package com.web.utils;
import java.io.File;
import java.util.List;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import jxl.Workbook;
import jxl.format.UnderlineStyle;
import jxl.write.Label;
import jxl.write.WritableCellFormat;
import jxl.write.WritableFont;
import jxl.write.WritableSheet;
import jxl.write.WritableWorkbook;
public class XLSUtils {
private static Log logger = LogFactory.getLog(XLSUtils.class);
public static File createXLSFile(String title, String[] columnName, List<String[]> content)
{
File tmpFile = null;
try
{
tmpFile = File.createTempFile(title, ".xls");
WritableWorkbook book = Workbook.createWorkbook(tmpFile);
// 建立工作表
WritableSheet sheet = book.createSheet("Sheet1", 0);
// 建立标題
if(columnName != null)
{
WritableFont wf_merge = new WritableFont(WritableFont.ARIAL,20,WritableFont.NO_BOLD,
false,UnderlineStyle.NO_UNDERLINE,jxl.format.Colour.BLACK);
WritableCellFormat wff_merge = new WritableCellFormat(wf_merge);
// 水準對齊
wff_merge.setAlignment(jxl.format.Alignment.CENTRE);
// 垂直對齊
wff_merge.setVerticalAlignment(jxl.format.VerticalAlignment.CENTRE);
// 建立表頭
for(int i = 0; i < columnName.length; i ++)
{
sheet.addCell(new Label(i, 0, columnName[i]));
}
}
// 建立内容
if(content != null)
{
for(int i = 0; i < content.size(); i ++)
{
String s[] = content.get(i);
for(int j = 0; j < s.length; j ++)
{
sheet.addCell(new Label(j, i + 1, s[j]));
}
}
}
book.write();
book.close();
}
catch (Exception e)
{
logger.info("建立xls異常? create xls exeception", e);
return null;
}
return tmpFile;
}
}
package com.web.utils;
import java.io.File;
import java.util.List;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import jxl.Workbook;
import jxl.format.UnderlineStyle;
import jxl.write.Label;
import jxl.write.WritableCellFormat;
import jxl.write.WritableFont;
import jxl.write.WritableSheet;
import jxl.write.WritableWorkbook;
