JavaScript Basics Part 1 – Variables
Throughout the previous chapter we learned three of the layouts, in the layout learned the streaming layout and flex layout and the final rem layout, which are related to the layout, because of the popularity of the mobile terminal, so it is inevitable to need to be compatible with various terminals in the front end. Therefore, there are still many ways, each showing its own magic. You can write according to the business scenario displayed or the layout method that your team is good at.
After learning the layout, today we entered a very important knowledge point in the front end, that is: JavaScript, commonly known as: js. It is also a very important and very interesting part of the front end, and writing business logic in the future work is inseparable from js! So the foundation must be solid.
Before learning js, we can roughly understand some of the basics of computers. Learn about the differences between programming languages and markup languages, memory, and more.
Programming language: there is a strong logic and behavior ability, in the programming language, you will see a lot of judgment logic, such as: if else, for, while and other logical behavior instructions, these are active.
Markup Language: Without issuing instructions to the computer, it is often used for formatting and linking, and the existence of markup language is used to read it passively.
Computers are composed of hardware and software, and the hardware is generally memory, mouse, keyboard, CPU, hard disk, etc.
Memory: The advent of memory has greatly improved the speed of data reading. A long time ago, because the CPU computing power was too fast and the hard disk read data too slowly, it wasted time, so there was memory to save the running data.
You don't need to understand these things for the time being, just remember them as an understanding. The more you study in the future, the more you will find that the basic skills are lacking.
The browser is divided into two parts, the rendering engine and the js engine
Rendering engine: used to parse HTML and CSS, commonly known as the kernel, such as the Blink of chrome browser, the old version of webkit.
js engine: Also known as the JS interpreter, it is used to read JavaScript code in web pages and run it after processing it, such as Chrome Browser V8
The first thing to note is that the browser itself does not execute JS code, but through the built-in JavaScript engine (interpreter) to execute JS code, the JS engine executes each sentence of source code line by line (converted to machine language), and then executed by the computer, so the JavaScript language is classified as a scripting language, and will be interpreted line by line
ECMAScript: It specifies the programming syntax and basic core knowledge of JS, which is equivalent to a standard for developing js, and is also a set of JS syntax industry standards that all browser vendors adhere to. The ES6 and ES5 you're familiar with are all from ECMAScript.
DOM: Page Document Object Model. It is a platform- and language-independent api. Just remember here, I will learn a lot of DOM APIs in the future.
BOM: Browser object model. The window object of the browser window is the top-level object of the BOM, and the other objects are all child objects of the object.
Note: When you first start learning, you will feel that these three components are more abstract. It's more difficult to understand, it doesn't matter how slowly, after the basic js part is learned, we will gradually learn the API methods of DOM and BOM
