一、isa指針結構
union isa_t
{
isa_t() { }
isa_t(uintptr_t value) : bits(value) { }
Class cls;
uintptr_t bits;
#if SUPPORT_PACKED_ISA
// extra_rc must be the MSB-most field (so it matches carry/overflow flags)
// nonpointer must be the LSB (fixme or get rid of it)
// shiftcls must occupy the same bits that a real class pointer would
// bits + RC_ONE is equivalent to extra_rc + 1
// RC_HALF is the high bit of extra_rc (i.e. half of its range)
// future expansion:
// uintptr_t fast_rr : 1; // no r/r overrides
// uintptr_t lock : 2; // lock for atomic property, @synch
// uintptr_t extraBytes : 1; // allocated with extra bytes
# if __arm64__
# define ISA_MASK 0x0000000ffffffff8ULL
# define ISA_MAGIC_MASK 0x000003f000000001ULL
# define ISA_MAGIC_VALUE 0x000001a000000001ULL
struct {
uintptr_t nonpointer : 1;
uintptr_t has_assoc : 1;
uintptr_t has_cxx_dtor : 1;
uintptr_t shiftcls : 33; // MACH_VM_MAX_ADDRESS 0x1000000000
uintptr_t magic : 6;
uintptr_t weakly_referenced : 1;
uintptr_t deallocating : 1;
uintptr_t has_sidetable_rc : 1;
uintptr_t extra_rc : 19;
# define RC_ONE (1ULL<<45)
# define RC_HALF (1ULL<<18)
};
# elif __x86_64__
# define ISA_MASK 0x00007ffffffffff8ULL
# define ISA_MAGIC_MASK 0x001f800000000001ULL
# define ISA_MAGIC_VALUE 0x001d800000000001ULL
struct {
uintptr_t nonpointer : 1;
uintptr_t has_assoc : 1;
uintptr_t has_cxx_dtor : 1;
uintptr_t shiftcls : 44; // MACH_VM_MAX_ADDRESS 0x7fffffe00000
uintptr_t magic : 6;
uintptr_t weakly_referenced : 1;
uintptr_t deallocating : 1;
uintptr_t has_sidetable_rc : 1;
uintptr_t extra_rc : 8;
# define RC_ONE (1ULL<<56)
# define RC_HALF (1ULL<<7)
};
# else
# error unknown architecture for packed isa
# endif
// SUPPORT_PACKED_ISA
#endif
#if SUPPORT_INDEXED_ISA
# if __ARM_ARCH_7K__ >= 2
# define ISA_INDEX_IS_NPI 1
# define ISA_INDEX_MASK 0x0001FFFC
# define ISA_INDEX_SHIFT 2
# define ISA_INDEX_BITS 15
# define ISA_INDEX_COUNT (1 << ISA_INDEX_BITS)
# define ISA_INDEX_MAGIC_MASK 0x001E0001
# define ISA_INDEX_MAGIC_VALUE 0x001C0001
struct {
uintptr_t nonpointer : 1;
uintptr_t has_assoc : 1;
uintptr_t indexcls : 15;
uintptr_t magic : 4;
uintptr_t has_cxx_dtor : 1;
uintptr_t weakly_referenced : 1;
uintptr_t deallocating : 1;
uintptr_t has_sidetable_rc : 1;
uintptr_t extra_rc : 7;
# define RC_ONE (1ULL<<25)
# define RC_HALF (1ULL<<6)
};
# else
# error unknown architecture for indexed isa
# endif
// SUPPORT_INDEXED_ISA
#endif
};
