net start mysql
#關閉:net stop mysql
#指令行進入資料庫:mysql -h 主機位址-u 使用者名 -p使用者密碼 ( -p後面的密碼要連着一起寫) mysql -u root -ppassword
#退出:exit:
#顯示目前mysql的version的各種資訊:status;
#顯示資料庫:show databases;
#判斷是否存在資料庫test,有的話先删除:drop database if exists test;
#建立資料庫:create database test;
#删除資料庫:drop database test;
#使用該資料庫:use test;
#顯示資料庫裡面的表(先進庫 在顯示表):show tables;
#先判斷表是否存在,存在先删除:drop table if exists student;
#建立表:create table student(
id int auto_increment primary key,
name varchar(50),
sex varchar(20),
date varchar(50),
content varchar(100)
)default charset=utf8;
#删除表:drop table student;
#檢視表的結構:describe student; 可以簡寫為desc student;
#插入資料:insert into student values(null,'aa','男','1994-05-14','yy');
insert into student values(null,'bb','女','1991-11-25','ll');
insert into student values(null,'cc','男','1992-10-5','tt');
insert into student values(null,'dd','男','1992-09-09','......');
insert into student values(null,'ee','女','1994-08-16','ll');
#查詢表中的資料:select * from student;
select id,name from student;
#修改某一條資料:update student set sex='男' where id=4;
#删除資料:delete from student where id=5;
# and 且:select * from student where date>'1949-10-1' and date<'2049-10-1';
# or 或:select * from student where date<'1992-10-5' or date>'1992-10-5';
#between:select * from student where date between'1992-2-3' and '1992-12-12';
#in查詢指定集合内的資料:select * from student where id in(1,3,5);
#排序文法 asc升序、desc 降序: 升序:select * from student order by id asc;
降序:select * from student order by id desc;
#分組查詢 #聚合函數:
select max(id),name,sex from student group by sex;
select min(date) from student;
select avg(id) as'求平均' from student;
select count(*) from student; #統計表中總數
select count(sex) from student; #統計表中性别總數 若有一條資料中sex為空的話,就不予以統計
select sum(id) from student;
#查詢第i條以後到第j條的資料(不包括第i條):select * from student limit 2,5;
#顯示3-5條資料
#檢視資料檔案路徑:mysql的資料檔案在datadir下,在資料庫中執行
show variables like '%datadir%';會顯示資料庫存放路徑
#鞏固練習: 建立表:create table person(
id int auto_increment primary key,
name varchar(10)not null,
sex varchar(50) ,
phone varchar(13),
account varchar(18),
password varchar(20)
create table c(
name varchar(10)notnull,
sex varchar(50) , #DEFAULT'男' ,
age int unsigned, #不能為負值(如為負值 則預設為0)
sno int unique #不可重複
);
drop table c;
desc c;
insert into c (id,name,sex,age,sno)values(null,'濤哥','男',68,1);
insert into c (id,name,sex,age,sno)values(null,'aa','男',68,2);
insert into c (id,name,sex,age,sno)values(null,'平平','男',35,3);
...
select * from c;
#修改資料update c setage=66 where id=2;update c setname='花花',age=21,sex='女'where id=2 delete from c where age=21;
#常用查詢語句:
select name,age ,id from c select * from c where age>40 and age<60; # and
select * from c where age<40 or age<60; #or
select * from c where age between 40 and 60 #between
select * from c where age in(30,48,68,99); #in
查詢指定集合内的資料select * from c order by age desc; #order by (asc升序 desc降序)
#分組查詢select name,max(age)from c groupby sex; #按性别分組查年齡最大值
#聚合函數selec tmin(age) from c;select avg(age) as'平均年齡 'from c;select count(*) from c; #統計表中資料總數select sum(age)from c;
