鍏充簬@Transactional娉ㄨВ锛?h5>娣誨姞浜嬪姟娉ㄨВ
1銆佷嬌鐢?propagation 鎸囧畾浜嬪姟鐨勪紶鎾涓? 鍗沖綋鍓嶇殑浜嬪姟鏂規硶琚彟澶栦竴涓簨鍔℃柟娉曡皟鐢ㄦ椂銆傚浣曚嬌鐢ㄤ簨鍔? 榛樿鍙栧€間負 REQUIRED, 鍗充嬌鐢ㄨ皟鐢ㄦ柟娉曠殑浜嬪姟REQUIRES_NEW: 浜嬪姟鑷繁鐨勪簨鍔? 璋冪敤鐨勪簨鍔℃柟娉曠殑浜嬪姟琚寕璧楓€?
2銆佷嬌鐢?isolation 鎸囧畾浜嬪姟鐨勯殧绂葷駭鍒? 鏈€甯哥敤鐨勫彇鍊間負 READ_COMMITTED銆?
3銆侀粯璁ゆ儏鍐典笅 Spring 鐨勫0鏄庡紡浜嬪姟瀵規墍鏈夌殑杩愯鏃跺紓甯歌繘琛屽洖婊? 涔熷彲浠ラ€氳繃瀵瑰簲鐨勫睘鎬ц繘琛岃缃? 閫氬父鎯呭喌涓嬪幓榛樿鍊煎嵆鍙€?銆佷嬌鐢?readOnly 鎸囧畾浜嬪姟鏄惁涓哄彧璇? 琛ㄧず杩欎釜浜嬪姟鍙鍙栨暟鎹絾涓嶆洿鏂版暟鎹紝杩欐牱鍙互甯姪鏁版嵁搴撳紩鎿庝紭鍖栦簨鍔? 鑻ョ湡鐨勬槸涓€涓彧璇誨彇鏁版嵁搴撳€肩殑鏂規硶, 搴旇缃?readOnly=true
5銆佷嬌鐢?timeout 鎸囧畾寮哄埗鍥炴粴涔嬪墠浜嬪姟鍙互鍗犵敤鐨勬椂闂淬€?
涓漢鐤戦棶鏈変竴浜涳紝缁撳熬鏉ヨ锛岀敤鏁欑▼渚嬪瓙鏉ヨ鍚с€?
鎴戠殑浠g爜濡備笅锛?/h4> BookShopDao鎺ュ彛
package com.demo.spring.bean;
public interface BookShopDao {
//鏍規嵁涔︾殑缂栧彿杩斿洖涔︾殑鍗曚環
public int findBookPriceByIsbn(String isbn);
//鏍規嵁涔︾殑缂栧彿杩斿洖杈撶殑搴撳瓨
public void updateBookStock(String isbn);
//鏇存柊鐢ㄦ埛璐︽埛浣欓
public void updateUserAccount(String username, int price);
}
澶嶅埗 瀹炵幇绫?/h5> package com.demo.spring.bean;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.jdbc.core.JdbcTemplate;
import org.springframework.stereotype.Repository;
@Repository("bookShopDao")
public class BookShopDaoImpl implements BookShopDao {
@Autowired
private JdbcTemplate jdbcTemplate;
@Override
public int findBookPriceByIsbn(String isbn) {
// TODO Auto-generated method stub
String sql = "SELECT price FROM book WHERE isbn=?";
Integer price = jdbcTemplate.queryForObject(sql, Integer.class, isbn);
return price;
}
@Override
public void updateBookStock(String isbn) {
// TODO Auto-generated method stub
String sql = "SELECT stock FROM book_stock WHERE isbn= ? ";
Integer stock = jdbcTemplate.queryForObject(sql, Integer.class, isbn);
if (stock == 0) {
throw new BookStockException("搴撳瓨涓嶈凍锛侊紒");
}
String sql1 = "UPDATE book_stock SET stock=stock-1 WHERE isbn=?";
jdbcTemplate.update(sql1, isbn);
}
@Override
public void updateUserAccount(String username, int price) {
// TODO Auto-generated method stub
String sql1 = "SELECT balance FROM account WHERE username= ? ";
Integer account = jdbcTemplate.queryForObject(sql1, Integer.class,
username);
if (account < price) {
throw new AccountException("浣欓涓嶈凍锛侊紒");
}
String sql = "UPDATE account SET balance=balance-? WHERE username=?";
jdbcTemplate.update(sql, price, username);
}
}
澶嶅埗 BookShopService鎺ュ彛
package com.demo.spring.bean;
public interface BookShopService {
//璐功
public void purchase(String username, String isbn);
}
澶嶅埗 瀹炵幇绫?/h4> package com.demo.spring.bean;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import org.springframework.transaction.annotation.Transactional;
@Service("bookShopService")
public class BookShopServiceImpl implements BookShopService {
@Autowired
private BookShopDao bookShopDao;
// @Transactional(propagation=Propagation.REQUIRES_NEW,isolation=Isolation.READ_COMMITTED,readOnly=true,timeout=5,noRollbackFor=AccountException.class)
@Transactional
@Override
public void purchase(String username, String isbn) {
try {
Thread.sleep(5000);
} catch (InterruptedException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
// TODO Auto-generated method stub
int price = bookShopDao.findBookPriceByIsbn(isbn);
bookShopDao.updateBookStock(isbn);
bookShopDao.updateUserAccount(username, price);
}
}
澶嶅埗 Cashier鎵歸噺璐功鎺ュ彛
package com.demo.spring.bean;
import java.util.List;
public interface Cashier {
//鎵歸噺璐功
public void checkout(String username, List<String> isbns);
}
澶嶅埗 瀹炵幇绫?/h4> package com.demo.spring.bean;
import java.util.List;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
@Service("cashier")
public class CashierImpl implements Cashier {
@Autowired
private BookShopService bookShopService;
// @Transactional
@Override
public void checkout(String username, List<String> isbns) {
// TODO Auto-generated method stub
