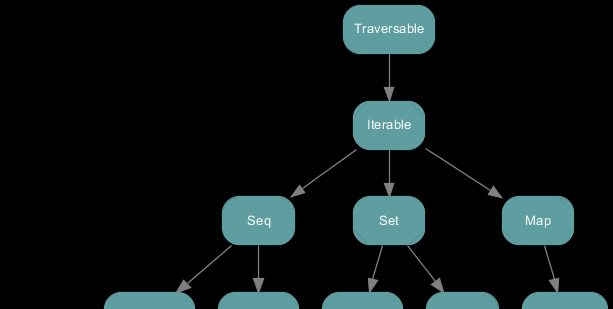
在sequences上操作,如下表,分为几种:
Indexing and length
方法:<code>apply</code>, <code>isDefinedAt</code>, <code>length</code>, <code>indices</code>, and<code>lengthCompare</code>
apply方法用于建立索引,因此类型Seq[T]的一个序列是一个partial函数,他获取一个int参数,并且产生了一个类型T的序列。综上,Seq[T]扩展了 <code>PartialFunction[Int, T]。</code>
<code> <code>lengthCompare</code> </code>允许比较两个sequences序列的长度,甚至有一个可以是无限大
Index search operations
方法:<code>indexOf</code>, <code>lastIndexOf</code>, <code>indexOfSlice</code>, <code>lastIndexOfSlice</code>,<code>indexWhere</code>, <code>lastIndexWhere</code>,
<code>segmentLength</code>, <code>prefixLength</code>返回索引或者匹配一些断言
Addition operations
方法:<code>+:</code>, <code>:+</code>, <code>padTo</code>
返回一些新的序列,通过头尾增加元素
Update operations
方法:<code>updated</code>, <code>patch</code>
返回一个新的序列,通过更新
Sorting operations
方法:<code>sorted</code>, <code>sortWith</code>, <code>sortBy</code>,
排序,根据多个标准
reversal operations
方法:<code>reverse</code>, <code>reverseIterator</code>, <code>reverseMap</code>
倒序产生或者处理一个序列
Comparisons
方法:<code>startsWith</code>, <code>endsWith</code>, <code>contains</code>, <code>containsSlice</code>, <code>corresponds</code>
比较连个元素或者在一个序列中查找一个元素
Multiset
方法:<code>intersect</code>, <code>diff</code>, <code>union</code>, <code>distinct</code>
在两个序列的元素上做类set的操作,或者移除重复值(这个估计是指单序列中)
如果一个序列是可变的(mutable),会提供一个额外的方法,update(书生:区别updated),它能让序列的元素更新。正如在Scala中,语法如seq(idx)=elem 仅仅是seq.update(idx,elem)的一个简写,可以说update给予了一些语法上的便利。
updates: 返回一个被修改后的新的序列代替原序列,对所有序列有效。
update: 修改序列中的元素, 只对可变mutable序列有效
Class Seq 函数
序列
WHAT IT IS
WHAT IT DOES
Indexing and Length:
<code>xs(i)</code>
(or, written out, <code>xs apply i</code>). The element of <code>xs</code> at index <code>i</code>.
<code>xs isDefinedAt i</code>
Tests whether <code>i</code> is contained in <code>xs.indices</code>.
<code>xs.length</code>
The length of the sequence (same as <code>size</code>).
<code>xs.lengthCompare ys</code>
Returns <code>-1</code> if <code>xs</code> is shorter than <code>ys</code>, <code>+1</code> if it is longer, and <code>0</code> is they have the same length. Works even if one if the sequences is infinite.
<code>xs.indices</code>
The index range of <code>xs</code>, extending from <code>0</code> to <code>xs.length - 1</code>.
Index Search:
<code>xs indexOf x</code>
The index of the first element in <code>xs</code> equal to <code>x</code> (several variants exist).
<code>xs lastIndexOf x</code>
The index of the last element in <code>xs</code> equal to <code>x</code> (several variants exist).
