import java.text.DecimalFormat;
public class Calculate {
// 形参为本金,利率,存入年限
// F:复利终值
// P:本金
// I:利率
// N:存入年限
// C: 年复利次数
double principal;//本金
double yearRate;//年利率
int years;//存入年限
int bonusTime;//年复利次数
double compoundInterest;//复利终值
double simpleInterest;
double i;
public Calculate(String P, String I, String N, String C, String F) {
if(P == null || P.length() <= 0){
principal = 0;
}else {
principal = Double.parseDouble(P);
}
if(I == null || I.length() <= 0){
yearRate = 0;
}else {
yearRate = Double.parseDouble(I);
}
if(N == null || N.length() <= 0){
years = 0;
}else {
years = Integer.parseInt(N);
}
if(C == null || C.length() <= 0){
bonusTime = 0;
}else {
bonusTime = Integer.parseInt(C);
}
if(F == null || F.length() <= 0){
compoundInterest = 0;
}else {
compoundInterest = Double.parseDouble(F);
}
i = Math.pow(1.0 + yearRate / bonusTime, bonusTime) - 1;
}
//求复利终值
public String calculateCompoundInterest(){
DecimalFormat df = new DecimalFormat("#.0000");
compoundInterest = principal * Math.pow((1 + i), years);
return df.format(compoundInterest);
}
//求本金
public String calculatePrincipal() {
DecimalFormat df = new DecimalFormat("#.0000");
principal = compoundInterest / Math.pow((1 + i), years);
return df.format(principal);
}
//求时间
public String calculateYears() {
if((Math.log(compoundInterest / principal) / Math.log(1 + i)) % 1 > 0){
years = (int) (Math.log(compoundInterest / principal) / Math.log(1 + i)) + 1;
}
return String.valueOf(years);
}
//求单利
public String calculatSimpleInterest() {
simpleInterest = principal * yearRate * years + principal;
return String.valueOf(simpleInterest);
}
}
1 import java.awt.Font;
2 import java.awt.event.MouseAdapter;
3 import java.awt.event.MouseEvent;
4
5 import javax.swing.JButton;
6 import javax.swing.JFrame;
7 import javax.swing.JLabel;
8 import javax.swing.JRadioButton;
9 import javax.swing.JTextField;
10 import javax.swing.SwingUtilities;
11 import javax.swing.UIManager;
12
13 import org.dyno.visual.swing.layouts.Constraints;
14 import org.dyno.visual.swing.layouts.GroupLayout;
15 import org.dyno.visual.swing.layouts.Leading;
16
17 //VS4E -- DO NOT REMOVE THIS LINE!
18 public class MainFrame extends JFrame {
19
20 private static final long serialVersionUID = 1L;
21 private JLabel jLabel0;
22 private JLabel jLabel1;
23 private JLabel jLabel2;
24 private JLabel jLabel3;
25 public MainFrame() {
26 initComponents();
27 }
28
29 private void initComponents() {
30 setLayout(new GroupLayout());
31 add(getJLabel0(), new Constraints(new Leading(60, 10, 10), new Leading(70, 10, 10)));
32 add(getJLabel1(), new Constraints(new Leading(60, 10, 10), new Leading(170, 10, 10)));
33 add(getJLabel2(), new Constraints(new Leading(60, 10, 10), new Leading(270, 10, 10)));
34 add(getJLabel3(), new Constraints(new Leading(60, 10, 10), new Leading(370, 10, 10)));
35 add(getJLabel4(), new Constraints(new Leading(60, 10, 10), new Leading(470, 10, 10)));
36 add(getJTextField0(), new Constraints(new Leading(290, 300, 10, 10), new Leading(70, 50, 10, 10)));
37 add(getJTextField1(), new Constraints(new Leading(290, 300, 10, 10), new Leading(170, 50, 10, 10)));
