南邮操作系统实验1
实验1:
运行以下程序,判断该程序创建了多少进程?
#include <stdio.h>
#include <unistd.h>
main()
{
pid_t pid1,pid2;
pid1=fork();
pid2=fork();
printf("pid = %d\n", getpid());
sleep(20); //为了观察
}
运行结果截图
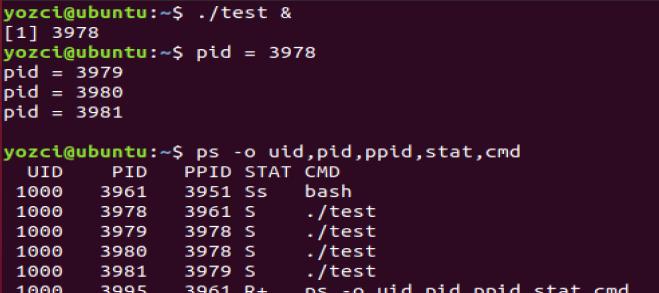
实验2:
1、父进程创建管道 2、父进程向管道写入Greetings 3、子进程从管道中读取消息,并显示在屏幕上
运行结果截图
实验3:
消息缓冲队列:
编写程序,利用消息缓冲队列实现client与server之间的通信
server.c
#include <stdlib.h>
#include <stdio.h>
#include <unistd.h>
#include <string.h>
#include <sys/types.h>
#include <sys/msg.h>
#include <sys/ipc.h>
#define MAXMSZ 250
#define MSGKEY 75
struct mymesg{
long mtype;
char mtext[MAXMSZ];
};
int main()
{
struct mymesg buf;
int msqid = msgget( MSGKEY,0777|IPC_CREAT);
int nbytes = sizeof(struct mymesg)-sizeof(int);
memset(&buf,0x00,sizeof(struct mymesg));
int mtype = 1;
int flag = msgrcv( msqid,&buf,nbytes,mtype,0);
buf.mtype = atol(buf.mtext);
printf("serving for client.server_pid = %d\n type=%d,message=%d\n",getpid(),mtype,msqid);
sprintf(buf.mtext,"%d",getpid());
nbytes = strlen(buf.mtext);
msgsnd(msqid,&buf,nbytes,0);
printf("server send message:type=%d text=%s,msglen=%d",(int)buf.mtype,buf.mtext,nbytes);
sleep(10);
msgctl(msqid,IPC_RMID,0);
}
client.c
#include <stdlib.h>
#include <stdio.h>
#include <unistd.h>
#include <string.h>
#include <sys/types.h>
#include <sys/msg.h>
#include <sys/ipc.h>
#define MAXMSZ 250
#define MSGKEY 75
struct mymesg{
long mtype;
char mtext[MAXMSZ];
};
int main()
{
int qid,nbytes;
struct mymesg m;
qid = msgget(MSGKEY,0);
memset(&m,0,sizeof(m));
nbytes = strlen(m.mtext);
m.mtype =1;
msgsnd(qid,&m,nbytes,0);
printf("client (pid=%d) send message: type = %ld,text = %d",qid,m.mtype,qid);
nbytes = sizeof(struct mymesg)-sizeof(int);
memset(&m,0x00,sizeof(struct mymesg));
int mtype =getpid();
int flag =msgrcv(qid,&m,nbytes,mtype,0);
printf("receive reply from server.type = %d,message = %s",(int)m.mtype,m.mtext);
}
运行结果截图
实验4:
共享存储区
用fork()创建子进程,利用共享存储区实现client和server的通信
#include<sys/types.h>
#include<sys/msg.h>
#include<sys/ipc.h>
#define SHMKEY 75
void CLIENT()
{
int shmid,i;
int *addr;
shmid=shmget(SHMKEY,1024, 0777|IPC_CREAT);
addr=shmat(shmid,0,0);
for(i=9;i>=0;i--)
{while(*addr!= -1);
printf("(client)sent\n");
*addr=i;
}
exit(0);
}
void SERVER()
{
int shmid;
int *addr;
shmid=shmget(SHMKEY,1024,0777|IPC_CREAT);
addr=shmat(shmid,0,0);
*addr=-1;
do
{
while(*addr ==-1);
printf("(server)received\n%d",*addr);
if(*addr!=0)
*addr=-1;
}while(*addr!=0);
wait(0);
shmctl(shmid,IPC_RMID,0);
}
void main()
{
if(fork())
{
SERVER();
}else
{CLIENT();
}
}
运行结果截图