《大話設計模式》閱讀筆記和總結。原書是C#編寫的,本人用Java實作了一遍,包括每種設計模式的UML圖實作和示例代碼實作。
目錄:
設計模式 Github位址: DesignPattern
說明
定義:橋接模式(Bridge),将抽象部分與它的實作部分分離,使它們都可以獨立地變化。
UML圖:
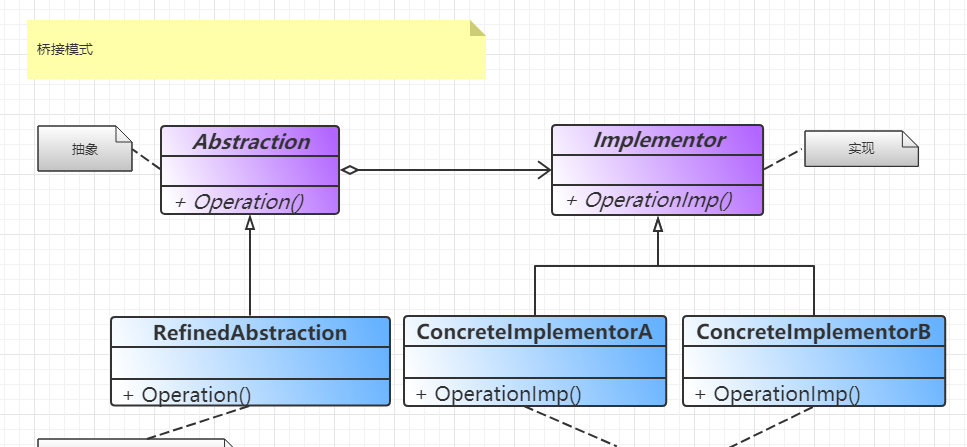
橋接模式UML圖.png
代碼實作:
Implementor類
abstract class Implementor{
public abstract void Operation();
}
ConcreteImplementorA和ConcreteImplementorB等派生類
class ConcreteImplementorA extends Implementor{
@Override
public void Operation() {
System.out.println("具體實作A的方法執行");
}
}
class ConcreteImplementorB extends Implementor{
@Override
public void Operation() {
System.out.println("具體實作B的方法執行");
}
}
Abstraction類
class Abstraction{
protected Implementor implementor;
public void setImplementor(Implementor implementor) {
this.implementor = implementor;
}
public void Operation(){
implementor.Operation();
}
}
RefinedAbstraction類
class RefinedAbstraction extends Abstraction{
@Override
public void Operation() {
implementor.Operation();
}
}
用戶端代碼
public class BridgePattern {
public static void main(String[] args){
Abstraction ab = new RefinedAbstraction();
ab.setImplementor(new ConcreteImplementorA());
ab.Operation();
ab.setImplementor(new ConcreteImplementorB());
ab.Operation();
}
}
運作結果
具體實作A的方法執行
具體實作B的方法執行
示例
例子:我們原來用的手機都是分為不同的品牌,每種手機裡面都内置了很多小遊戲,同時也有很多軟體,但是不同手機之間的遊戲是不能互相移植的。用程式模拟這個情景。
橋接模式示例UML圖.png
手機品牌類
public abstract class HandsetBrand {
protected HandsetSoft soft;
public void setSoft(HandsetSoft soft) {
this.soft = soft;
}
//運作
public abstract void Run();
}
手機品牌M
public class HandsetBrandM extends HandsetBrand {
@Override
public void Run() {
soft.Run();
}
}
手機品牌N
public class HandsetBrandN extends HandsetBrand {
@Override
public void Run() {
soft.Run();
}
}
手機軟體
public abstract class HandsetSoft {
public abstract void Run();
}
遊戲、通訊錄等具體類
手機遊戲
public class HandsetGame extends HandsetSoft {
@Override
public void Run() {
System.out.println("運作手機遊戲");
}
}
手機通訊錄
public class HandsetAddressList extends HandsetSoft {
@Override
public void Run() {
System.out.println("運作手機通訊錄");
}
}
public class Main {
public static void main(String[] args){
HandsetBrand ab;
ab = new HandsetBrandN();
ab.setSoft(new HandsetGame());
ab.Run();
ab.setSoft(new HandsetAddressList());
ab.Run();
ab = new HandsetBrandM();
ab.setSoft(new HandsetGame());
ab.Run();
ab.setSoft(new HandsetAddressList());
ab.Run();
}
}
運作手機遊戲
運作手機通訊錄
運作手機遊戲
運作手機通訊錄