38 add(getJTextField2(), new Constraints(new Leading(290, 300, 10, 10), new Leading(270, 50, 10, 10)));
39 add(getJTextField3(), new Constraints(new Leading(290, 300, 10, 10), new Leading(370, 50, 10, 10)));
40 add(getJTextField4(), new Constraints(new Leading(290, 300, 10, 10), new Leading(470, 50, 10, 10)));
41 add(getJRadioButton0(), new Constraints(new Leading(630, 10, 10), new Leading(70, 10, 10)));
42 add(getJRadioButton1(), new Constraints(new Leading(630, 10, 10), new Leading(170, 120, 10)));
43 add(getJRadioButton2(), new Constraints(new Leading(630, 10, 10), new Leading(270, 10, 10)));
44 add(getJRadioButton3(), new Constraints(new Leading(630, 10, 10), new Leading(370, 10, 10)));
45 add(getJButton0(), new Constraints(new Leading(650, 10, 10), new Leading(470, 10, 10)));
46 setSize(800, 600);
47 }
48
49 private JRadioButton getJRadioButton3() {
50 if (jRadioButton3 == null) {
51 jRadioButton3 = new JRadioButton();
52 jRadioButton3.setFont(new Font("宋体", Font.BOLD, 40));
53 jRadioButton3.setSelected(false);
54 jRadioButton3.setText("求单利");
55 jRadioButton3.addMouseListener(new MouseAdapter() {
56
57 public void mouseClicked(MouseEvent event) {
58 jRadioButton3MouseMouseClicked(event);
59 }
60 });
61 }
62 return jRadioButton3;
63 }
64
65 private JRadioButton getJRadioButton2() {
66 if (jRadioButton2 == null) {
67 jRadioButton2 = new JRadioButton();
68 jRadioButton2.setFont(new Font("宋体", Font.BOLD, 40));
69 jRadioButton2.setSelected(false);
70 jRadioButton2.setText("求时间");
71 jRadioButton2.addMouseListener(new MouseAdapter() {
72
73 public void mouseClicked(MouseEvent event) {
74 jRadioButton2MouseMouseClicked(event);
75 }
76 });
77 }
78 return jRadioButton2;
79 }
80
81 private JTextField getJTextField4() {
82 if (jTextField4 == null) {
83 jTextField4 = new JTextField();
84 jTextField4.setFont(font);
85 jTextField4.setEditable(false);
86 }
87 return jTextField4;
88 }
89
90 private JLabel getJLabel4() {
91 if (jLabel4 == null) {
92 jLabel4 = new JLabel();
93 jLabel4.setFont(new Font("宋体", Font.BOLD, 40));
94 jLabel4.setText("复利终值:");
95 }
96 return jLabel4;
97 }
98
99 private JRadioButton getJRadioButton1() {
100 if (jRadioButton1 == null) {
101 jRadioButton1 = new JRadioButton();
102 jRadioButton1.setFont(new Font("宋体", Font.BOLD, 40));
103 jRadioButton1.setSelected(true);
104 jRadioButton1.setText("求复利");
105 jRadioButton1.addMouseListener(new MouseAdapter() {
106
107 public void mouseClicked(MouseEvent event) {
108 jRadioButton1MouseMouseClicked(event);
109 }
110 });
111 }
112 return jRadioButton1;
113 }
114
115 private JRadioButton getJRadioButton0() {
116 if (jRadioButton0 == null) {
117 jRadioButton0 = new JRadioButton();
118 jRadioButton0.setFont(new Font("宋体", Font.BOLD, 40));
119 jRadioButton0.setSelected(false);
120 jRadioButton0.setText("求本金");
121 jRadioButton0.addMouseListener(new MouseAdapter() {
122
123 public void mouseClicked(MouseEvent event) {
124 jRadioButton0MouseMouseClicked(event);
125 }
126 });
127 }
128 return jRadioButton0;
129 }
130
131 private JButton getJButton0() {
132 if (jButton0 == null) {
133 jButton0 = new JButton();
134 jButton0.setFont(new Font("宋体", Font.BOLD, 40));
135 jButton0.setText("计算");
136 jButton0.addMouseListener(new MouseAdapter() {