for (String isbn : isbns) {
bookShopService.purchase(username, isbn);
}
}
}
澶嶅埗 璐︽埛浣欓涓嶈凍寮傚父锛堣嚜瀹氫箟寮傚父锛?/h4> package com.demo.spring.bean;
public class AccountException extends RuntimeException {
/**
*
*/
private static final long serialVersionUID = 1L;
public AccountException() {
super();
// TODO Auto-generated constructor stub
}
public AccountException(String message, Throwable cause,
boolean enableSuppression, boolean writableStackTrace) {
super(message, cause, enableSuppression, writableStackTrace);
// TODO Auto-generated constructor stub
}
public AccountException(String message, Throwable cause) {
super(message, cause);
// TODO Auto-generated constructor stub
}
public AccountException(String message) {
super(message);
// TODO Auto-generated constructor stub
}
public AccountException(Throwable cause) {
super(cause);
// TODO Auto-generated constructor stub
}
}
澶嶅埗 搴撳瓨涓嶈凍寮傚父锛堣嚜瀹氫箟寮傚父锛?/h4> package com.demo.spring.bean;
public class BookStockException extends RuntimeException {
private static final long serialVersionUID = 1L;
public BookStockException() {
super();
// TODO Auto-generated constructor stub
}
public BookStockException(String message, Throwable cause,
boolean enableSuppression, boolean writableStackTrace) {
super(message, cause, enableSuppression, writableStackTrace);
// TODO Auto-generated constructor stub
}
public BookStockException(String message, Throwable cause) {
super(message, cause);
// TODO Auto-generated constructor stub
}
public BookStockException(String message) {
super(message);
// TODO Auto-generated constructor stub
}
public BookStockException(Throwable cause) {
super(cause);
// TODO Auto-generated constructor stub
}
}
澶嶅埗 娴嬭瘯绫?/h4> package com.demo.spring.bean;
import java.util.Arrays;
import org.junit.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class MainTest {
private ApplicationContext ctx;
@Autowired
private Cashier cashier;
{
ctx=new ClassPathXmlApplicationContext("bean.xml");
cashier=(Cashier) ctx.getBean("cashier");
}
@Test
public void test(){
// System.out.println(bookShopDao.findBookPriceByIsbn("1001"));
cashier.checkout("rongrong", Arrays.asList("1001","1002"));
}
}
澶嶅埗 bean鏂囦歡
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:aop="http://www.springframework.org/schema/aop"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:tx="http://www.springframework.org/schema/tx"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.2.xsd
http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-4.1.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-4.1.xsd
http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx-4.1.xsd">
<!-- 瑁呰澆鑷瀵煎叆鐨勫寘 -->
<context:component-scanbase-package="com.demo.spring.bean"></context:component-scan>
<!-- 寮曞叆澶栭儴鏁版嵁婧?-->
<context:property-placeholder location="classpath:db.properties"/>
<!-- 閰嶇疆mysql鏁版嵁婧?-->
<bean id="datasource" class="org.springframework.jdbc.datasource.DriverManagerDataSource">
<property name="driverClassName" value="${db.driverClassName}"></property>
<property name="url" value="${db.url}"></property>
<property name="username" value="${db.username}"></property>
<property name="password" value="${db.password}"></property>
</bean>
<!-- 閰嶇疆jdbc妯℃澘 -->
<bean id="jdbcTemplate" class="org.springframework.jdbc.core.JdbcTemplate">
<property name="dataSource" ref="datasource"></property>
</bean>
<!-- 閰嶇疆浜嬪姟绠$悊鍣?-->
<bean id="transactionManagertest" class="org.springframework.jdbc.datasource.DataSourceTransactionManager">
<property name="dataSource" ref="datasource"></property>
</bean>
<!-- 鍚敤浜嬪姟娉ㄨВ -->
<tx:annotation-driven transaction-manager="transactionManagertest"/>
