While analyzing source code of a large number of open source Java projects, I found Java developers frequently sort in two ways. One is using the sort() Collections Arrays TreeMap TreeSet
method of
or
, and the other is using sorted data structures, such as
and
.
1. Using sort() Method
If it is a collection, use Collections.sort() method.
|
If it is an array, use
Arrays.sort()
method.
|
This is very convenient if a collection or an array is already set up.
2. Using Sorted Data Structures
If it is a list or set, use
TreeSet
to sort.
|
If it is a map, use
TreeMap
to sort.
TreeMap
is sorted by key.
|
|
This approach is very useful, if you would do a lot of search operations for the collection. The sorted data structure will give time complexity of
O(logn)
, which is lower than
O(n)
3. Bad Practices
There are still bad practices, such as using self-defined sorting algorithm. Take the code below for example, not only the algorithm is not efficient, but also it is not readable. This happens a lot in different forms of variations.
|
捐助開發者
在興趣的驅動下,寫一個
免費
的東西,有欣喜,也還有汗水,希望你喜歡我的作品,同時也能支援一下。 當然,有錢捧個錢場(支援支付寶和微信 以及扣扣群),沒錢捧個人場,謝謝各位。
個人首頁:
http://knight-black-bob.iteye.com/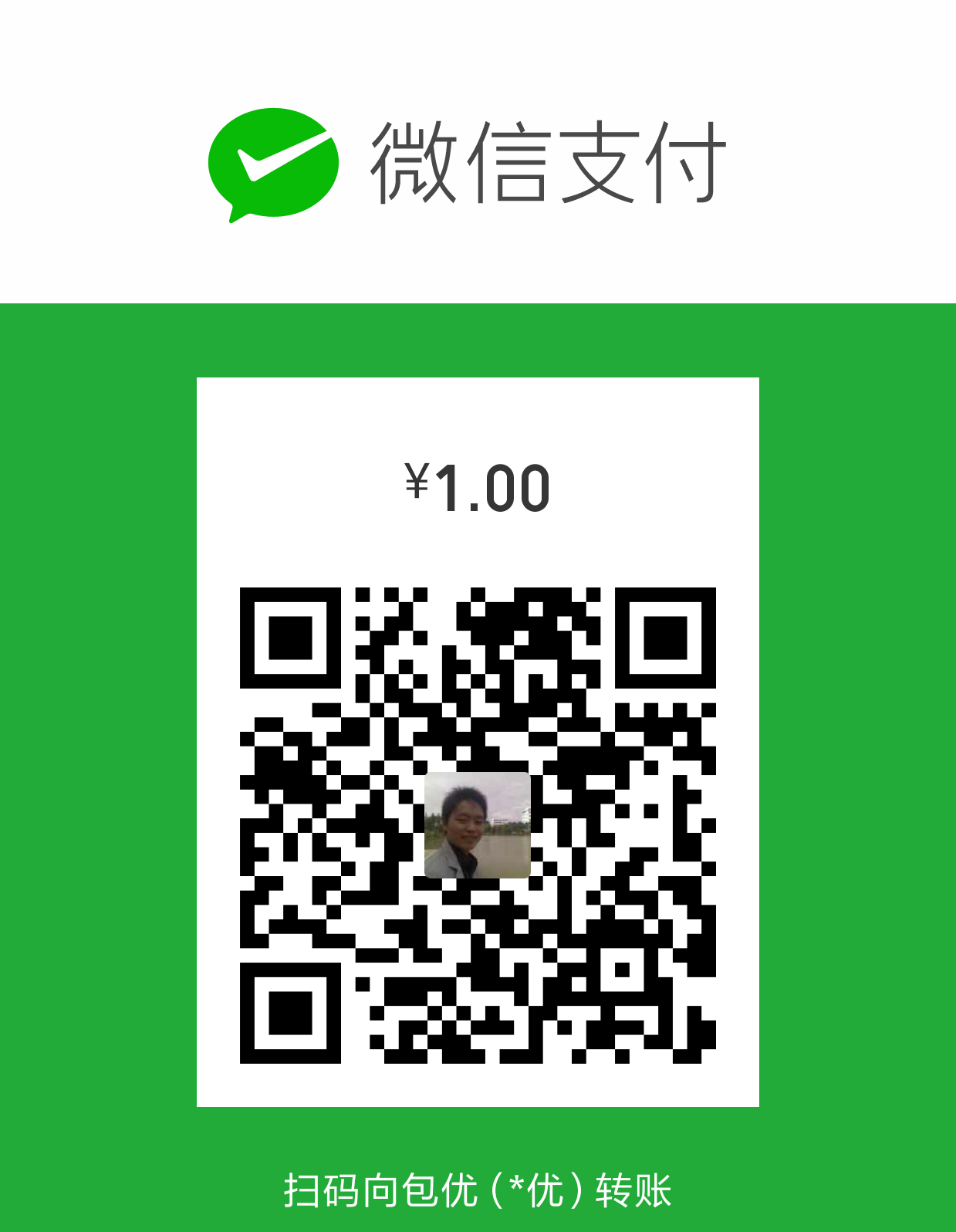
謝謝您的贊助,我會做的更好!