137
138 public void mouseClicked(MouseEvent event) {
139 jButton0MouseMouseClicked(event);
140 }
141 });
142 }
143 return jButton0;
144 }
145
146 private JTextField getJTextField3() {
147 if (jTextField3 == null) {
148 jTextField3 = new JTextField();
149 jTextField3.setFont(font);
150 }
151 return jTextField3;
152 }
153
154 private JTextField getJTextField2() {
155 if (jTextField2 == null) {
156 jTextField2 = new JTextField();
157 jTextField2.setFont(font);
158 }
159 return jTextField2;
160 }
161
162 private JTextField getJTextField1() {
163 if (jTextField1 == null) {
164 jTextField1 = new JTextField();
165 jTextField1.setFont(font);
166 }
167 return jTextField1;
168 }
169
170 private JTextField getJTextField0() {
171 if (jTextField0 == null) {
172 jTextField0 = new JTextField();
173 jTextField0.setFont(font);
174 }
175 return jTextField0;
176 }
177
178 Font font = new Font("宋体",Font.BOLD,40);
179 private JTextField jTextField0;
180 private JTextField jTextField1;
181 private JTextField jTextField2;
182 private JTextField jTextField3;
183 private JButton jButton0;
184 private JRadioButton jRadioButton0;
185 private JRadioButton jRadioButton1;
186 private JLabel jLabel4;
187 private JTextField jTextField4;
188 private JRadioButton jRadioButton2;
189 private JRadioButton jRadioButton3;
190 private static final String PREFERRED_LOOK_AND_FEEL = "javax.swing.plaf.metal.MetalLookAndFeel";
191 private JLabel getJLabel3() {
192 if (jLabel3 == null) {
193 jLabel3 = new JLabel();
194 jLabel3.setFont(font);
195 jLabel3.setText("年复利次数:");
196 }
197 return jLabel3;
198 }
199
200 private JLabel getJLabel2() {
201 if (jLabel2 == null) {
202 jLabel2 = new JLabel();
203 jLabel2.setFont(font);
204 jLabel2.setText("存入年限:");
205 }
206 return jLabel2;
207 }
208
209 private JLabel getJLabel1() {
210 if (jLabel1 == null) {
211 jLabel1 = new JLabel();
212 jLabel1.setFont(new Font("宋体", Font.BOLD, 40));
213 jLabel1.setText("年利率比:");
214 }
215 return jLabel1;
216 }
217
218 private JLabel getJLabel0() {
219 if (jLabel0 == null) {
220 jLabel0 = new JLabel();
221 jLabel0.setFont(font);
222 jLabel0.setText("存入本金:");
223 }
224 return jLabel0;
225 }
226
227 private static void installLnF() {
228 try {
229 String lnfClassname = PREFERRED_LOOK_AND_FEEL;
230 if (lnfClassname == null)
231 lnfClassname = UIManager.getCrossPlatformLookAndFeelClassName();
232 UIManager.setLookAndFeel(lnfClassname);
233 } catch (Exception e) {
234 System.err.println("Cannot install " + PREFERRED_LOOK_AND_FEEL + " on this platform:" + e.getMessage());
235 }
236 }
237
238 /**
239 * Main entry of the class.
240 * Note: This class is only created so that you can easily preview the result at runtime.
241 * It is not expected to be managed by the designer.
242 * You can modify it as you like.
243 */
244 public static void main(String[] args) {
245 installLnF();
246 SwingUtilities.invokeLater(new Runnable() {
247 public void run() {
248 MainFrame frame = new MainFrame();
249 frame.setDefaultCloseOperation(MainFrame.EXIT_ON_CLOSE);
250 frame.setTitle("复利计算器 V3.0");
251 frame.getContentPane().setPreferredSize(frame.getSize());
252 frame.pack();