</beans>
澶嶅埗 鏁版嵁婧?/h4> db.driverClassName=com.mysql.jdbc.Driver
db.url=jdbc:mysql://localhost:3306/spring
db.username=root
db.password=root
澶嶅埗
spring事物中的傳播及隔離
package com.demo.spring.bean;
public interface BookShopDao {
//鏍規嵁涔︾殑缂栧彿杩斿洖涔︾殑鍗曚環
public int findBookPriceByIsbn(String isbn);
//鏍規嵁涔︾殑缂栧彿杩斿洖杈撶殑搴撳瓨
public void updateBookStock(String isbn);
//鏇存柊鐢ㄦ埛璐︽埛浣欓
public void updateUserAccount(String username, int price);
}
package com.demo.spring.bean;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.jdbc.core.JdbcTemplate;
import org.springframework.stereotype.Repository;
@Repository("bookShopDao")
public class BookShopDaoImpl implements BookShopDao {
@Autowired
private JdbcTemplate jdbcTemplate;
@Override
public int findBookPriceByIsbn(String isbn) {
// TODO Auto-generated method stub
String sql = "SELECT price FROM book WHERE isbn=?";
Integer price = jdbcTemplate.queryForObject(sql, Integer.class, isbn);
return price;
}
@Override
public void updateBookStock(String isbn) {
// TODO Auto-generated method stub
String sql = "SELECT stock FROM book_stock WHERE isbn= ? ";
Integer stock = jdbcTemplate.queryForObject(sql, Integer.class, isbn);
if (stock == 0) {
throw new BookStockException("搴撳瓨涓嶈凍锛侊紒");
}
String sql1 = "UPDATE book_stock SET stock=stock-1 WHERE isbn=?";
jdbcTemplate.update(sql1, isbn);
}
@Override
public void updateUserAccount(String username, int price) {
// TODO Auto-generated method stub
String sql1 = "SELECT balance FROM account WHERE username= ? ";
Integer account = jdbcTemplate.queryForObject(sql1, Integer.class,
username);
if (account < price) {
throw new AccountException("浣欓涓嶈凍锛侊紒");
}
String sql = "UPDATE account SET balance=balance-? WHERE username=?";
jdbcTemplate.update(sql, price, username);
}
}
澶嶅埗 BookShopService鎺ュ彛
package com.demo.spring.bean;
public interface BookShopService {
//璐功
public void purchase(String username, String isbn);
}
澶嶅埗 瀹炵幇绫?/h4> package com.demo.spring.bean;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import org.springframework.transaction.annotation.Transactional;
@Service("bookShopService")
public class BookShopServiceImpl implements BookShopService {
@Autowired
private BookShopDao bookShopDao;
// @Transactional(propagation=Propagation.REQUIRES_NEW,isolation=Isolation.READ_COMMITTED,readOnly=true,timeout=5,noRollbackFor=AccountException.class)
@Transactional
@Override
public void purchase(String username, String isbn) {
try {
Thread.sleep(5000);
} catch (InterruptedException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
// TODO Auto-generated method stub
int price = bookShopDao.findBookPriceByIsbn(isbn);
bookShopDao.updateBookStock(isbn);
bookShopDao.updateUserAccount(username, price);
}
}
澶嶅埗 Cashier鎵歸噺璐功鎺ュ彛
package com.demo.spring.bean;
import java.util.List;
public interface Cashier {
//鎵歸噺璐功
public void checkout(String username, List<String> isbns);
}
澶嶅埗 瀹炵幇绫?/h4> package com.demo.spring.bean;
import java.util.List;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
@Service("cashier")
public class CashierImpl implements Cashier {
@Autowired
private BookShopService bookShopService;
// @Transactional
@Override
public void checkout(String username, List<String> isbns) {
// TODO Auto-generated method stub
for (String isbn : isbns) {
bookShopService.purchase(username, isbn);
}
}
}
澶嶅埗 璐︽埛浣欓涓嶈凍寮傚父锛堣嚜瀹氫箟寮傚父锛?/h4> package com.demo.spring.bean;
public class AccountException extends RuntimeException {
/**
*
*/
private static final long serialVersionUID = 1L;
public AccountException() {
super();
// TODO Auto-generated constructor stub
}
public AccountException(String message, Throwable cause,
