1.1 String概述
- 字元串是由多個字元組成的一串資料
- 字元串可以看成是字元數組
1.2 構造方法
- public String(String original)
- public String(char[] value)
- public String(char[] value,int offset,int count)
- 直接指派也可以是一個對象
- 注意:字元串是一種比較特殊的引用資料類型,直接輸出字元串對象輸出的是該對象中的資料
package com.study.demo.stringdemo;
/**
* @Auther: lds
* @Date: 2018/11/23 14:41
* @Description:
*/
public class StringDemo01 {
public static void main(String[] args) {
//方式 1
//String(String original):把字元串資料封裝成字元串對象
String s1 = new String("hello");
System.out.println("s1:" + s1);
System.out.println("---------");
//方式 2
//String(char[] value):把字元數組的資料封裝成字元串對象
char[] chs = {'h', 'e', 'l', 'l', 'o'};
String s2 = new String(chs);
System.out.println("s2:" + s2);
System.out.println("---------");
//方式 3
//String(char[] value, int index, int count):把字元數組中的一部分資料封裝成字元串對象
//String s3 = new String(chs,0,chs.length);
String s3 = new String(chs, 1, 3);
System.out.println("s3:" + s3);
System.out.println("---------");
//方式 4
String s4 = "hello";
System.out.println("s4:" + s4);
}
}
1.3 String 的特點
- 通過構造方法建立字元串對象
String s = new String(“hello”);
- 直接指派建立字元串對象
String s = “hello”;
- 差別是什麼?(面試的經典問題,String s = new String(“hello”);是幾個對象?)
package com.study.demo.stringdemo;
/**
* @Auther: lds
* @Date: 2018/11/23 14:47
* @Description:
*/
public class StringDemo02 {
public static void main(String[] args) {
String s1 = new String("hello");
String s2 = "hello";
System.out.println("s1:" + s1);
System.out.println("s2:" + s2);
System.out.println("s1==s2:" + (s1 == s2)); //false
String s3 = "hello";
System.out.println("s1==s3:" + (s1 == s3)); //false
System.out.println("s2==s3:" + (s2 == s3)); //true
}
}
代碼很直覺的反映了,s1和s2不相等,這裡所說的相等,不僅僅是數值上面的相等,還有它們的實體位址是不是相等的!
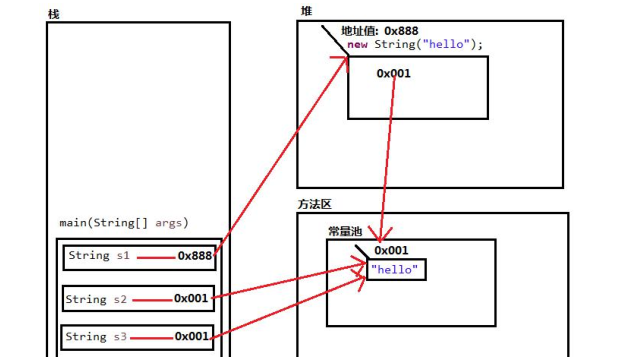
1.4 常用方法
- 判斷功能方法
- boolean equals(Object obj):比較字元串的内容是否相同
- boolean equalsIgnoreCase(String str):比較字元串的内容是否相同,忽略大小寫
- boolean startsWith(String str):判斷字元串對象是否以指定的 str 開頭
- boolean endsWith(String str):判斷字元串對象是否以指定的 str 結尾
package com.study.demo.stringdemo;
/**
* @Auther: lds
* @Date: 2018/11/23 15:00
* @Description:
*/
public class StringDemo03 {
public static void main(String[] args) {
//建立字元串對象
String s1 = "hello";
String s2 = "hello";
String s3 = "Hello";
//boolean equals(Object obj):比較字元串的内容是否相同
System.out.println(s1.equals(s2));
System.out.println(s1.equals(s3));
System.out.println("-----------");
//boolean equalsIgnoreCase(String str):比較字元串的内容是否相同,忽略大小寫
System.out.println(s1.equalsIgnoreCase(s2));
System.out.println(s1.equalsIgnoreCase(s3));
System.out.println("-----------");
//boolean startsWith(String str):判斷字元串對象是否以指定的 str 開頭
System.out.println(s1.startsWith("he"));
System.out.println(s1.startsWith("ll"));
}
}
例:模拟使用者登入案例,給三次機會,并提示還有幾次
package com.study.demo.stringdemo;
import java.util.Scanner;
/**
* @Auther: lds
* @Date: 2018/11/23 15:31
* @Description:
*/
public class StringDemo04 {
public static void main(String[] args) {
//定義兩個字元串對象,用于存儲已經存在的使用者名和密碼
String username = "admin";
String password = "admin";
//給三次機會,用 for 循環實作
for (int i = 0; i < 3; i++) {
//鍵盤錄入使用者名和密碼