#修改表的名字文法格式:alter table tbl_name rename to new_name
例如: alter table c rename to a
#表結構修改create table test
(
id int not null auto_increment primary key, #設定主鍵
name varchar(20)not null default'NoName', #設定預設值
department_id int not null,
position_id int not null,unique (department_id,position_id) #設定唯一值
#向表中增加一個字段(列) 文法格式:alter table tablename add column name type;/alter table tablename add(columnname type);
alter table test add column eat varchar(20); 這裡的 eat 代表的是新字段
#修改某個表的字段名稱及指定為空或非空:alter table 表名稱 change 字段原名稱 字段新名稱 字段類型 [是否允許非空];
#修改某個表的字段類型及指定為空或非空:alter table 表名稱 change 字段名稱 字段名稱 字段類型 [是否允許非空];
alter table 表名稱 modify 字段名稱 字段類型 [是否允許非空];
#student表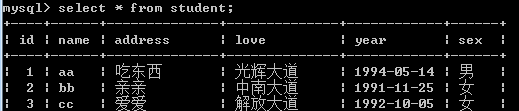
student
#coder表coder表
為了看到多表關聯的效果,我将student表的 year 字段改成 date 字段: alert table student change year date date;表字段修改完畢以後然後插入資料:
insert into student values(null,'dd','嘿嘿咻咻','中山大道','1992-09-09','男');
insert into student values(null,'dd','雅蠛蝶','方圓E時光','1988-10-2','女');
insert into student values(null,'dd','用力呀寶寶','越秀公園','1989-03-6','男');
insert into student values(null,'dd','用力呀寶寶','越秀公園','1989-03-06','男');
最終的student表為:
操作符用于連接配接兩個以上的 SELECT 語句的結果組合到一個結果集合中。(預設情況下 UNION 操作符已經删除了重複資料)
下面的 SQL 語句從 "coder" 和 "student" 表中選取所有不同的date(隻有不同的值):按照升序對結果進行統一排序:
select date from student union select date from coder order by date;下面的 SQL 語句從 "coder" 和 "student" 表中選取所有的date(含重複值 使用
union all):
select date from student union all select date from coder order by date;INNER JOIN(内連接配接,或等值連接配接):擷取兩個表中字段比對關系的記錄。
LEFT JOIN(左連接配接):擷取左表所有記錄,即使右表沒有對應比對的記錄。
RIGHT JOIN(右連接配接): 與 LEFT JOIN 相反,用于擷取右表所有記錄,即使左表沒有對應比對的記錄。
現在有兩張表 一個是 coder表 ;一個是student表;兩張表資料如下:
select a.id, a.name, b.date FROM coder a JOIN student b ON a.name = b.name;
這裡的a 代表的就是 coder表:這裡的b 代表的就是 student表,結果如下:
Join
其中LEFT JOIN 以及RIGHT JOIN 關鍵字,上面也說到了,分别擷取左、右兩邊所有記錄:
SELECT a.id, a.name, b.date FROM coder a LEFT JOIN student b ON a.name = b.name;
LEFT JOIN
SELECT a.id, a.name, b.date FROM coder a RIGHT JOIN student b ON a.name = b.name;
RIGHT JOIN
NULL 值處理MySQL 使用 SQL SELECT 指令及 WHERE 子句來讀取資料表中的資料,當提供的查詢條件字段為 NULL 時,該指令可能就無法正常工作。
是以為了處理這種情況,MySQL提供了三大運算符:
IS NULL: 當列的值是 NULL,此運算符傳回 true。
IS NOT NULL: 當列的值不為 NULL, 運算符傳回 true。
<=>: 比較操作符(不同于=運算符),當比較的的兩個值為 NULL 時傳回 true。
關于 NULL 的條件比較運算是比較特殊的。我們
不能直接使用 = NULL 或 != NULL 這種Java的文法在表中查找 NULL 值 。在 MySQL 中,NULL 值與任何其它值的比較(即使是 NULL)永遠傳回 false,即 NULL = NULL 傳回false 。
是以MySQL 中處理 NULL 的
正确使用姿勢是 使用IS NULL 和 IS NOT NULL 運算符。
NULL運算符
正規表達式:MySQL中使用 REGEXP 操作符來進行正規表達式比對。假定現有login表 正規表達式MYSQL文法格式如下:
login
查找name字段中以'小'為開頭的所有資料(使用 ^ 這個符号):
SELECT name FROM login WHERE name REGEXP '^小';
查找name字段中以'王'為結尾的所有資料(使用 $ 這個符号):
SELECT name FROM login WHERE name REGEXP '王$';
查找date字段中包含'小明'字元串的所有資料:
SELECT name FROM login WHERE name REGEXP '小明';
select語句
#用文本方式将資料裝入資料庫表中(例如D:/mysql.txt)loaddata local infile "D:/mysql.txt"intotable MYTABLE;
#導入.sql檔案指令(例如D:/mysql.sql)
source d:/mysql.sql; #或者/. d:/mysql.sql;
如果這篇文章對你有幫助,希望各位看官留下寶貴的star,謝謝。
Ps:著作權歸作者所有,轉載請注明作者, 商業轉載請聯系作者獲得授權,非商業轉載請注明出處(開頭或結尾請添加轉載出處,添加原文url位址),文章請勿濫用,也希望大家尊重筆者的勞動成果。