boolean enableSuppression, boolean writableStackTrace) {
super(message, cause, enableSuppression, writableStackTrace);
// TODO Auto-generated constructor stub
}
public AccountException(String message, Throwable cause) {
super(message, cause);
// TODO Auto-generated constructor stub
}
public AccountException(String message) {
super(message);
// TODO Auto-generated constructor stub
}
public AccountException(Throwable cause) {
super(cause);
// TODO Auto-generated constructor stub
}
}
澶嶅埗 搴撳瓨涓嶈凍寮傚父锛堣嚜瀹氫箟寮傚父锛?/h4> package com.demo.spring.bean;
public class BookStockException extends RuntimeException {
private static final long serialVersionUID = 1L;
public BookStockException() {
super();
// TODO Auto-generated constructor stub
}
public BookStockException(String message, Throwable cause,
boolean enableSuppression, boolean writableStackTrace) {
super(message, cause, enableSuppression, writableStackTrace);
// TODO Auto-generated constructor stub
}
public BookStockException(String message, Throwable cause) {
super(message, cause);
// TODO Auto-generated constructor stub
}
public BookStockException(String message) {
super(message);
// TODO Auto-generated constructor stub
}
public BookStockException(Throwable cause) {
super(cause);
// TODO Auto-generated constructor stub
}
}
澶嶅埗 娴嬭瘯绫?/h4> package com.demo.spring.bean;
import java.util.Arrays;
import org.junit.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class MainTest {
private ApplicationContext ctx;
@Autowired
private Cashier cashier;
{
ctx=new ClassPathXmlApplicationContext("bean.xml");
cashier=(Cashier) ctx.getBean("cashier");
}
@Test
public void test(){
// System.out.println(bookShopDao.findBookPriceByIsbn("1001"));
cashier.checkout("rongrong", Arrays.asList("1001","1002"));
}
}
澶嶅埗 bean鏂囦歡
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:aop="http://www.springframework.org/schema/aop"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:tx="http://www.springframework.org/schema/tx"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.2.xsd
http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-4.1.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-4.1.xsd
http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx-4.1.xsd">
<!-- 瑁呰澆鑷瀵煎叆鐨勫寘 -->
<context:component-scanbase-package="com.demo.spring.bean"></context:component-scan>
<!-- 寮曞叆澶栭儴鏁版嵁婧?-->
<context:property-placeholder location="classpath:db.properties"/>
<!-- 閰嶇疆mysql鏁版嵁婧?-->
<bean id="datasource" class="org.springframework.jdbc.datasource.DriverManagerDataSource">
<property name="driverClassName" value="${db.driverClassName}"></property>
<property name="url" value="${db.url}"></property>
<property name="username" value="${db.username}"></property>
<property name="password" value="${db.password}"></property>
</bean>
<!-- 閰嶇疆jdbc妯℃澘 -->
<bean id="jdbcTemplate" class="org.springframework.jdbc.core.JdbcTemplate">
<property name="dataSource" ref="datasource"></property>
</bean>
<!-- 閰嶇疆浜嬪姟绠$悊鍣?-->
<bean id="transactionManagertest" class="org.springframework.jdbc.datasource.DataSourceTransactionManager">
<property name="dataSource" ref="datasource"></property>
</bean>
<!-- 鍚敤浜嬪姟娉ㄨВ -->
<tx:annotation-driven transaction-manager="transactionManagertest"/>
</beans>
澶嶅埗 鏁版嵁婧?/h4> db.driverClassName=com.mysql.jdbc.Driver
db.url=jdbc:mysql://localhost:3306/spring
db.username=root
db.password=root
澶嶅埗
spring事物中的傳播及隔離
package com.demo.spring.bean;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import org.springframework.transaction.annotation.Transactional;
@Service("bookShopService")
public class BookShopServiceImpl implements BookShopService {