分析:
1.我們知道,執行個體對象的isa指針指向該對象所屬類的類對象;類對象的isa指向其元類對象;
2.真機為arm64架構,模拟器和mac電腦為x86架架構,以下以arm64為例講解;
3.在64位系統下,指針所占位元組為8個即64位;
4.在arm64之前,isa就是一個普通的指針,存放着類(元類)對象的位址;之後,則需要&
ISA_MASK掩碼,才能擷取到類(元類)對象的位址,此時isa指針為一個共用體,存儲的資訊不局限于類(元類)對象的位址;
5.存儲資訊介紹:
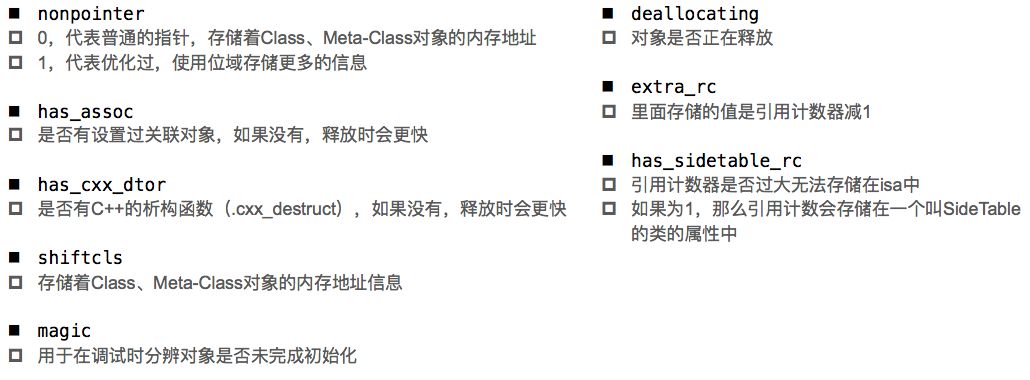
其中,shiftcls結構體成員變量(33位)用來存儲類(元類)對象的位址;
二、類(元類)對象的位址取值原理——位域
1.結構體支援位域運算
//代碼
struct bs {
unsigned a : 9;//如果超過位域範圍(511),則隻取範圍内的值,其他位(高位)丢棄
unsigned b : 4;
unsigned c : 3;
}bit, *pbit;
void test1()
{
bit.a = 512;//超過位域範圍報警告
bit.b = 10;
bit.c = 7;
NSLog(@"%d,%d,%d\n", bit.a, bit.b, bit.c);
pbit=&bit;
pbit-> a=0;
pbit-> b&=3;
pbit-> c|=1;
printf("%d,%d,%d\n ",pbit-> a,pbit-> b,pbit-> c);
}
//輸出
2019-10-08 18:22:37.051464+0800 SetAndGetsForMask[1966:248996] 0,10,7
0,2,7
Program ended with exit code: 0
//分析
1)unsigned即無符号整型,占4個位元組;結構體中成員變量所占記憶體互相獨立且連續;
2)以a為例,所占位數為9位即0b111111111(十進制511),是以a的取值範圍0~511,如果是512(二進制0b1000000000),由于隻取低9位(000000000),是以取出值為0;
3)按位與&:兩個都為1運算結果為1,否則為0;按位或|:兩個都為0運算結果為0,否則為1;
2.參照isa,共用體套用結構體,一個char字元(一個位元組)存儲多個BOOL值并制定存儲位置
2.設定類屬性BOOL值(setter and getter)
//Person
#import "Person.h"
//mask即掩碼,表示二進制數(0b開頭)
#define TallMask (1<<0) //表示1左移0位:0b 0000 0001
#define RichMask (1<<1) //表示1左移1位:0b 0000 0010
#define HandsomeMask (1<<2) //表示1左移2位:0b 0000 0100
//拓展:10<<3即在10對應的二進制數後添加3個0
@interface Person()
{
char _saveBox;
}
@end
@implementation Person
- (instancetype)init
{
if (self = [super init]) {
//用一個位元組來存儲三個變量:從最右往左依次為Tall、Rich、Handsome
_saveBox = 0b00000101;
}
return self;
}
/*思路
0000 0101(_saveBox)
|0000 0001(掩碼)
---------
0000 0001(指派tall為1)
0000 0101
&1111 1110(掩碼取反)
---------
0000 0100(指派tall為0)
1.如果賦的值為1,則按位或;
2.如果賦的值為0,則掩碼先取反,後按位與;
*/
- (void)setTall:(BOOL)tall
{
if (tall) {
_saveBox |= TallMask;
} else {
_saveBox &= ~TallMask;
}
}
- (void)setRich:(BOOL)rich
{
if (rich) {
_saveBox |= RichMask;
} else {
_saveBox &= ~RichMask;
}
}
- (void)setHandsome:(BOOL)handsome
{
if (handsome) {
_saveBox |= HandsomeMask;
} else {
_saveBox &= ~HandsomeMask;
}
}
/*思路
0000 0101
&0000 0001
---------
0000 0001(取出tall值)
1.按位與,用掩碼取出_saveBox中特定位;
2.結果>=1,取反為0,再取反為1;同理,為0則雙取反後為0;
*/
- (BOOL)isTall
{
return !!(_saveBox & TallMask);
}
- (BOOL)isRich
{
return !!(_saveBox & RichMask);
}
- (BOOL)isHandsome
{
return !!(_saveBox & HandsomeMask);
}
@end
//Student
#import "Student.h"
@interface Student()
{
/*思路
1.用一個結構體來存放變量;
2.結構體支援位域:按先後順序,一個char字元一個位元組(0b0000 0000),從最右至左依次為tall、rich、handsome;
*/