public class XLSUtils {
private static Log logger = LogFactory.getLog(XLSUtils.class);
public static File createXLSFile(String title, String[] columnName, List<String[]> content)
{
File tmpFile = null;
try
{
tmpFile = File.createTempFile(title, ".xls");
WritableWorkbook book = Workbook.createWorkbook(tmpFile);
// 建立工作表
WritableSheet sheet = book.createSheet("Sheet1", 0);
// 建立标題
if(columnName != null)
{
WritableFont wf_merge = new WritableFont(WritableFont.ARIAL,20,WritableFont.NO_BOLD,
false,UnderlineStyle.NO_UNDERLINE,jxl.format.Colour.BLACK);
WritableCellFormat wff_merge = new WritableCellFormat(wf_merge);
// 水準對齊
wff_merge.setAlignment(jxl.format.Alignment.CENTRE);
// 垂直對齊
wff_merge.setVerticalAlignment(jxl.format.VerticalAlignment.CENTRE);
// 建立表頭
for(int i = 0; i < columnName.length; i ++)
{
sheet.addCell(new Label(i, 0, columnName[i]));
}
}
// 建立内容
if(content != null)
{
for(int i = 0; i < content.size(); i ++)
{
String s[] = content.get(i);
for(int j = 0; j < s.length; j ++)
{
sheet.addCell(new Label(j, i + 1, s[j]));
}
}
}
book.write();
book.close();
}
catch (Exception e)
{
logger.info("建立xls異常? create xls exeception", e);
return null;
}
return tmpFile;
}
}
package com.web.utils;
import java.io.File;
import java.util.List;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
public class DownLoadExcel {
public static String downLoadExcel(String title, String[] columnName, List<String[]> content, HttpServletRequest request, HttpServletResponse response)
{
try{
File file = XLSUtils.createXLSFile(title, columnName, content);
if(file == null)
{
return null;
}
FileServerUtil.downFile(file, request, response);
}
catch (Exception e)
{
e.printStackTrace();
}
return null;
}
}
捐助開發者
在興趣的驅動下,寫一個
免費
的東西,有欣喜,也還有汗水,希望你喜歡我的作品,同時也能支援一下。 當然,有錢捧個錢場(右上角的愛心标志,支援支付寶和PayPal捐助),沒錢捧個人場,謝謝各位。
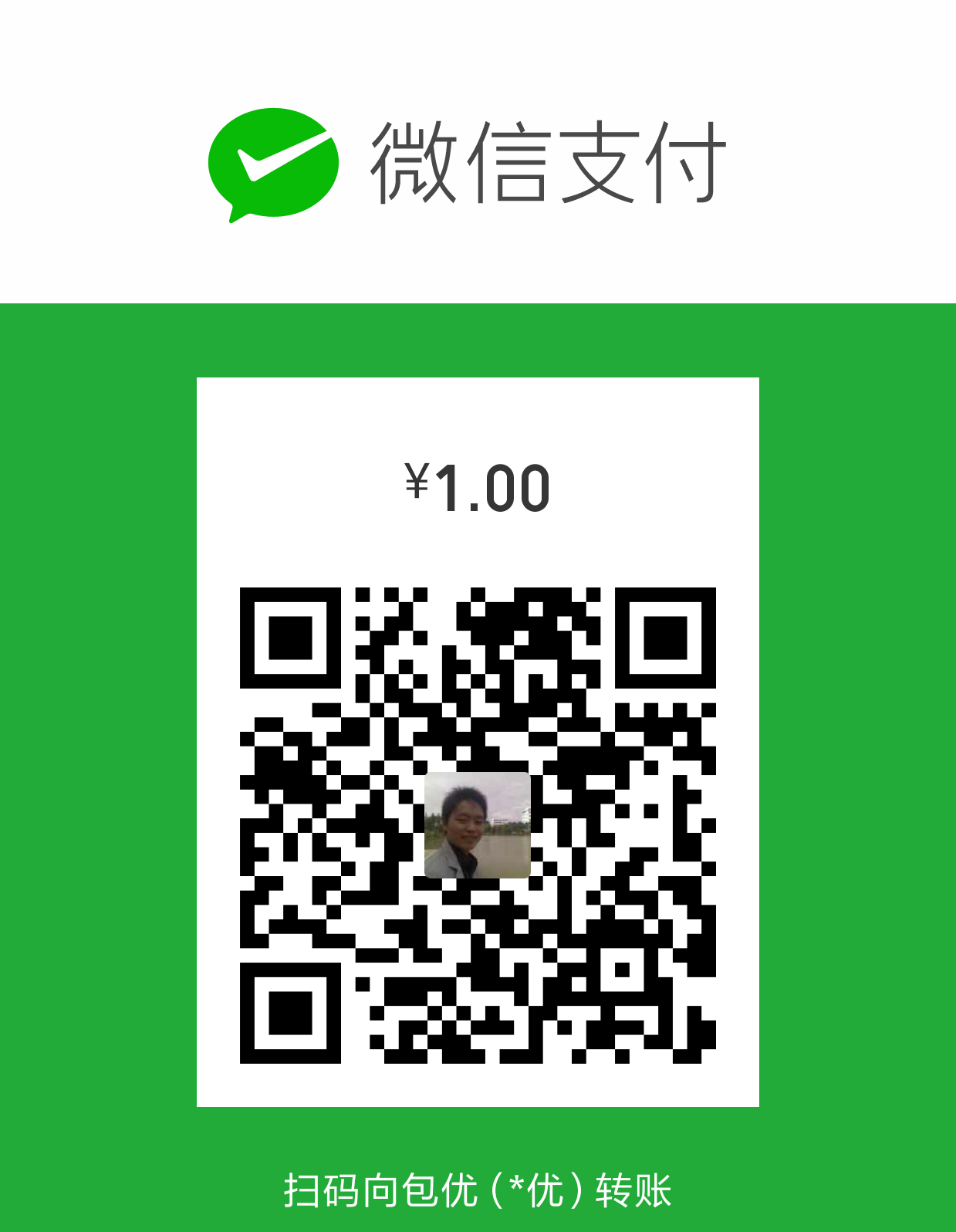
謝謝您的贊助,我會做的更好!