Scanner sc = new Scanner(System.in);
System.out.println("請輸入使用者名:");
String name = sc.nextLine();
System.out.println("請輸入密碼:");
String pwd = sc.nextLine();
//拿鍵盤錄入的使用者名和密碼和已經存在的使用者名和密碼進行比較
if (username.equals(name) && password.equals(pwd)) {
System.out.println("登入成功");
break;
} else {
if ((2 - i) == 0) {
System.out.println("使用者名和密碼被鎖定,請與管理者聯系!");
} else {
System.out.println("登入失敗,你還有" + (2 - i) + "次機會!");
}
}
}
}
}
- 擷取功能方法
- int length():擷取字元串的長度,其實也就是字元個數
- char charAt(int index):擷取指定索引處的字元
- int indexOf(String str):擷取 str 在字元串對象中第一次出現的索引,沒有傳回-1
- String substring(int start):從 start 開始截取字元串到字元串結尾
- String substring(int start,int end):從 start 開始,到 end 結束截取字元串;包括 start,不包括 end
package com.study.demo.stringdemo;
/**
* @Auther: lds
* @Date: 2018/11/23 16:07
* @Description:
*/
public class StringDemo05 {
public static void main(String[] args) {
//建立字元串對象
String s = "helloworld";
//int length():擷取字元串的長度,其實也就是字元個數
System.out.println(s.length());
System.out.println("--------");
//char charAt(int index):擷取指定索引處的字元
System.out.println(s.charAt(0));
System.out.println(s.charAt(1));
System.out.println("--------");
//int indexOf(String str):擷取 str 在字元串對象中第一次出現的索引,沒有傳回-1
System.out.println(s.indexOf("l"));
System.out.println(s.indexOf("owo"));
System.out.println(s.indexOf("ak"));
System.out.println("--------");
//String substring(int start):從 start 開始截取字元串到字元串結尾
System.out.println(s.substring(0));
System.out.println(s.substring(5));
System.out.println("--------");
//String substring(int start,int end):從 start 開始,到 end 結束截取字元串。包括 start,不包括 end
System.out.println(s.substring(0, s.length()));
System.out.println(s.substring(3, 8));
}
}
- 轉換功能方法
- char[] toCharArray():把字元串轉換為字元數組
- String toLowerCase():把字元串轉換為小寫字元串
- String toUpperCase():把字元串轉換為大寫字元串
package com.study.demo.stringdemo;
import java.util.Scanner;
/**
* @Auther: lds
* @Date: 2018/11/23 16:11
* @Description:
*/
public class StringDemo06 {
public static void main(String[] args) {
//鍵盤錄入一個字元串
Scanner sc = new Scanner(System.in);
System.out.println("請輸入一個字元串:");
String s = sc.nextLine();
//截取首字母
String s1 = s.substring(0, 1);
//截取除了首字母以外的字元串
String s2 = s.substring(1);
//2 轉大寫+3 轉小寫
String s3 = s1.toUpperCase()+s2.toLowerCase();
//輸出即可
System.out.println("s3:"+s3);
}
}
- 去除空格和分割功能方法
- String trim()去除字元串兩端空格
- String[] split(String str)按照指定符号分割字元串
package com.study.demo.stringdemo;
/**
* @Auther: lds
* @Date: 2018/11/23 16:19
* @Description:
*/
public class StringDemo07 {
public static void main(String[] args) {
//建立字元串對象
String s1 = "helloworld";
String s2 = " helloworld ";
String s3 = " hello world ";
System.out.println("---" + s1 + "---");
System.out.println("---" + s1.trim() + "---");
System.out.println("---" + s2 + "---");
System.out.println("---" + s2.trim() + "---");
System.out.println("---" + s3 + "---");
System.out.println("---" + s3.trim() + "---");
System.out.println("-------------------");
//String[] split(String str)
//建立字元串對象
String s4 = "aa,bb,cc";
String[] strArray = s4.split(",");
for (int x = 0; x < strArray.length; x++) {