Like css, js will be introduced in three ways: inline, inline, and external. Where we generally write code using inline. Generally using third-party libraries will also be useful for outreach, but if it is outreach, < script></script> the middle is not allowed to write code.
Single-line comment: //
Multi-line comment: /**/
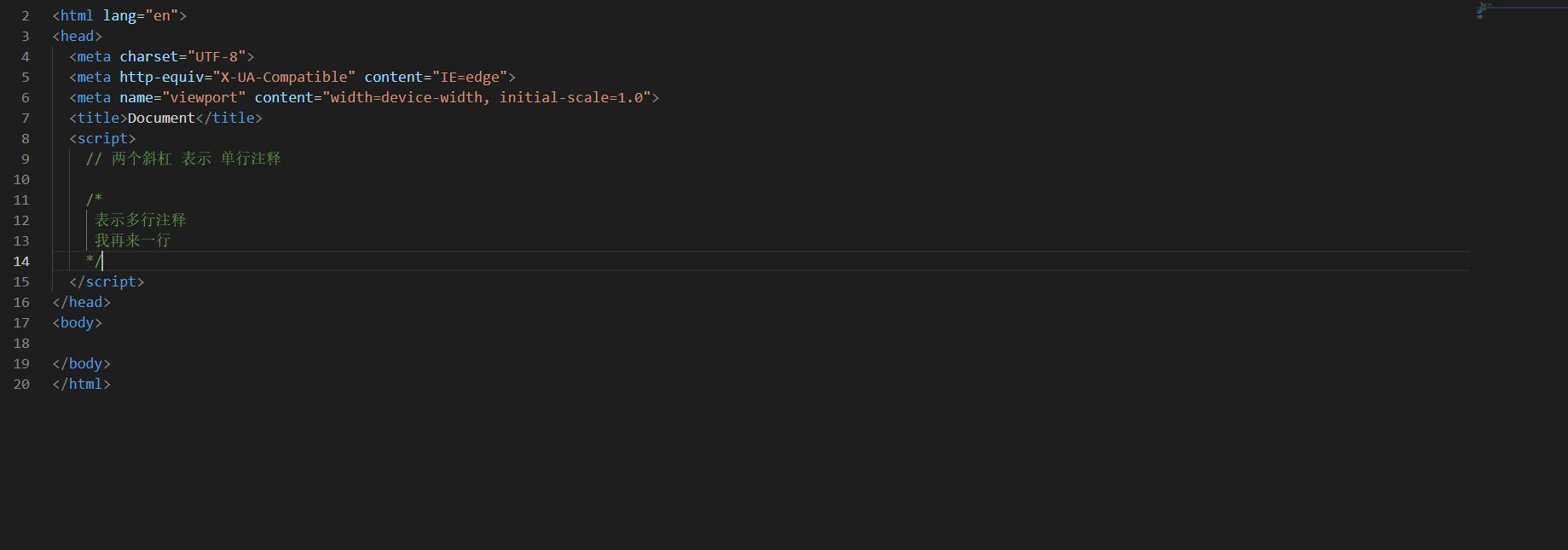
alert(msg) – The browser pops up an alert box
console .log (msg) – The browser console prints out the output information
prompt(msg) – The browser pops up an input box that the user can enter
Variables Overview: Variables are about applying for a space from memory to hold data
Use of variables: By declaring variables with var, the computer automatically allocates space to the program
Assignment: var myName = 'stk'
Note: If a value is reassigned, its original value will be overwritten, and the variable value will be subject to the last assignment
Declare multiple variables:
If only no value is declared, it will appear: undefined
- Introduction to data types: Different data types in the computer, the storage space is different
- Simple data types:
Number: Numeric type (integer, floating-point)
MAX_VALUE – represents the maximum value of the data num. MAX_VALUE
MIN_VALUE – represents the minimum value num of the data. MIN_VALUE
isNaN() - Determines non-numeric. If false is returned means a number, if true means not a number Usage: console.log (isNaN(12)) - returns false
Boolean: Boolean type (true, false)
String: String type ('Zhang San')
Note: Any type and string added up becomes a string type
String escape characters
\n : Line break
\ : Slash
' : Single quotation marks
" : Double quotation marks
\t : tab indentation
\b : Spaces
Gets the string length: name.length
undefined: No value is given, it is undefined, for example: var a; output a prints undefined
Null: Declared null NaN appears if the number and undefined are added, (not a number)
Detect what type the variable is: typeof
Converts a variable of one data type to another data type
- Convert string types
toString() - convert string: var num = 1; alert(num.toString())
String() —— var num = 1; alert(String(num))
Plus stitching string (emphasis) – var num = 1; alert(num + 'I'm a string')
- Convert number types (emphasis)
ParseInt() function - parseInt('79') converts integers
ParseFloat() function - parseFloat('78.21') converts decimals
Number() function - parseFloat('12')
- Other types convert boolean types
Boolean() function - Boolean('True')
Note: Values that represent null, negative, are converted to false, such as "", 0, NaN, null, undefined, and the rest are true
Computers cannot directly understand any language other than machine language, so the programmer's abbreviated programming language must be compiled into a machine language before it can be executed. The tool for compiling machine languages in programming languages is called: compilers.
But there are two ways for compilers to compile, one is to compile and the other is to interpret, and the difference between the two methods is that the compilation time is different
Compiled language: A compiler is a compiler that compiles code before it executes and then generates an intermediate code file
Interpreted language: The interpreter is a timely interpretation at runtime and executes immediately (also called an interpreter when the compiler runs in an interpretive manner)
Languages such as Java are called compiled languages, while the js we learn are called interpreted languages.
This article is still quite a lot of content, but also a js entry foundation, the basics are always boring, so it needs to be adhered to and practiced. "Read the book a hundred times, and its meaning is self-evident." This article is the end of the foundation, if there is any problem, please feel free to leave a message ~