png"; //圖檔的格式\
/**
* 定義二維碼的參數
*/
HashMap hints=new HashMap();
hints.put(EncodeHintType.CHARACTER_SET,"utf-8"); //指定字元編碼為“utf-8”
hints.put(EncodeHintType.ERROR_CORRECTION,ErrorCorrectionLevel.M); //指定二維碼的糾錯等級為中級
hints.put(EncodeHintType.MARGIN, 2); //設定圖檔的邊距
/**
* 生成二維碼
*/
try {
BitMatrix bitMatrix=new MultiFormatWriter().encode(content, BarcodeFormat.QR_CODE, width, height,hints);
Path file=new File(filePath).toPath();
MatrixToImageWriter.writeToPath(bitMatrix, format, file);
} catch (Exception e) {
e.printStackTrace();
}
}
public String getContentFromQRCode(String filePath) {
MultiFormatReader formatReader=new MultiFormatReader();
File file=new File(filePath);
BufferedImage image;
try {
image = ImageIO.read(file);
BinaryBitmap binaryBitmap=new BinaryBitmap(new HybridBinarizer
(new BufferedImageLuminanceSource(image)));
HashMap hints=new HashMap();
hints.put(EncodeHintType.CHARACTER_SET,"utf-8"); //指定字元編碼為“utf-8”
Result result=formatReader.decode(binaryBitmap,hints);
return result.toString();
} catch (Exception e) {
e.printStackTrace();
return null;
}
}
}
使用工具類生成二維碼:
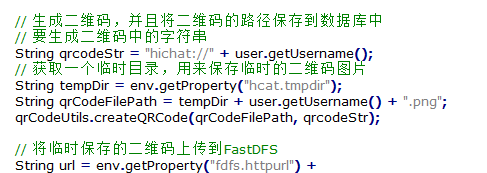
// 生成二維碼,并且将二維碼的路徑儲存到資料庫中
// 要生成二維碼中的字元串
String qrcodeStr = "hichat://" + user.getUsername();
// 擷取一個臨時目錄,用來儲存臨時的二維碼圖檔
String tempDir = env.getProperty("hcat.tmpdir");
String qrCodeFilePath = tempDir + user.getUsername() + ".png";
qrCodeUtils.createQRCode(qrCodeFilePath, qrcodeStr);
// 将臨時儲存的二維碼上傳到FastDFS
String url = env.getProperty("fdfs.httpurl") +
fastDFSClient.uploadFile(new File(qrCodeFilePath));
完整:
package com.itheima.hchat.service.impl;
import com.itheima.hchat.mapper.TbFriendMapper;
import com.itheima.hchat.mapper.TbFriendReqMapper;
import com.itheima.hchat.mapper.TbUserMapper;
import com.itheima.hchat.pojo.*;
import com.itheima.hchat.pojo.vo.User;
import com.itheima.hchat.service.UserService;
import com.itheima.hchat.util.FastDFSClient;
import com.itheima.hchat.util.IdWorker;
import com.itheima.hchat.util.QRCodeUtils;
import org.apache.commons.lang3.StringUtils;
import org.omg.SendingContext.RunTime;
import org.springframework.beans.BeanUtils;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.core.env.Environment;
import org.springframework.stereotype.Service;
import org.springframework.transaction.annotation.Transactional;
import org.springframework.util.DigestUtils;
import org.springframework.web.multipart.MultipartFile;
import javax.management.RuntimeErrorException;
import java.io.File;
import java.io.IOException;
import java.util.Date;
import java.util.List;
@Service
@Transactional
public class UserServiceImpl implements UserService {
@Autowired
private TbUserMapper userMapper;
@Autowired
private IdWorker idWorker;
@Autowired
private FastDFSClient fastDFSClient;
@Autowired
private Environment env;
@Autowired
private QRCodeUtils qrCodeUtils;
@Autowired
private TbFriendMapper friendMapper;
@Autowired
private TbFriendReqMapper friendReqMapper;
@Override
public List<TbUser> findAll() {
return userMapper.selectByExample(null);
}
@Override
public User login(String username, String password) {
if(StringUtils.isNotBlank(username) && StringUtils.isNotBlank(password)) {
TbUserExample example = new TbUserExample();
TbUserExample.Criteria criteria = example.createCriteria();