struct {
char tall : 1;//用一位來存儲
char rich : 1;
char handsome : 1;
}_tallRichHandsome;
}
@end
@implementation Student
- (void)setTall:(BOOL)tall
{
_tallRichHandsome.tall = tall;
}
- (void)setRich:(BOOL)rich
{
_tallRichHandsome.rich = rich;
}
- (void)setHandsome:(BOOL)handsome
{
_tallRichHandsome.handsome = handsome;
}
- (BOOL)isTall
{
return !!_tallRichHandsome.tall;//非0(包括負數)取反為0
}
- (BOOL)isRich
{
return !!_tallRichHandsome.rich;
}
- (BOOL)isHandsome
{
return !!_tallRichHandsome.handsome;
}
@end
//Worker
#import "Worker.h"
#define TallMask (1<<0)//也可以左移6位,剩餘位沒用到
#define RichMask (1<<1)
#define HandsomeMask (1<<2)
#define ThinMask (1<<3)
@interface Worker()
{
//蘋果系統設計思路
union {
char bits;//一個位元組存儲結構體中的所有成員變量
struct {//擺設用:位域,增加可讀性
char tall : 1;//占一位
char rich : 1;
char handsome : 1;
char thin : 1;
};
}_tallRichHandsome;
}
@end
@implementation Worker
- (void)setTall:(BOOL)tall
{
if (tall) {
NSLog(@"----%c", _tallRichHandsome.bits);
_tallRichHandsome.bits |= TallMask;
} else {
_tallRichHandsome.bits &= ~TallMask;
}
}
- (void)setRich:(BOOL)rich
{
if (rich) {
_tallRichHandsome.bits |= RichMask;
} else {
_tallRichHandsome.bits &= ~RichMask;
}
}
- (void)setHandsome:(BOOL)handsome
{
if (handsome) {
_tallRichHandsome.bits |= HandsomeMask;
} else {
_tallRichHandsome.bits &= ~HandsomeMask;
}
}
- (void)setThin:(BOOL)thin
{
if (thin) {
_tallRichHandsome.bits |= ThinMask;
} else {
_tallRichHandsome.bits &= ~ThinMask;
}
}
- (BOOL)isTall
{
return !!(_tallRichHandsome.bits & TallMask);
}
- (BOOL)isRich
{
return !!(_tallRichHandsome.bits & RichMask);
}
- (BOOL)isHandsome
{
return !!(_tallRichHandsome.bits & HandsomeMask);
}
- (BOOL)isThin
{
return !!(_tallRichHandsome.bits & ThinMask);
}
@end
//main
#import <Foundation/Foundation.h>
#import "Person.h"
#import "Student.h"
#import "Worker.h"
#import "Engineer.h"
struct bs {
unsigned a : 9;//如果超過位域範圍(511),則隻取範圍内的值,其他位(高位)丢棄
unsigned b : 4;
unsigned c : 3;
}bit, *pbit;
void test1()
{
bit.a = 512;//超過位域範圍報警告
bit.b = 10;
bit.c = 7;
NSLog(@"%d,%d,%d\n", bit.a, bit.b, bit.c);
pbit=&bit;
pbit-> a=0;
pbit-> b&=3;
pbit-> c|=1;
printf("%d,%d,%d\n ",pbit-> a,pbit-> b,pbit-> c);
}
void test2()
{
Person *per = [[Person alloc] init];
per.tall = NO;
per.rich = NO;
per.handsome = YES;
NSLog(@"%d %d %d", per.isTall, per.isRich, per.isHandsome);
}
void test3()
{
Student *stu = [[Student alloc] init];
stu.tall = YES;
stu.rich = NO;
stu.handsome = YES;