System.out.println(strArray[x]);
}
}
}
2.1 StringBuilder概述
我們如果對字元串進行拼接操作,每次拼接,都會建構一個新的 String 對象,既耗時,又浪費空間。而 StringBuilder 就可以解決這個問題。
2.2 StringBuilder和String的差別
- String 的内容是固定的。
- StringBuilder 的内容是可變的。
2.3 構造方法
- public StringBuilder()
- 和構造方法相關的方法
- public int capacity():傳回目前容量,理論值
- public int length():傳回長度(字元數),實際值
package com.study.demo.stringbuilder;
/**
* @Auther: lds
* @Date: 2018/11/23 16:30
* @Description:
*/
public class StringBuilderDemo01 {
public static void main(String[] args) {
//建立對象
StringBuilder builder = new StringBuilder();
System.out.println("builder:" + builder);
System.out.println("builder.capacity():" + builder.capacity());
System.out.println("builder.length():" + builder.length());
}
}
2.4 常用方法
- 添加功能
- public StringBuilder append(任意類型):添加資料,并傳回自身對象
- 反轉功能
- public StringBuilder reverse()
package com.study.demo.stringbuilder;
/**
* @Auther: lds
* @Date: 2018/11/23 16:35
* @Description:
*/
public class StringBuilderDemo02 {
public static void main(String[] args) {
//建立對象
StringBuilder builder = new StringBuilder();
//public StringBuilder append(任意類型)
//StringBuilder builder2 = builder.append("hello");
/*
System.out.println("builder:"+builder);
System.out.println("builder2:"+builder2);
System.out.println(builder == builder2); //true
*/
/*
builder.append("hello");
builder.append("world");
builder.append(true);
builder.append(100);
*/
//鍊式程式設計
builder.append("hello").append("world").append(true).append(100);
System.out.println("builder:" + builder);
//public StringBuilder reverse()
builder.reverse();
System.out.println("builder:" + builder);
}
}
- StringBuilder與 String 的互相轉換
- StringBuilder -- String
- public String toString():通過 toString()就可以實作把 StringBuilder 轉成 String
- String -- StringBuilder
- StringBuilder(String str):通過構造方法就可以實作把 String 轉成 StringBuilde
package com.study.demo.stringbuilder;
/**
* @Auther: lds
* @Date: 2018/11/23 16:39
* @Description:
*/
public class StringBuilderDemo03 {
public static void main(String[] args) {
//StringBuilder -- String
/*
StringBuilder builder = new StringBuilder();
builder.append("hello").append("world");
String s = builder.toString();
System.out.println(s);
*/
//String -- StringBuilder
String s = "helloworld";
StringBuilder builder = new StringBuilder(s);
System.out.println(builder);
}
}
例:判斷對稱字元串,"abc"不是對稱字元串,"aba"、"abba"、"aaa"、"mnanm"是對稱字元串
package com.study.demo.stringbuilder;
import java.util.Scanner;
/**
* @Auther: lds
* @Date: 2018/11/23 17:19
* @Description:
*/
public class StringBuilderDemo04 {
public static void main(String[] args) {
//1:鍵盤錄入一個字元串
Scanner sc = new Scanner(System.in);
System.out.println("請輸入一個字元串:");
String s = sc.nextLine();
//3:調用方法
boolean b = isSymmetry(s);
//4:輸出結果
System.out.println("b:" + b);
}
/*
* 2:寫方法實作判斷一個字元串是否是對稱字元串
* 兩個明确:
* 傳回值類型:boolean
* 參數清單:String s
*/
public static boolean isSymmetry(String s) {
//把字元串反轉,和反轉前的字元串進行比較,如果内容相同,就說明是對稱字元串
StringBuilder builder = new StringBuilder(s);
builder.reverse();
String result = builder.toString();
return result.equals(s);
}
}