PoJO is defined as a simple object without rules, and in everyday code hierarchy pojo is divided into VO, BO, PO, and DTOs
VO (view object/value object) represents a layer object
1. The data displayed by the front-end needs to be converted to VO when the interface data is returned to the front-end
2. Personal understanding of the use scenario, in the interface layer service, the DTO is converted into VO and returned to the front desk
B0 (bussines object) Business layer object
1. Business objects that are mainly used within the service
2. It can contain multiple objects and can be used for aggregation operations of objects
3. Personal understanding of the use case, in the service layer service, from the DTO to bo and then after the business processing, it is converted to the DTO and returned to the interface layer
PO (persistent object) Persistent object
1. The location is database data, which is used to store the data extracted by the database
2. Only data is stored, not data operations
3. Personal understanding of the use scenario, in the database layer, the obtained database data is stored in the PO, and then converted to the DTO and returned to the service layer
DTO (Data Transfer Object) Data transfer object
1. In the call between services, the data object is transmitted
2. Personal understanding, DTO is the interaction between services that can exist in each layer of services (interface, service, database, etc.) using DTOs to decouple
DO(domain object) Domain entity object
There are now two main versions of DO:
(1) As defined in Alibaba's development manual, DO(Data Object) is equivalent to the PO above
(2) In the domain-driven design of DDD (Domain-Driven Design), DO (Domain Object) is equivalent to the BO above
Reference Documentation:
https://juejin.cn/post/6952848675924082718
https://juejin.cn/post/6844904046097072141
https://zhuanlan.zhihu.com/p/264675395
Plugin name: Simple Object Copy
1. Define the method of entry and exit parameters
2. In the cursor positioning method, use the shortcut keys ALT+INSERT(WIN), command+N(mac), or right-click the mouse to select Generate, pop up the generate option box, select genCopyMethod, and the code will be generated
Requires handwritten code
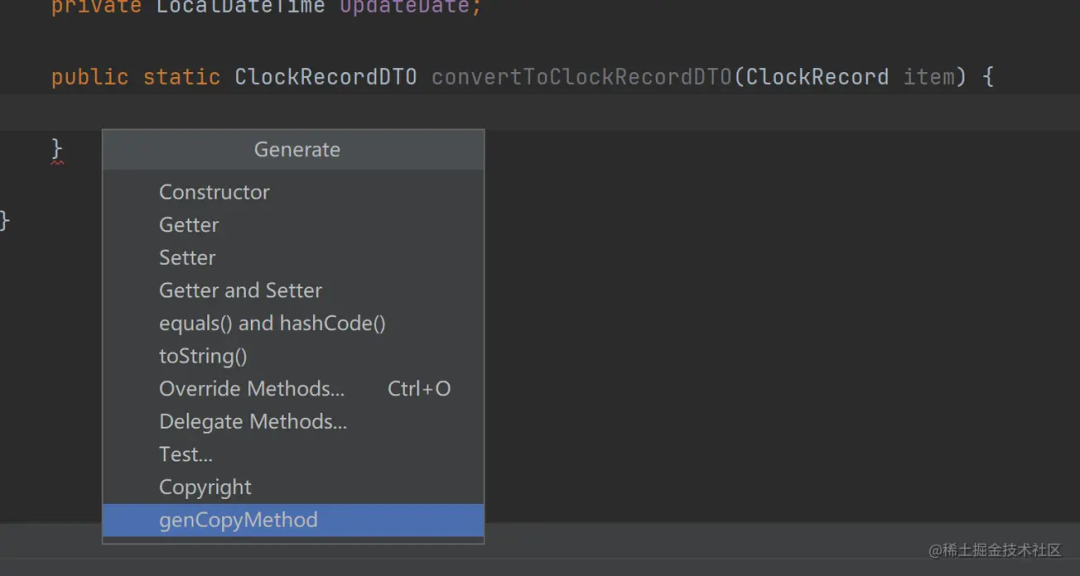
One-click generated display
Complex object transformation display (one-click generated display)
Example of complex object conversion source code:
@Data
public class UserVO {
private String name;
private Date entryDate;
private String userId;
private List<RoleVO> roleList;
private RoomVO room;
public static UserVO convertToUserVO(UserDTO item) {
if (item == ) {
return ;
}
UserVO result = new UserVO;
result.setName(item.getName);
result.setEntryDate(item.getEntryDate);
result.setUserId(item.getUserId);
List<RoleDTO> roleList = item.getRoleList;
if (roleList == ) {
result.setRoleList;
} else {
result.setRoleList(roleList.stream.map(UserVO::convertToRoleVO).collect(Collectors.toList);
}
result.setRoom(convertToRoomVO(item.getRoom));
return result;
}
public static RoomVO convertToRoomVO(RoomDTO item) {
if (item == ) {
return ;
}
RoomVO result = new RoomVO;
result.setRoomId(item.getRoomId);
result.setBuildingId(item.getBuildingId);
result.setRoomName;
result.setBuildingName;
return result;
}
public static RoleVO convertToRoleVO(RoleDTO item) {
if (item == ) {
return ;
}
RoleVO result = new RoleVO;
result.setRoleId(item.getRoleId);
result.setRoleName(item.getRoleName);
result.setCreateTime(item.getCreateTime);
return result;
}
}
@Data
public class UserDTO {
private String name;
private Date entryDate;
private String userId;
private List<RoleDTO> roleList;
private RoomDTO room;
}
@Data
public class RoleVO {
private String roleId;
private String roleName;
private LocalDateTime createTime;
}
@Data
public class RoleDTO {
private String roleId;
private String roleName;
private LocalDateTime createTime;
}
@Data
public class RoomVO {
private String roomId;
private String buildingId;
private String roomName;
private String buildingName;
}