@Autowired
private BookShopDao bookShopDao;
// @Transactional(propagation=Propagation.REQUIRES_NEW,isolation=Isolation.READ_COMMITTED,readOnly=true,timeout=5,noRollbackFor=AccountException.class)
@Transactional
@Override
public void purchase(String username, String isbn) {
try {
Thread.sleep(5000);
} catch (InterruptedException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
// TODO Auto-generated method stub
int price = bookShopDao.findBookPriceByIsbn(isbn);
bookShopDao.updateBookStock(isbn);
bookShopDao.updateUserAccount(username, price);
}
}
package com.demo.spring.bean;
import java.util.List;
public interface Cashier {
//鎵歸噺璐功
public void checkout(String username, List<String> isbns);
}
package com.demo.spring.bean;
import java.util.List;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
@Service("cashier")
public class CashierImpl implements Cashier {
@Autowired
private BookShopService bookShopService;
// @Transactional
@Override
public void checkout(String username, List<String> isbns) {
// TODO Auto-generated method stub
for (String isbn : isbns) {
bookShopService.purchase(username, isbn);
}
}
}
澶嶅埗 璐︽埛浣欓涓嶈凍寮傚父锛堣嚜瀹氫箟寮傚父锛?/h4> package com.demo.spring.bean;
public class AccountException extends RuntimeException {
/**
*
*/
private static final long serialVersionUID = 1L;
public AccountException() {
super();
// TODO Auto-generated constructor stub
}
public AccountException(String message, Throwable cause,
boolean enableSuppression, boolean writableStackTrace) {
super(message, cause, enableSuppression, writableStackTrace);
// TODO Auto-generated constructor stub
}
public AccountException(String message, Throwable cause) {
super(message, cause);
// TODO Auto-generated constructor stub
}
public AccountException(String message) {
super(message);
// TODO Auto-generated constructor stub
}
public AccountException(Throwable cause) {
super(cause);
// TODO Auto-generated constructor stub
}
}
澶嶅埗 搴撳瓨涓嶈凍寮傚父锛堣嚜瀹氫箟寮傚父锛?/h4> package com.demo.spring.bean;
public class BookStockException extends RuntimeException {
private static final long serialVersionUID = 1L;
public BookStockException() {
super();
// TODO Auto-generated constructor stub
}
public BookStockException(String message, Throwable cause,
boolean enableSuppression, boolean writableStackTrace) {
super(message, cause, enableSuppression, writableStackTrace);
// TODO Auto-generated constructor stub
}
public BookStockException(String message, Throwable cause) {
super(message, cause);
// TODO Auto-generated constructor stub
}
public BookStockException(String message) {
super(message);
// TODO Auto-generated constructor stub
}
public BookStockException(Throwable cause) {
super(cause);
// TODO Auto-generated constructor stub
}
}
澶嶅埗 娴嬭瘯绫?/h4> package com.demo.spring.bean;
import java.util.Arrays;
import org.junit.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class MainTest {
private ApplicationContext ctx;
@Autowired
private Cashier cashier;
{
ctx=new ClassPathXmlApplicationContext("bean.xml");
cashier=(Cashier) ctx.getBean("cashier");
}
@Test
public void test(){
// System.out.println(bookShopDao.findBookPriceByIsbn("1001"));
cashier.checkout("rongrong", Arrays.asList("1001","1002"));
}
}
澶嶅埗 bean鏂囦歡
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:aop="http://www.springframework.org/schema/aop"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:tx="http://www.springframework.org/schema/tx"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.2.xsd
http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-4.1.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-4.1.xsd
