自定義cell:frame,autolayout,xib,storyboard,不等高問題
利用frame和autolayout自定義cell
建立一個繼承自 UITableViewCell
的子類,比如LJTgCell
UITableViewCell
@interface XMGTgCell : UITableViewCell
@end
在LJTgCell.m檔案中
- 重寫
方法-initWithStyle:reuseIdentifier:
- 在這個方法中添加所有需要顯示的子控件
- 給子控件做一些初始化設定(設定字型、文字顔色等)
- 注意:如果是autolayout就要
添加子控件的完整限制
/**
* 在這個方法中添加所有的子控件
*/
- (instancetype)initWithStyle:(UITableViewCellStyle)style reuseIdentifier:(NSString *)reuseIdentifier
{
if (self = [super initWithStyle:style reuseIdentifier:reuseIdentifier]) {
// ......
}
return self;
}
- 重寫
方法-layoutSubviews
- 一定要調用
[super layoutSubviews]
- 在這個方法中計算和設定所有子控件的frame
- 一定要調用
/**
* 在這個方法中計算所有子控件的frame
*/
- (void)layoutSubviews
{
[super layoutSubviews]; / / 這句話很容易漏寫
// ......
}
在LJTgCell.h檔案中提供一個模型屬性,比如LJTg模型
@class LJTg;
@interface LJTgCell : UITableViewCell
/** 團購模型資料 */
@property (nonatomic, strong) LJTg *tg;
@end
在LJTgCell.m中重寫模型屬性的set方法
- 在set方法中給子控件設定模型資料
- (void)setTg:(XMGTg *)tg
{
_tg = tg;
// .......
}
在控制器中
- 實作資料源方法
// 有多少組
- (NSInteger)numberOfSectionsInTableView:(UITableView *)tableView
// 每組有多少行
- (NSInteger)tableView:(UITableView *)tableView
numberOfRowsInSection:(NSInteger)section
// 每行裡面的内容
- (UITableViewCell *)tableView:(UITableView *)tableView
cellForRowAtIndexPath:(NSIndexPath *)indexPath
- 注冊cell的類型(frame版)
[self.tableView registerC lass:[LJTgCell class] forCellReuseIdentifier:ID];
- 注意:注冊cell的類型(xib版)
[self.tableView registerNib:[UINib nibWithNibName:
NSStringFromClass([LJTgCell class]) bundle:nil]forCellReuseIdentifier:ID];
- 給cell傳遞模型資料
- (UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath
{
// 通路緩存池
XMGTgCell *cell = [tableView dequeueReusableCellWithIdentifier:ID];
// 設定資料(傳遞模型資料)
cell.tg = self.tgArray[indexPath.row];
return cell;
}
利用xib自定義cell
建立一個繼承自 UITableViewCell
的子類,比如LJTgCell
UITableViewCell
@interface LJTgCell : UITableV iewCell
@end
建立一個xib檔案(檔案名最好跟類名一緻,比如LJTgCell.xib)
- 可以在建立cell類上直接建立對應的xib檔案
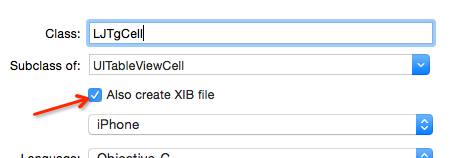
- 修改cell的class為LJTgCell
- 綁定循環利用辨別
- 添加子控件,設定子控件限制
- 将子控件連線到類擴充中
@interface LJTgCell()
@property (weak, nonatomic) IBOutlet UIImageView *iconImageView;
@property (weak, nonatomic) IBOutlet UILabel *titleLabel;
@property (weak, nonatomic) IBOutlet UILabel *priceLabel;
@property (weak, nonatomic) IBOutlet UILabel *buyCountLabel;
@end
在LJTgCell.h檔案中提供一個模型屬性,比如LJTg模型
@class LJTg;
@interface LJTgCell : UITableViewCell
/** 團購模型資料 */
@property (nonatomic, strong) LJTg *tg;
@end
在LJTgCell.m中重寫模型屬性的set方法
- 在set方法中給子控件設定模型資料
- (void)setTg:(Tg *)tg
{
_tg = tg;
// .......