@Data
public class RoomDTO {
private String roomId;
private String buildingId;
}
1. There are many similar types of tools on the market without intrusion, and the more commonly used ones are
1、Spring BeanUtils (copyProperties)
2、Cglib BeanCopier (copyProperties)
3、Apache BeanUtils (copyProperties)
4、Apache PropertyUtils (copyProperties)
5、Dozer
6、mapstruct
7. JSON serialization and then deserialization
These tools, not only to introduce the corresponding dependent jar package, but also to the code intrusion, to call the corresponding API method to convert, once encountered type inconsistency, the field name slightly changed, you need to write another java code to complete the field, the overall code is very ugly.
Example:
1. mapstruct
The same code, not only to introduce dependencies, write the following conversion mapper, but also, in the corresponding place to call the corresponding API (code intrusion verification), but Simple Object Copy only needs to be generated with one click.
RoomName, buildingName, and mapstruct alternatives that do not exist in RoomDTO are easy to ignore. Properties that do not exist in the source entity, no prompt, be careful that the front end always asks why.
After the Simple Object Copy plugin code is generated, the non-existent fields also generate empty methods, which are directly compiled and supplemented by prompts, which are not easy to ignore
Code that requires handwriting:
@Mapper(componentModel = "spring",uses = {RoleVOMapper.class,RoomVOMapper.class})
publicinterfaceUserMapper{
UserConverter INSTANCE = Mappers.getMapper(UserConverter.class);
UserVO toUserVO(UserDTO userDTO);
}
@Mapper(componentModel = "spring")
publicinterfaceRoleMapper{
RoleVO toRoleVO(RoleDTO roleDTO);
}
@Mapper(componentModel = "spring")
publicinterfaceRoomMapper{
RoomVO toRoomVO(RoomDTO roomDTO);
}
publicclassMain{
publicstaticvoidmain(String[] args) {
UserDTO user = ;
UserVO userVO = UserMapper.INSTANCE.toUserVO(user);
userVO.getRoomVO.setRoomName("大厅1");
userVO.getRoomVO.setBuildingName("尚德楼");
}
}
1. BeanUtils
Slightly worse performance.
Do not support complex objects or write a lot of code, code fields are not clear and difficult to understand, others are difficult to take over. RoomName, buildingName and BeanUtils, which do not exist in roomDTO, are easy to ignore. Properties that do not exist in the source entity, no prompt, be careful that the front end always asks why.
@Data
publicclassUserVO{
private String name;
private Date entryDate;
private String userId;
private List<RoleVO> roleList;
private RoomVO room;
public static UserVO convertToUserVO(UserDTO item) {
if (item == ) {
return ;
}
UserVO result = new UserVO;
BeanUtils.copyProperties(item,result);
List<RoleDTO> roleList = item.getRoleList;
if (roleList == ) {
result.setRoleList;
} else {
result.setRoleList(roleList.stream.map(UserVO::convertToRoleVO).collect(Collectors.toList);
}
result.setRoom(convertToRoomVO(item.getRoom));
return result;
}
public static RoomVO convertToRoomVO(RoomDTO item) {
if (item == ) {
return ;
}
RoomVO result = new RoomVO;
BeanUtils.copyProperties(item,result);
result.setRoomName;
result.setBuildingName;
return result;
}
public static RoleVO convertToRoleVO(RoleDTO item) {
if (item == ) {
return ;
}
RoleVO result = new RoleVO;
BeanUtils.copyProperties(item,result);
return result;
}
}
2. Performance advantages
Compared with the above tool class, either reflection is used, or the operation is performed by proxy and serialization. Compared to the pure set method to transform, the gap is not an order of magnitude. I will not repeat it this time.
3. Flexibility, compatibility
Compared with the above tool classes, plug-ins have great advantages, and will not be repeated, let's compare below, I used the idea plug-in generateO2O before
Here I recommend another plugin I use: generateAllSetter, which is better to eat with,
4. How do I download it?
Open idea plugins and cut to market place search: Simple Object Copy
Source: juejin.cn/post/7053264631262871583