package cn.hy.weixin.util;
import net.sf.json.JSONObject;
public class CommonUtil {
public static String OPENID_ID = "https://api.weixin.qq.com/sns/oauth2/access_token?appid=APPID&secret=APPSECRET&code=CODE&grant_type=authorization_code";
/**
* 根据code获取openid
* @param code
* @return
*/
public static String getOpenId(String code){
String openid = null;
String url = OPENID_ID.replace("APPID", HttpUtil.APPID).replace("APPSECRET", HttpUtil.APPSECRET).replace("CODE", code);
JSONObject jsonObject = HttpUtil.doGetRequest(url);
if(jsonObject != null){
openid = jsonObject.getString("openid");
}
return openid;
}
}
package cn.hy.weixin.util;
import java.io.IOException;
import java.io.UnsupportedEncodingException;
import java.util.List;
import net.sf.json.JSONObject;
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.NameValuePair;
import org.apache.http.client.ClientProtocolException;
import org.apache.http.client.HttpClient;
import org.apache.http.client.entity.UrlEncodedFormEntity;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.entity.StringEntity;
import org.apache.http.impl.client.DefaultHttpClient;
import org.apache.http.util.EntityUtils;
/**
* http工具类
*
*/
public class HttpUtil {
public static final String APPID = "xxx";
public static final String APPSECRET = "xxx";
public static final String ACCESS_TOKEN_URL = "https://api.weixin.qq.com/cgi-bin/token?grant_type=client_credential&appid=APPID&secret=APPSECRET";
public static final String CREATE_MENU_URL = "https://api.weixin.qq.com/cgi-bin/menu/create?access_token=ACCESS_TOKEN";
/**
* 封装get请求
* @param url
* @return
*/
public static JSONObject doGetRequest(String url){
DefaultHttpClient httpClient = new DefaultHttpClient();
HttpClient newhttpClient = WebClientDevWrapper.wrapClient(httpClient);
System.out.println("url**************" + url);
HttpGet httpGet = new HttpGet(url);
JSONObject jsonObject = null;
try {