criteria.andUsernameEqualTo(username);
List<TbUser> userList = userMapper.selectByExample(example);
if(userList != null && userList.size() == 1) {
// 對密碼進行校驗
String encodingPassword = DigestUtils.md5DigestAsHex(password.getBytes());
if(encodingPassword.equals(userList.get(0).getPassword())) {
User user = new User();
BeanUtils.copyProperties(userList.get(0), user);
return user;
}
}
}
return null;
}
@Override
public void register(TbUser user) {
try {
// 1. 判斷這個使用者名是否存在
TbUserExample example = new TbUserExample();
TbUserExample.Criteria criteria = example.createCriteria();
criteria.andUsernameEqualTo(user.getUsername());
List<TbUser> userList = userMapper.selectByExample(example);
if(userList != null && userList.size() > 0) {
throw new RuntimeException("使用者已存在");
}
// 2. 将使用者資訊儲存到資料庫中
// 使用雪花算法來生成唯一ID
user.setId(idWorker.nextId());
// 對密碼進行MD5加密
user.setPassword(DigestUtils.md5DigestAsHex(user.getPassword().getBytes()));
user.setPicSmall("");
user.setPicNormal("");
user.setNickname(user.getUsername());
// 生成二維碼,并且将二維碼的路徑儲存到資料庫中
// 要生成二維碼中的字元串
String qrcodeStr = "hichat://" + user.getUsername();
// 擷取一個臨時目錄,用來儲存臨時的二維碼圖檔
String tempDir = env.getProperty("hcat.tmpdir");
String qrCodeFilePath = tempDir + user.getUsername() + ".png";
qrCodeUtils.createQRCode(qrCodeFilePath, qrcodeStr);
// 将臨時儲存的二維碼上傳到FastDFS
String url = env.getProperty("fdfs.httpurl") +
fastDFSClient.uploadFile(new File(qrCodeFilePath));
user.setQrcode(url);
user.setCreatetime(new Date());
userMapper.insert(user);
} catch (IOException e) {
e.printStackTrace();
throw new RuntimeException("注冊失敗");
}
}
@Override
public User upload(MultipartFile file, String userid) {
try {
// 傳回在FastDFS中的URL路徑,這個路徑是不帶http://192.168.25.133/..
String url = fastDFSClient.uploadFace(file);
// 在FastDFS上傳的時候,會自動生成一個縮略圖
// 檔案名_150x150.字尾
String[] fileNameList = url.split("\\.");
String fileName = fileNameList[0];
String ext = fileNameList[1];
String picSmallUrl = fileName + "_150x150." + ext;
String prefix = env.getProperty("fdfs.httpurl");
TbUser tbUser = userMapper.selectByPrimaryKey(userid);
// 設定頭像大圖檔
tbUser.setPicNormal(prefix + url);
// 設定頭像小圖檔
tbUser.setPicSmall(prefix + picSmallUrl);
// 将新的頭像URL更新到資料庫中
userMapper.updateByPrimaryKey(tbUser);
// 将使用者資訊傳回到Controller
User user = new User();
BeanUtils.copyProperties(tbUser, user);
return user;
} catch (Exception e) {
e.printStackTrace();
return null;
}
}
@Override
public void updateNickname(String id, String nickname) {
if(StringUtils.isNotBlank(nickname)) {
TbUser tbUser = userMapper.selectByPrimaryKey(id);
tbUser.setNickname(nickname);
userMapper.updateByPrimaryKey(tbUser);
}
else {
throw new RuntimeException("昵稱不能為空");
}
}
@Override
public User findById(String userid) {
TbUser tbUser = userMapper.selectByPrimaryKey(userid);
User user = new User();
BeanUtils.copyProperties(tbUser, user);
return user;
}
@Override
public User findByUsername(String userid, String friendUsername) {
TbUserExample example = new TbUserExample();
TbUserExample.Criteria criteria = example.createCriteria();
criteria.andUsernameEqualTo(friendUsername);
List<TbUser> userList = userMapper.selectByExample(example);
TbUser friend = userList.get(0);
User friendUser = new User();
BeanUtils.copyProperties(friend, friendUser);
return friendUser;
}
}