NSLog(@"%d %d %d", stu.isTall, stu.isRich, stu.isHandsome);
}
void test4()
{
Worker *worker = [[Worker alloc] init];
// worker.tall = YES;
worker.rich = NO;
worker.handsome = NO;
worker.thin = YES;
NSLog(@"%d %d %d", worker.isThin, worker.isRich, worker.isHandsome);
}
void test5()
{
Engineer *engineer = [[Engineer alloc] init];
// engineer.age = 12;
// engineer.level = 6;
// engineer.workers = 5;
//0b 1111 1111 1111 1111(十進制:65535)
//0b 0010 1100 1110 1101(十進制:11501)
engineer->_personalInfo.bits =11501;
NSLog(@"%d %d %d", engineer.getAge, engineer.getLevel, engineer.getWorkers);
//2019-10-08 16:42:09.612140+0800 SetAndGetsForMask[1488:127227] 7 16 8160
//
}
int main(int argc, const char * argv[]) {
@autoreleasepool {
test1();
// test2();
// test3();
// test4();
// test5();
}
return 0;
}
//列印
2019-10-09 10:42:04.998750+0800 SetAndGetsForMask[2513:316066] 0 0 1
2019-10-09 10:42:04.999093+0800 SetAndGetsForMask[2513:316066] 1 0 1
2019-10-09 10:42:04.999122+0800 SetAndGetsForMask[2513:316066] 1 0 0
Program ended with exit code: 0
//分析(以Worker為例)
1)共用體中所有成員共同占用一塊記憶體區,其大小等于最大那個成員所占位元組數;
2)Worker中的結構體并為定義變量,編譯器不會計算其記憶體,僅是增加可讀性;
3)Worker中隻有一個char型變量bits(占一個位元組),故該共用體變量_tallRichHandsome也占一個位元組;
4)結構體的位域限制變量的取值範圍(一位:即0或1),mask掩碼規定該變量存儲的位置(在哪一位上);
3.設定類屬性非BOOL類型(setter and getter)——限定變量值範圍且指定存儲位置
//Engineer
#import <Foundation/Foundation.h>
NS_ASSUME_NONNULL_BEGIN
//位域位置(變量值存儲位置)
#define AgeMask 0b00000111//最低三位存儲
#define LevelMask (1<<4)//低位往高位數,第5位存儲
#define WorkersMask 0b0001111111100000
@interface Engineer : NSObject
{
@public
union {
int bits;
struct {//位域範圍(變量值範圍)
int age : 3;
int level : 1;
int workers : 8;
};
}_personalInfo;
}
//- (void)setAge:(int)age;
//- (void)setLevel:(int)level;
//- (void)setWorkers:(int)workers;
- (int)getAge;
- (int)getLevel;
- (int)getWorkers;
@end
NS_ASSUME_NONNULL_END
#import "Engineer.h"
@implementation Engineer
//- (void)setAge:(int)age
//{
// self->_personalInfo.bits |= AgeMask;
//}
//
//- (void)setLevel:(int)level
//{
// self->_personalInfo.bits |= LevelMask;
//}
//
//- (void)setWorkers:(int)workers
//{
// self->_personalInfo.bits |= WorkersMask;
//}
- (int)getAge
{
return self->_personalInfo.bits & AgeMask;
}
- (int)getLevel
{
return self->_personalInfo.bits & LevelMask;
}
- (int)getWorkers
{
return self->_personalInfo.bits & WorkersMask;
}
@end
2019-10-09 11:08:14.617655+0800 SetAndGetsForMask[2630:349068] 5 0 3296