253 frame.setLocationRelativeTo(null);
254 frame.setVisible(true);
255 }
256 });
257
258 }
259
260 private void jButton0MouseMouseClicked(MouseEvent event) {
261
262 // if (jTextField0.getText() == null || jTextField0.getText().length() <= 0) {
263 // JOptionPane.showMessageDialog( null,"请输入存入本金");
264 // }else if (jTextField1.getText() == null || jTextField1.getText().length() <= 0){
265 // JOptionPane.showMessageDialog( null,"请输入利率比");
266 // }else if (jTextField2.getText() == null || jTextField2.getText().length() <= 0) {
267 // JOptionPane.showMessageDialog( null,"请输入存入年限");
268 // }else if (jTextField3.getText() == null || jTextField3.getText().length() <= 0) {
269 // JOptionPane.showMessageDialog( null,"请输入年复利次数");
270 // }
271 Calculate calculate = new Calculate(jTextField0.getText(), jTextField1.getText(), jTextField2.getText(), jTextField3.getText(), jTextField4.getText());
272 if(!jTextField0.isEditable()){
273 jTextField0.setText(calculate.calculatePrincipal());
274 }else if (!jTextField2.isEditable()) {
275 jTextField2.setText(calculate.calculateYears());
276 }else if (!jTextField4.isEditable()) {
277 if(jRadioButton1.isSelected()){
278 jTextField4.setText(calculate.calculateCompoundInterest());
279 }else {
280 jTextField4.setText(calculate.calculatSimpleInterest());
281 }
282 }
283
284
285 }
286 public void clear() {
287 jTextField0.setText(null);
288 jTextField1.setText(null);
289 jTextField2.setText(null);
290 jTextField3.setText(null);
291 jTextField4.setText(null);
292 }
293
294 private void jRadioButton0MouseMouseClicked(MouseEvent event) {
295 clear();
296 jRadioButton0.setSelected(true);
297 jRadioButton1.setSelected(false);
298 jRadioButton2.setSelected(false);
299 jRadioButton3.setSelected(false);
300 jTextField0.setEditable(false);
301 jTextField2.setEditable(true);
302 jTextField3.setEditable(true);
303 jTextField4.setEditable(true);
304 }
305
306 private void jRadioButton1MouseMouseClicked(MouseEvent event) {
307 clear();
308 jRadioButton0.setSelected(false);
309 jRadioButton1.setSelected(true);
310 jRadioButton2.setSelected(false);
311 jRadioButton3.setSelected(false);
312 jTextField0.setEditable(true);
313 jTextField2.setEditable(true);
314 jTextField3.setEditable(true);
315 jTextField4.setEditable(false);
316 jLabel4.setText("复利终值:");
317 }
318
319 private void jRadioButton2MouseMouseClicked(MouseEvent event) {
320 clear();
321 jRadioButton0.setSelected(false);
322 jRadioButton1.setSelected(false);
323 jRadioButton2.setSelected(true);
324 jRadioButton3.setSelected(false);
325 jTextField0.setEditable(true);
326 jTextField2.setEditable(false);
327 jTextField3.setEditable(true);
328 jTextField4.setEditable(true);
329 }
330
331 private void jRadioButton3MouseMouseClicked(MouseEvent event) {
332 clear();
333 jRadioButton0.setSelected(false);
334 jRadioButton1.setSelected(false);
335 jRadioButton2.setSelected(false);
336 jRadioButton3.setSelected(true);
337 jTextField0.setEditable(true);
338 jTextField2.setEditable(true);
339 jTextField3.setEditable(false);
340 jTextField4.setEditable(false);
341 jLabel4.setText("单利本息:");
342
343 }
344
345 }
实现计算复利,计算本金,计算单利,计算存款时间
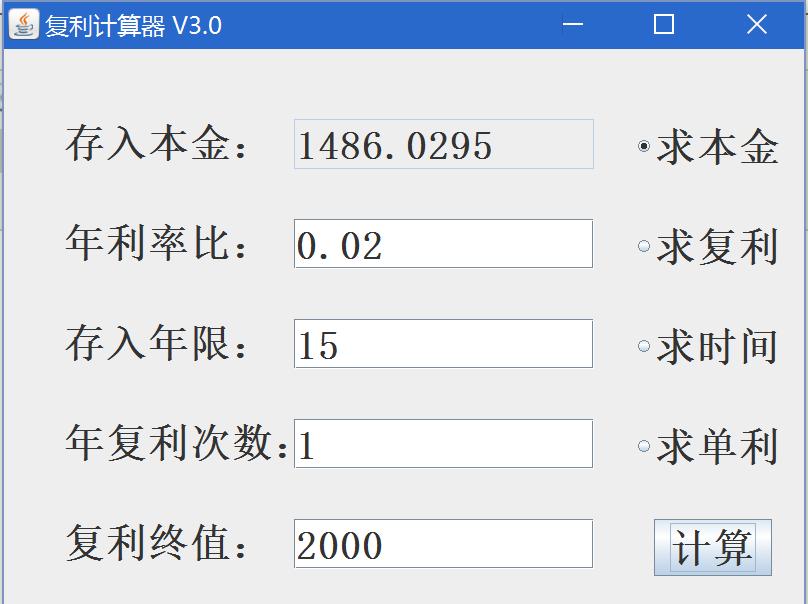