【本文链接】
【题目】
在8×8的国际象棋上摆放八个皇后,使其不能相互攻击,即任意两个皇后不得处在同一行、同一列或者同一对角斜线上。下图中的每个黑色格子表示一个皇后,这就是一种符合条件的摆放方法。请求出总共有多少种摆法。
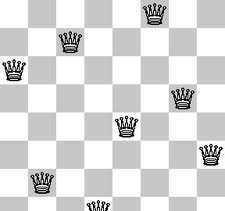
【分析】
之前博文介绍过字符串的全排列,八皇后问题也可以转换为全排列问题。
由于八个皇后的任意两个不能处在同一行,那么每一个皇后占据一行。于是我们可以定义一个数组pos[8],pos[i]=value表示第i行将皇后放置于value位置。先把pos的八个数字分别用0-7初始化,接下来对数组pos数组做全排列。由于我们是用不同的数字初始化数组中的数字,因此任意两个皇后肯定不同列。我们只需要判断得到的每一个排列对应的八个皇后是不是在同一对角斜线上,也就是数组的两个下标i和j,是不是i-j==pos
[i]- pos [j]或者j-i==pos [i]- pos [j]。
【代码】
C++
Code
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
// 54_EightQueensPuzzle.cpp : Defines the entry point for the console application.
//
/*
version: 1.0
author: hellogiser
blog: http://www.cnblogs.com/hellogiser
date: 2014/5/24
*/
#include "stdafx.h"
#include <iostream>
using namespace std;
#define N 8
int m_nPermutationCount = 0;
int m_nValidCount = 0;
// swap a and b
void Swap(int &a, int &b)
{
int t = a;
a = b;
b = t;
}
// check whether pos array is valid
bool CheckValid(int *pos, int n)
for (int i = 0; i < n; ++i)
{
for (int j = i + 1; j < n; j++)
{
//only need to check whether pos[i] and pos[j] are on diagonal
if (i - j == pos[i] - pos[j] || j - i == pos[i] - pos[j])
{
return false;
}
}
}
return true;
// print pos array
void Print(int *pos, int n)
printf("%d ", pos[i]);
void Permutation(int *pos, int n, int index)
m_nPermutationCount++;
if (index == n)
// this permutation generated
if (CheckValid(pos, n))
// valid permutation
m_nValidCount++;
//Print(pos,n);
}
else
for (int i = index; i < n; ++i)
Swap(pos[index], pos[i]);
Permutation(pos, n, index + 1);
void EightQueensPuzzle()
// init pos
int pos[N];
for (int i = 0; i < N; i++)
pos[i] = i;
Permutation(pos, N, 0);
void test_main()
EightQueensPuzzle();
cout << m_nPermutationCount << endl;
cout << m_nValidCount << endl; //92
int _tmain(int argc, _TCHAR *argv[])
test_main();
return 0;
【参考】