}
在控制器中
- 注冊xib檔案(xib版)
[self.tableView registerNib:[UINibnibWithNibName:
NSStringFromClass([LJTgCell class]) bundle:nil] forCellReuseIdentifier:ID];
- 給cell傳遞模型資料
- (UITableViewCell *)tableView:(UITableView *)tableView
cellForRowAtIndexPath:(NSIndexPath *)indexPath
{
// 通路緩存池
LJTgCell *cell = [tableView dequeueReusableCellWithIdentifier:ID];
// 設定資料(傳遞模型資料)
cell.tg = self.tgs[indexPath.row];
return cell;
}
利用stroyboard自定義cell
建立一個繼承自 UITableViewCell
的子類,比如LJTgCell
UITableViewCell
@interface LJTgCell : UITableViewCell
@end
在storyboard檔案中,找到UITableView裡面的cell(動态cell)
- 修改cell的class為LJTgCell
- 綁定循環利用辨別(此時就不用在控制器中注冊了)
- 添加子控件,設定子控件限制
- 将子控件連線到類擴充中
@interface LJTgCell()
@property (weak, nonatomic) IBOutlet UIImageView *iconImageView;
@property (weak, nonatomic) IBOutlet UILabel *titleLabel;
@property (weak, nonatomic) IBOutlet UILabel *priceLabel;
@property (weak, nonatomic) IBOutlet UILabel *buyCountLabel;
@end
cell不等高的方法(2種)
方法一:直接在控制器設定height的viewDidLoad方法中設定
- (void)viewDidLoad {
[super viewDidLoad];
// 告訴tableView所有cell的真實高度是自動計算(根據設定的限制來計算)
self.tableView.rowHeight = UITableViewAutomaticDimension;
// 告訴tableView所有cell的估算高度
self.tableView.estimatedRowHeight = 44;
}
方法二:給模型增加frame資料
在LJStatus.m中
- 所有子控件的frame
- cell的高度
@interface LJStatus : NSObject
/**** 文字\圖檔資料 ****/
// .....
/**** frame資料 ****/
/** 頭像的frame */
@property (nonatomic, assign) CGRect iconFrame;
// .....
/** cell的高度 */
@property (nonatomic, assign) CGFloat cellH;
@end
- 重寫模型cellH屬性的get方法
- (CGFloat)cellH
{
if (_cellH == 0) {
// ... 計算所有子控件的frame、cell的高度
}
return _cellH;
}
在控制器中
- 實作一個傳回cell高度的代理方法
- 在這個方法中傳回indexPath位置對應cell的高度
/**
* 傳回每一行cell的具體高度
*/
- (CGFloat)tableView:(UITableView *)tableView heightForRowAtIndexPath:(NSIndexPath *)indexPath
{
LJStatus *status = self.statuses[indexPath.row];
return status.cellH;
}
- 給cell傳遞模型資料
- (UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath
{
static NSString *ID = @"status";
// 通路緩存池
LJStatusCell *cell = [tableView dequeueReusableCellWithIdentifier:ID];
// 設定資料(傳遞模型資料)
cell.status = self.statuses[indexPath.row];
return cell;
}
建立一個繼承自 UITableViewCell
的子類,比如LJStatusCell
UITableViewCell
@interface LJStatusCell : UITableViewCell
@end
在LJStatusCell.m檔案中
- 重寫
方法-initWithStyle:reuseIdentifier:
- 在這個方法中添加所有可能顯示的子控件
- 給子控件做一些初始化設定(設定字型、文字顔色等)
/**
* 在這個方法中添加所有的子控件
*/
- (instancetype)initWithStyle:(UITableViewCellStyle)style reuseIdentifier:(NSString *)reuseIdentifier
{
if (self = [super initWithStyle:style reuseIdentifier:reuseIdentifier]) {
// ......
}
return self;
}
在LJStatusCell.h檔案中提供一個模型屬性,比如LJStatus模型
@class LJStatus;
@interface LJStatusCell : UITableViewCell
/** 團購模型資料 */
@property (nonatomic, strong) LJStatus *status;
@end
在LJStatusCell.m檔案中重寫模型屬性的set方法
- 在set方法中給子控件設定模型資料
- (void)setStatus:(LJStatus *)status
{
_status = status;
// .......
}
重寫 -layoutSubviews
方法
-layoutSubviews
- 一定要調用
[super layoutSubviews]
- 在這個方法中
所有子控件的frame設定
/**
* 在這個方法中設定所有子控件的frame
*/
- (void)layoutSubviews
{
[super layoutSubviews];
// ......
}
轉載于:https://www.cnblogs.com/LongLJ/p/4995921.html