HttpResponse response = newhttpClient.execute(httpGet);
HttpEntity entity = response.getEntity();
String result = EntityUtils.toString(entity, "UTF-8");
jsonObject = JSONObject.fromObject(result);
} catch (Exception e) {
e.printStackTrace();
}
return jsonObject;
}
/**
* 封装post请求
* @param url
* @param prarm
* @return
*/
public static JSONObject doPostRequest(String url,String prarm){
DefaultHttpClient httpClient = new DefaultHttpClient();
HttpClient newhttpClient = WebClientDevWrapper.wrapClient(httpClient);
HttpPost httpPost = new HttpPost(url);
JSONObject jsonObject = null;
try {
httpPost.setEntity(new StringEntity(prarm, "UTF-8"));
HttpResponse response = newhttpClient.execute(httpPost);
HttpEntity entity = response.getEntity();
String result = EntityUtils.toString(entity, "UTF-8");
jsonObject = JSONObject.fromObject(result);
} catch (Exception e) {
e.printStackTrace();
}
return jsonObject;
}
/**
* 发送短息 post请求并返回结果
*/
public static String post(String url,String reqEncoding,String respEncoding,List<NameValuePair> param) {
HttpClient httpclient=new DefaultHttpClient();
String resStr = "";
// 创建httppost
HttpPost httppost = new HttpPost(url);
// 创建参数队列
List<NameValuePair> formparams = param;
UrlEncodedFormEntity uefEntity;
try {
uefEntity = new UrlEncodedFormEntity(formparams, reqEncoding);
httppost.setEntity(uefEntity);
HttpResponse response;
response = httpclient.execute(httppost);
HttpEntity entity = response.getEntity();
if (entity != null) {
resStr = EntityUtils.toString(entity,respEncoding);
}
} catch (ClientProtocolException e) {
e.printStackTrace();
} catch (UnsupportedEncodingException e1) {
e1.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
} finally {
// 关闭连接,释放资源
httpclient.getConnectionManager().shutdown();
}
return resStr;
}
}
捐助开发者
在兴趣的驱动下,写一个
免费
的东西,有欣喜,也还有汗水,希望你喜欢我的作品,同时也能支持一下。 当然,有钱捧个钱场(右上角的爱心标志,支持支付宝和PayPal捐助),没钱捧个人场,谢谢各位。
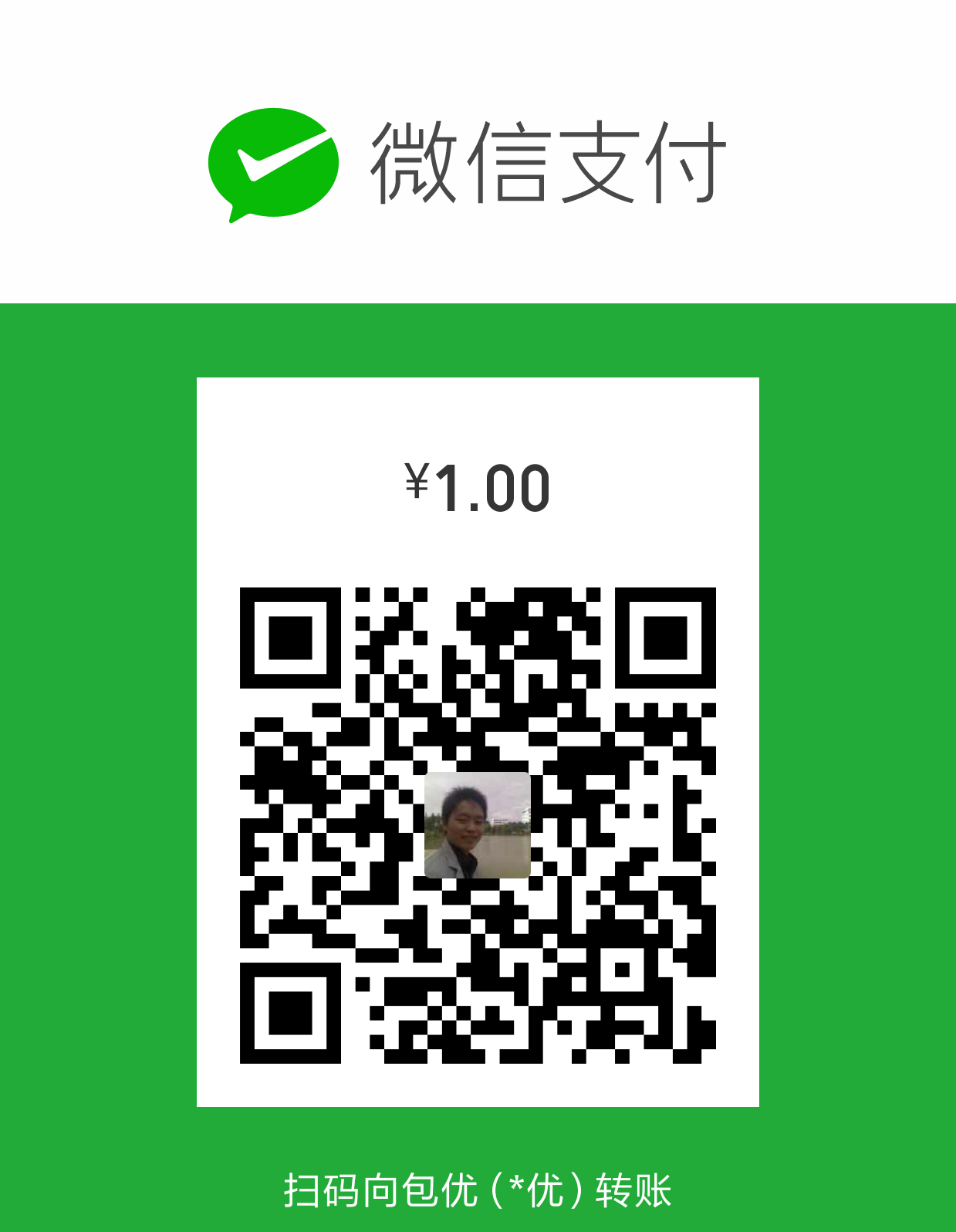
谢谢您的赞助,我会做的更好!