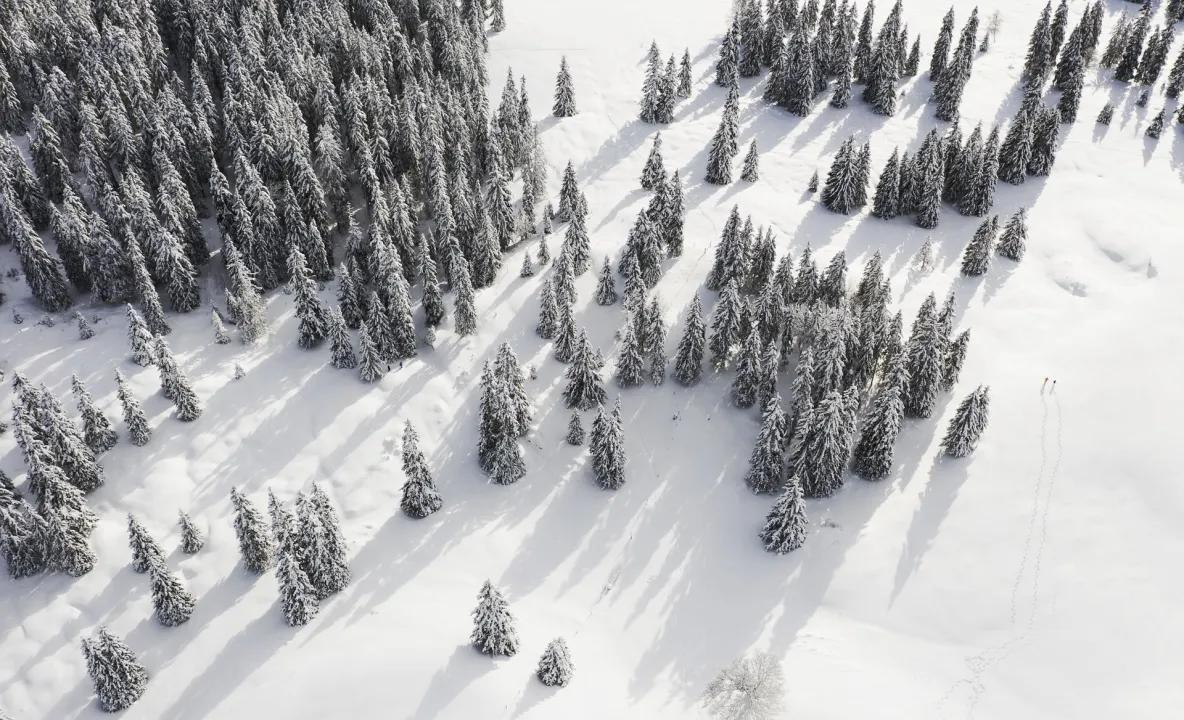
Spring MVC執行流程
Spirng MVC的執行流程是一個非常重要的知識點,它可以讓我們很清楚的了解Spring MVC是如何工作的,後面我們會通過源碼的方式整個流程以及涉及到的元件。再開始分析之前,我打算基于Servlet手寫一個Spirng MVC,這樣你就能明白大概的執行流程,不至于在看源碼的時候太暈。
定義注解
@Target(ElementType.TYPE)
@Retention(RetentionPolicy.RUNTIME)
public @interface Controller {
String value() default "";
}
@Target({ElementType.TYPE, ElementType.METHOD})
@Retention(RetentionPolicy.RUNTIME)
public @interface RequestMapping {
String value() default "";
}
定義中央控制器
基本上所有的邏輯都在這個類中,主要流程如下
- 建立DispatcherServlet的時候,tomcat會調用init()方法,在裡面初始化url和對應的處理方法的映射關系
- 當有請求來的時候,從uriInvokeInfoMap中拿對應的方法,如果有對應的方法,反射調用方法,拿到頁面名字,拼接頁面位址,轉發到相應的頁面,否則傳回404
@WebServlet(urlPatterns="/", loadOnStartup = 1)
public class DispatcherServlet extends HttpServlet {
// 儲存所有的handler
private List<Object> beanList = new ArrayList<>();
// 儲存 uri 和 handler 的映射關系
private Map<String, HandlerMethod> uriHandlerMethodMap = new HashMap<>();
private static final String SLASH = "/";
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
doPost(req, resp);
}
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
String uri = req.getRequestURI();
String contextPath = req.getContextPath();
// 去掉項目路徑
uri = uri.replace(contextPath, "");
System.out.println(uri);
if (uri == null) {
return;
}
HandlerMethod handlerMethod = uriHandlerMethodMap.get(uri);
if (handlerMethod == null) {
resp.getWriter().write("404");
return;
}
String pageName = (String)methodInvoke(handlerMethod.getBean(), handlerMethod.getMethod());
viewResolver(pageName, req, resp);
}
// 視圖解析器
public void viewResolver(String pageName, HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
String prefix = "/";
String suffix = ".jsp";
req.getRequestDispatcher(prefix + pageName + suffix).forward(req, resp);
}
// 反射執行方法
private Object methodInvoke(Object object, Method method) {
try {
return method.invoke(object);
} catch (Exception e) {
e.printStackTrace();
return null;
}
}
@Override
public void init() throws ServletException {
// 擷取指定包下的Class對象
List<Class<?>> classList = ClassUtil.getAllClassByPackageName("com.javashitang.controller");
// 找到所有标注了@Controller的類
findAllConrollerClass(classList);
// 初始化 uri 和 handler 的映射關系
handlerMapping();
}
public void findAllConrollerClass(List<Class<?>> list) {
list.forEach(bean -> {
// 将被@Controller注解修飾的類放到beanList
if (bean.isAnnotationPresent(Controller.class)) {
try {
beanList.add(bean.newInstance());
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
// 根據url找到相應的處理類
public void handlerMapping() {
for (Object bean : beanList) {
Class<? extends Object> classInfo = bean.getClass();
// 擷取類上的@RequestMapping資訊
RequestMapping beanRequestMapping = classInfo.getDeclaredAnnotation(RequestMapping.class);
String baseUrl = beanRequestMapping != null ? beanRequestMapping.value() : "";
Method[] methods = classInfo.getDeclaredMethods();
for (Method method : methods) {
System.out.println(method.getName());
// 擷取方法上的@RequestMapping資訊
RequestMapping methodRequestMapping = method.getDeclaredAnnotation(RequestMapping.class);
if (methodRequestMapping != null) {
String requestUrl = SLASH + baseUrl + SLASH + methodRequestMapping.value();
// 為了處理@Controller和@RequestMapping value 中加了 / 字首的情況
requestUrl = requestUrl.replaceAll("/+", "/");
HandlerMethod handlerMethod = new HandlerMethod(bean, method);
uriHandlerMethodMap.put(requestUrl, handlerMethod);
}
}
}
}
}
将方法及其對應的類做了一下封裝
public class HandlerMethod {
private Object bean;
private Method method;
public HandlerMethod(Object bean, Method method) {
this.bean = bean;
this.method = method;
}
}
開始測試
@Controller
@RequestMapping("index")
public class IndexController {
@RequestMapping("user")
public String user() {
return "user";
}
}
通路如下連接配接,頁面正常顯示
http://localhost:8080/show/index/user