http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx-4.1.xsd">
<!-- 瑁呰澆鑷瀵煎叆鐨勫寘 -->
<context:component-scanbase-package="com.demo.spring.bean"></context:component-scan>
<!-- 寮曞叆澶栭儴鏁版嵁婧?-->
<context:property-placeholder location="classpath:db.properties"/>
<!-- 閰嶇疆mysql鏁版嵁婧?-->
<bean id="datasource" class="org.springframework.jdbc.datasource.DriverManagerDataSource">
<property name="driverClassName" value="${db.driverClassName}"></property>
<property name="url" value="${db.url}"></property>
<property name="username" value="${db.username}"></property>
<property name="password" value="${db.password}"></property>
</bean>
<!-- 閰嶇疆jdbc妯℃澘 -->
<bean id="jdbcTemplate" class="org.springframework.jdbc.core.JdbcTemplate">
<property name="dataSource" ref="datasource"></property>
</bean>
<!-- 閰嶇疆浜嬪姟绠$悊鍣?-->
<bean id="transactionManagertest" class="org.springframework.jdbc.datasource.DataSourceTransactionManager">
<property name="dataSource" ref="datasource"></property>
</bean>
<!-- 鍚敤浜嬪姟娉ㄨВ -->
<tx:annotation-driven transaction-manager="transactionManagertest"/>
</beans>
澶嶅埗 鏁版嵁婧?/h4> db.driverClassName=com.mysql.jdbc.Driver
db.url=jdbc:mysql://localhost:3306/spring
db.username=root
db.password=root
澶嶅埗
spring事物中的傳播及隔離
package com.demo.spring.bean;
public class AccountException extends RuntimeException {
/**
*
*/
private static final long serialVersionUID = 1L;
public AccountException() {
super();
// TODO Auto-generated constructor stub
}
public AccountException(String message, Throwable cause,
boolean enableSuppression, boolean writableStackTrace) {
super(message, cause, enableSuppression, writableStackTrace);
// TODO Auto-generated constructor stub
}
public AccountException(String message, Throwable cause) {
super(message, cause);
// TODO Auto-generated constructor stub
}
public AccountException(String message) {
super(message);
// TODO Auto-generated constructor stub
}
public AccountException(Throwable cause) {
super(cause);
// TODO Auto-generated constructor stub
}
}
package com.demo.spring.bean;
public class BookStockException extends RuntimeException {
private static final long serialVersionUID = 1L;
public BookStockException() {
super();
// TODO Auto-generated constructor stub
}
public BookStockException(String message, Throwable cause,
boolean enableSuppression, boolean writableStackTrace) {
super(message, cause, enableSuppression, writableStackTrace);
// TODO Auto-generated constructor stub
}
public BookStockException(String message, Throwable cause) {
super(message, cause);
// TODO Auto-generated constructor stub
}
public BookStockException(String message) {
super(message);
// TODO Auto-generated constructor stub
}
public BookStockException(Throwable cause) {
super(cause);
// TODO Auto-generated constructor stub
}
}
澶嶅埗 娴嬭瘯绫?/h4> package com.demo.spring.bean;
import java.util.Arrays;
import org.junit.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class MainTest {
private ApplicationContext ctx;
@Autowired
private Cashier cashier;
{
ctx=new ClassPathXmlApplicationContext("bean.xml");
cashier=(Cashier) ctx.getBean("cashier");
}
@Test
public void test(){
// System.out.println(bookShopDao.findBookPriceByIsbn("1001"));
cashier.checkout("rongrong", Arrays.asList("1001","1002"));
}
}
澶嶅埗 bean鏂囦歡
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:aop="http://www.springframework.org/schema/aop"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:tx="http://www.springframework.org/schema/tx"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.2.xsd
http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-4.1.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-4.1.xsd