<code>xs indexOfSlice ys</code>
The first index of <code>xs</code> such that successive elements starting from that index form the sequence <code>ys</code>.
<code>xs lastIndexOfSlice ys</code>
The last index of <code>xs</code> such that successive elements starting from that index form the sequence <code>ys</code>.
<code>xs indexWhere p</code>
The index of the first element in xs that satisfies <code>p</code> (several variants exist).
<code>xs segmentLength (p, i)</code>
The length of the longest uninterrupted segment of elements in <code>xs</code>, starting with <code>xs(i)</code>, that all satisfy the predicate <code>p</code>.
<code>xs prefixLength p</code>
The length of the longest prefix of elements in <code>xs</code> that all satisfy the predicate <code>p</code>.
Additions:
<code>x +: xs</code>
A new sequence that consists of <code>x</code> prepended to <code>xs</code>.
<code>xs :+ x</code>
A new sequence that consists of <code>x</code> appended to <code>xs</code>.
<code>xs padTo (len, x)</code>
The sequence resulting from appending the value <code>x</code> to <code>xs</code> until length <code>len</code> is reached.
Updates:
<code>xs patch (i, ys, r)</code>
The sequence resulting from replacing <code>r</code> elements of <code>xs</code> starting with <code>i</code> by the patch <code>ys</code>.
<code>xs updated (i, x)</code>
A copy of <code>xs</code> with the element at index <code>i</code> replaced by <code>x</code>.
<code>xs(i) = x</code>
(or, written out, <code>xs.update(i, x)</code>, only available for <code>mutable.Seq</code>s). Changes the element of <code>xs</code> at index <code>i</code> to <code>x</code>.
Sorting:
<code>xs.sorted</code>
A new sequence obtained by sorting the elements of <code>xs</code> using the standard ordering of the element type of <code>xs</code>.
<code>xs sortWith lt</code>
A new sequence obtained by sorting the elements of <code>xs</code> using <code>lt</code> as comparison operation.
<code>xs sortBy f</code>
A new sequence obtained by sorting the elements of <code>xs</code>. Comparison between two elements proceeds by mapping the function <code>f</code> over both and comparing the results.
Reversals:
<code>xs.reverse</code>
A sequence with the elements of <code>xs</code> in reverse order.
<code>xs.reverseIterator</code>
An iterator yielding all the elements of <code>xs</code> in reverse order.
<code>xs reverseMap f</code>
A sequence obtained by mapping <code>f</code> over the elements of <code>xs</code> in reverse order.
Comparisons:
<code>xs startsWith ys</code>
Tests whether <code>xs</code> starts with sequence <code>ys</code> (several variants exist).
<code>xs endsWith ys</code>
Tests whether <code>xs</code> ends with sequence <code>ys</code> (several variants exist).
<code>xs contains x</code>
Tests whether <code>xs</code> has an element equal to <code>x</code>.
<code>xs containsSlice ys</code>
Tests whether <code>xs</code> has a contiguous subsequence equal to <code>ys</code>.
<code>(xs corresponds ys)(p)</code>
Tests whether corresponding elements of <code>xs</code> and <code>ys</code> satisfy the binary predicate <code>p</code>.
Multiset Operations:
<code>xs intersect ys</code>
The multi-set intersection of sequences <code>xs</code> and <code>ys</code> that preserves the order of elements in <code>xs</code>.
<code>xs diff ys</code>
The multi-set difference of sequences <code>xs</code> and <code>ys</code> that preserves the order of elements in <code>xs</code>.
<code>xs union ys</code>
Multiset union; same as <code>xs ++ ys</code>.
<code>xs.distinct</code>
A subsequence of <code>xs</code> that contains no duplicated element.
Vector提供了一个令人感兴趣的折中,在indexed和linear,他能有效的确保稳定的index和linear访问的时间总开销 。 因此,他是一个非常好的混合访问模式。
本文转自 wws5201985 51CTO博客,原文链接:http://blog.51cto.com/yjplxq/1432054,如需转载请自行联系原作者