https://github.com/grpc-ecosystem/grpc-gateway
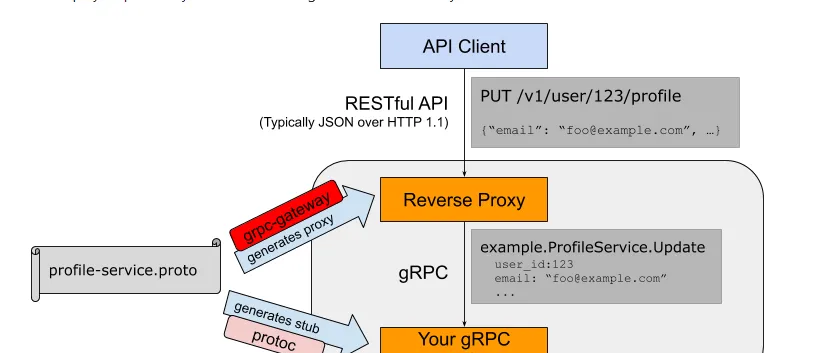
在grpc之上加一層代理并轉發,轉變成protobuf格式來通路grpc服務
安裝
go get -u github.com/grpc-ecosystem/grpc-gateway/protoc-gen-grpc-gateway
go get -u github.com/grpc-ecosystem/grpc-gateway/protoc-gen-swagger
go get -u github.com/golang/protobuf/protoc-gen-go
Prod.proto
syntax="proto3";
package services;
import "google/api/annotations.proto";
message ProdRequest {
int32 prod_id =1; //傳入的商品ID
}
message ProdResponse{
int32 prod_stock=1;//商品庫存
}
service ProdService {
rpc GetProdStock (ProdRequest) returns (ProdResponse){
option (google.api.http) = {
get: "/v1/prod/{prod_id}"
};
}
}
生成兩個檔案
首先cd 進入pbfiles
這會生成Prod.pb.go
protoc --go_out=plugins=grpc:../services Prod.proto
這會生成Prod.pb.gw.go
protoc --grpc-gateway_out=logtostderr=true:../services Prod.proto
httpserver.go
package main
import (
"context"
"github.com/grpc-ecosystem/grpc-gateway/runtime"
"google.golang.org/grpc"
"grpcpro/services"
"log"
"net/http"
)
func main() {
gwmux:=runtime.NewServeMux()
opt := []grpc.DialOption{grpc.WithInsecure()}
err:=services.RegisterProdServiceHandlerFromEndpoint(context.Background(),
gwmux,"localhost:8081",opt)
if err != nil {
log.Fatal(err)
}
httpServer:=&http.Server{
Addr:":8080",
Handler:gwmux,
}
httpServer.ListenAndServe()
}
server.go
package main
import (
"google.golang.org/grpc"
"grpcpro/services"
"net"
)
func main() {
rpcServer:=grpc.NewServer()
services.RegisterProdServiceServer(rpcServer,new(services.ProdService))
lis,_:=net.Listen("tcp",":8081")
rpcServer.Serve(lis)
}