http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx-4.1.xsd">
<!-- 瑁呰澆鑷瀵煎叆鐨勫寘 -->
<context:component-scanbase-package="com.demo.spring.bean"></context:component-scan>
<!-- 寮曞叆澶栭儴鏁版嵁婧?-->
<context:property-placeholder location="classpath:db.properties"/>
<!-- 閰嶇疆mysql鏁版嵁婧?-->
<bean id="datasource" class="org.springframework.jdbc.datasource.DriverManagerDataSource">
<property name="driverClassName" value="${db.driverClassName}"></property>
<property name="url" value="${db.url}"></property>
<property name="username" value="${db.username}"></property>
<property name="password" value="${db.password}"></property>
</bean>
<!-- 閰嶇疆jdbc妯℃澘 -->
<bean id="jdbcTemplate" class="org.springframework.jdbc.core.JdbcTemplate">
<property name="dataSource" ref="datasource"></property>
</bean>
<!-- 閰嶇疆浜嬪姟绠$悊鍣?-->
<bean id="transactionManagertest" class="org.springframework.jdbc.datasource.DataSourceTransactionManager">
<property name="dataSource" ref="datasource"></property>
</bean>
<!-- 鍚敤浜嬪姟娉ㄨВ -->
<tx:annotation-driven transaction-manager="transactionManagertest"/>
</beans>
澶嶅埗 鏁版嵁婧?/h4> db.driverClassName=com.mysql.jdbc.Driver
db.url=jdbc:mysql://localhost:3306/spring
db.username=root
db.password=root
澶嶅埗
spring事物中的傳播及隔離
package com.demo.spring.bean;
import java.util.Arrays;
import org.junit.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class MainTest {
private ApplicationContext ctx;
@Autowired
private Cashier cashier;
{
ctx=new ClassPathXmlApplicationContext("bean.xml");
cashier=(Cashier) ctx.getBean("cashier");
}
@Test
public void test(){
// System.out.println(bookShopDao.findBookPriceByIsbn("1001"));
cashier.checkout("rongrong", Arrays.asList("1001","1002"));
}
}
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:aop="http://www.springframework.org/schema/aop"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:tx="http://www.springframework.org/schema/tx"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.2.xsd
http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-4.1.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-4.1.xsd
http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx-4.1.xsd">
<!-- 瑁呰澆鑷瀵煎叆鐨勫寘 -->
<context:component-scanbase-package="com.demo.spring.bean"></context:component-scan>
<!-- 寮曞叆澶栭儴鏁版嵁婧?-->
<context:property-placeholder location="classpath:db.properties"/>
<!-- 閰嶇疆mysql鏁版嵁婧?-->
<bean id="datasource" class="org.springframework.jdbc.datasource.DriverManagerDataSource">
<property name="driverClassName" value="${db.driverClassName}"></property>
<property name="url" value="${db.url}"></property>
<property name="username" value="${db.username}"></property>
<property name="password" value="${db.password}"></property>
</bean>
<!-- 閰嶇疆jdbc妯℃澘 -->
<bean id="jdbcTemplate" class="org.springframework.jdbc.core.JdbcTemplate">
<property name="dataSource" ref="datasource"></property>
</bean>
<!-- 閰嶇疆浜嬪姟绠$悊鍣?-->
<bean id="transactionManagertest" class="org.springframework.jdbc.datasource.DataSourceTransactionManager">
<property name="dataSource" ref="datasource"></property>
</bean>
<!-- 鍚敤浜嬪姟娉ㄨВ -->
<tx:annotation-driven transaction-manager="transactionManagertest"/>
</beans>
db.driverClassName=com.mysql.jdbc.Driver
db.url=jdbc:mysql://localhost:3306/spring
db.username=root
db.password=root
澶嶅埗 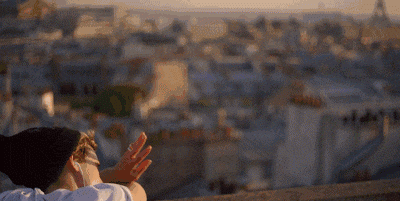
鐤戦棶濡備笅锛?/h4>
1銆佸墠鎻愭潯浠訛細鐢ㄦ埛鎸夌収1001锛?002杩欑椤哄簭鍘昏喘涔︼紝璋冪敤checkout鎺ュ彛鎵歸噺璐功 @Transactional娉ㄨВ纭疄婊¤凍鍘熷瓙鎬ф搷浣滐紝瑕佷箞閮藉仛锛岃涔堜笉鍋?浣嗘槸锛屾垜璇曢獙浜嗕笅锛屽鏋滃湪public void checkout(String username, Listisbns) 涓婃柟涓嶅姞Transactional娉ㄨВ涓庡湪public void purchase(String username, String isbn)涓婃柟鍔燖Transactional(propagation=Propagation.REQUIRES_NEW鏁堟灉涓€鏍?