Program ended with exit code: 0
//說明
1)掩碼mask既可以直接用二進制(0b開頭)或十六進制(0x開頭)表示,也可以左移符号<<表示(一般用于位域為1的情況);
2)掩碼表示所占位數:1表示占住該位,0未占;并且所占位數應當是連續的,不存在兩側為1,中間為0的情況;
三、按位或(疊加)
typedef NS_OPTIONS(NSUInteger, UIViewAutoresizing) {
UIViewAutoresizingNone = 0,
UIViewAutoresizingFlexibleLeftMargin = 1 << 0,
UIViewAutoresizingFlexibleWidth = 1 << 1,
UIViewAutoresizingFlexibleRightMargin = 1 << 2,
UIViewAutoresizingFlexibleTopMargin = 1 << 3,
UIViewAutoresizingFlexibleHeight = 1 << 4,
UIViewAutoresizingFlexibleBottomMargin = 1 << 5
};
self.view.autoresizingMask = UIViewAutoresizingFlexibleWidth | UIViewAutoresizingFlexibleRightMargin;
1.分析:在iOS中,對view的自動布局經常會用到上述代碼,蘋果的做法是定義個枚舉且每種類型都隻占不同的一個二進制位,按位或相當于設定的場景都要考慮到;
2.如下代碼:
typedef enum{
YBOptionTypeNone = 0, //0b0000
YBOptionTypeOne = 1<<0, //0b0001
YBOptionTypeTwo = 1<<1, //0b0010
YBOptionTypeThree = 1<<2, //0b0100
YBOptionTypeFour = 1<<3 //0b1000
}YBOptionType;
void test6(YBOptionType option)
{
/*按位或疊加(包含多種情況),按位與取出(獲得特定情況)
0b0001
0b0100
| 0b1000
----------
0b1101
& 0b0100
----------
0b0100
*/
if (option & YBOptionTypeOne) {
NSLog(@"contain YBOptionTypeOne");
}
if (option & YBOptionTypeTwo) {
NSLog(@"contain YBOptionTypeTwo");
}
if (option & YBOptionTypeThree) {
NSLog(@"contain YBOptionTypeThree");
}
if (option & YBOptionTypeFour) {
NSLog(@"contain YBOptionTypeFour");
}
}
int main(int argc, const char * argv[]) {
@autoreleasepool {
// test1();
// test2();
// test3();
// test4();
// test5();
test6(YBOptionTypeOne | YBOptionTypeThree | YBOptionTypeFour);
}
return 0;
}
2019-10-22 21:55:17.154998+0800 SetAndGetsForMask[996:46994] contain YBOptionTypeOne
2019-10-22 21:55:17.155318+0800 SetAndGetsForMask[996:46994] contain YBOptionTypeThree
2019-10-22 21:55:17.155332+0800 SetAndGetsForMask[996:46994] contain YBOptionTypeFour
Program ended with exit code: 0
四、結論
1.arm64之後,isa是一個共用體類型的指針,存儲内部套用的結構體中的所有成員變量;
2.根據結構體的位域來限制成員變量的值範圍,用掩碼來規定成員變量存儲的位置,對掩碼按位與運算取出特定位置的成員變量的值;
如:用bits對ISA_MASK按位與運算後,得到的是類(元類)對象的位址;
可以看到shiftcls成員變量位域為33位,所占bits變量的存儲位置為:地位到高位第四位起,最低三位是空出來的
————是以,在arm64架構中,所有的類和元類對象位址二進制表示時最低三位都為0,十六進制表示時最低一位為0或8(這個用class和object_getClass去列印位址,此處不再展示了)!
3.按位與作用為在集合中取出某個特定值,按位或則将若幹個特定值集合到一個值中(即所有設定場景都要考慮到);
拓展:
//電腦示範
1.模拟器為x86_64架構,64位指針占八個位元組;在結構體中,自上而下的變量在isa中的存儲位置為從低位到高位即電腦中下到上、左到右——這點應該沒問題;
2.per執行個體對象曾經被弱指針指向過(現在釋放了),且曾經關聯過對象(現在置空),而對應的位為1即表示确實曾經被弱指針指向過(如果沒有則會為0),曾經關聯過對象;是以
has_assoc和weakly_referenced分别表示對象曾經被弱指針指向過和曾經關聯過對象——其他變量分析以此類推;
GitHub