2銆佸綋璐︽埛浣欓涓?20锛屽彲浠ユ弧瓒寵處鎴峰噺鍘?001鐨勫崟浠鳳紝1001鐨勫簱瀛樺噺1 浣嗘槸锛屽皢璐︽埛浣欓鏀逛負80锛屼粠鍗曚環涓婄湅鍙互涔?002閭f湰涔︼紝鎸夌収涓婇潰鐨勯『搴忓幓涔頒功锛屾寜鐓т笂闈㈠姞涓婃敞瑙Transactional(propagation=Propagation.REQUIRES_NEW鏁堟灉涓€鏍鳳紝鏍規湰涓嶅ソ浣匡紝鎴戜釜浜鴻寰楀洜涓哄湪鏇存柊璐︽埛浣欓锛岄偅鏈変釜鍒ゆ柇鍏堟煡璇?001鐨勪功鐨勫崟浠風‘瀹炲ぇ浜庡綋鍓嶈處鎴蜂綑棰?0锛屽厛鍒ゆ柇浜嗭紝鎵€浠ユ姏寮傚父鍚庨潰浠g爜灏變笉璧頒簡
3銆佸彟澶栧綋鍓嶈處鎴蜂綑棰濆彲浠ヤ拱1002杩欐湰涔︼紝鎯沖湪涓嶆敼鍙樿喘涔︾殑椤哄簭鎯呭喌涓嬶紝鐢ˊTransactional娉ㄨВ瀹炵幇锛屽彲浠ヤ拱1002杩欐湰涔︼紵锛屽噺鍘誨綋鍓嶈處鎴蜂綑棰?0锛屾洿鏂?002涔︾殑搴撳瓨锛屽摢浣嶅ぇ绁炵湅鍒幫紝甯垜鐪嬩笅锛屾€庝箞鐢ㄨ繖涓敞瑙e疄鐜幫紵
浠ヤ笂涓烘垜鐨勪釜浜虹枒鎯戠殑鐐癸紝鏈夊叴瓒g殑鍚屽鍙互鐮旂┒涓嬶紝鐒跺悗鍦ㄥ叕鍙風暀瑷€缁欐垜鍗沖彲锛屽皬缂栦笉鑳滄劅璋紒
闄勪笂锛歴ql
/*
Navicat MySQL Data Transfer
Source Server : myTestdata
Source Server Version : 50627
Source Host : localhost:3306
Source Database : spring
Target Server Type : MYSQL
Target Server Version : 50627
File Encoding : 65001
Date: 2017-01-18 11:28:50
*/
SET FOREIGN_KEY_CHECKS=0;
-- ----------------------------
-- Table structure for account
-- ----------------------------
DROP TABLE IF EXISTS `account`;
CREATE TABLE `account` (
`username` varchar(50) NOT NULL,
`balance` int(11) DEFAULT NULL,
PRIMARY KEY (`username`)
) ENGINE=InnoDB DEFAULT CHARSET=gbk;
-- ----------------------------
-- Records of account
-- ----------------------------
INSERT INTO `account` VALUES ('rongrong', '300');
INSERT INTO `account` VALUES ('zhangsan', '200');
-- ----------------------------
-- Table structure for book
-- ----------------------------
DROP TABLE IF EXISTS `book`;
CREATE TABLE `book` (
`isbn` varchar(50) NOT NULL,
`book_name` varchar(100) DEFAULT NULL,
`price` int(11) DEFAULT NULL,
PRIMARY KEY (`isbn`)
) ENGINE=InnoDB DEFAULT CHARSET=gbk;
-- ----------------------------
-- Records of book
-- ----------------------------
INSERT INTO `book` VALUES ('1001', 'java', '100');
INSERT INTO `book` VALUES ('1002', 'python', '60');
-- ----------------------------
-- Table structure for book_stock
-- ----------------------------
DROP TABLE IF EXISTS `book_stock`;
CREATE TABLE `book_stock` (
`isbn` varchar(50) NOT NULL,
`bookname` varchar(100) DEFAULT NULL,
`stock` int(11) DEFAULT NULL,
PRIMARY KEY (`isbn`)
) ENGINE=InnoDB DEFAULT CHARSET=gbk;
-- ----------------------------
-- Records of book_stock
-- ----------------------------
INSERT INTO `book_stock` VALUES ('1001', 'java', '10');
INSERT INTO `book_stock` VALUES ('1002', 'python', '10');
澶嶅埗