在微服務架構中,根據業務來拆分成一個個的服務,服務與服務之間可以互相調用(RPC),在Spring Cloud可以用RestTemplate+Ribbon和Feign來調用。為了保證其高可用,單個服務通常會叢集部署。由于網絡原因或者自身的原因,服務并不能保證100%可用,如果單個服務出現問題,調用這個服務就會出現線程阻塞,此時若有大量的請求湧入,Servlet容器的線程資源會被消耗完畢,導緻服務癱瘓。服務與服務之間的依賴性,故障會傳播,會對整個微服務系統造成災難性的嚴重後果,這就是服務故障的“雪崩”效應。
為了解決這個問題,業界提出了斷路器模型。
一、斷路器簡介
Netflix has created a library called Hystrix that implements the circuit breaker pattern. In a microservice architecture it is common to have multiple layers of service calls.
. —-摘自官網
Netflix開源了Hystrix元件,實作了斷路器模式,SpringCloud對這一元件進行了整合。 在微服務架構中,一個請求需要調用多個服務是非常常見的,如下圖:
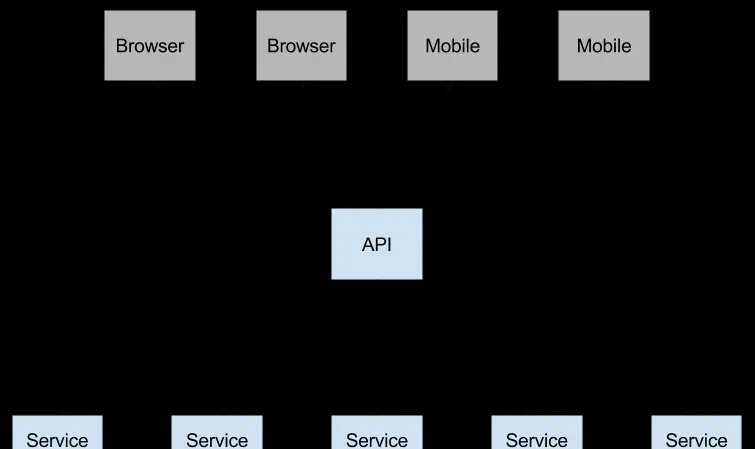
較底層的服務如果出現故障,會導緻連鎖故障。當對特定的服務的調用的不可用達到一個閥值(Hystric 是5秒20次) 斷路器将會被打開。
斷路打開後,可用避免連鎖故障,fallback方法可以直接傳回一個固定值。
二、Ribbon+Hystrix
pom檔案添加jar檔案
<!-- 斷路器 -->
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-netflix-hystrix</artifactId>
</dependency>
啟動類添加@EnableHystrix 注解
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cloud.client.discovery.EnableDiscoveryClient;
import org.springframework.cloud.client.loadbalancer.LoadBalanced;
import org.springframework.cloud.netflix.hystrix.EnableHystrix;
import org.springframework.context.annotation.Bean;
import org.springframework.web.client.RestTemplate;
@SpringBootApplication
@EnableDiscoveryClient // 定義為用戶端
@EnableHystrix // 設定斷路器
public class RibbonApplication {
public static void main(String[] args) {
SpringApplication.run(RibbonApplication.class, args);
}
/**
* @LoadBalanced注解表明這個restRemplate開啟負載均衡的功能。
* @return
*/
@Bean
@LoadBalanced
RestTemplate restTemplate() {
return new RestTemplate();
}
}
HelloService類配置斷路方法
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import org.springframework.web.client.RestTemplate;
import com.netflix.hystrix.contrib.javanica.annotation.HystrixCommand;
/**
* 問題一: 什麼是REST REST(RepresentationalState Transfer)是Roy Fielding
* 提出的一個描述互聯系統架構風格的名詞。REST定義了一組體系架構原則,您可以根據這些原則設計以系統資源為中心的Web
* 服務,包括使用不同語言編寫的用戶端如何通過 HTTP處理和傳輸資源狀态。
*
* 為什麼稱為 REST?Web本質上由各種各樣的資源組成,資源由URI
* 唯一辨別。浏覽器(或者任何其它類似于浏覽器的應用程式)将展示出該資源的一種表現方式,或者一種表現狀态。
* 如果使用者在該頁面中定向到指向其它資源的連結,則将通路該資源,并表現出它的狀态。
* 這意味着用戶端應用程式随着每個資源表現狀态的不同而發生狀态轉移,也即所謂REST。
*
* 問題二: RestTemplate Spring'scentral class for synchronous client-side HTTP
* access.It simplifies communication with HTTPservers, and enforces RESTful
* principles. Ithandles HTTP connections, leaving application code to provide
* URLs(with possible template variables) andextract results.
* 簡單說就是:簡化了發起HTTP請求以及處理響應的過程,并且支援REST
*
* @author 于志強
*
* 2018年11月28日 上午11:10:45
*/
@Service
public class HelloService {
@Autowired
RestTemplate restTemplate; //
@HystrixCommand(fallbackMethod = "hiError") // hiError是你服務斷後走的方法名字
public String hiService() {
return restTemplate.getForObject("http://CLIENTNAME/test", String.class);
}
public String hiError() {
return "頁面通路不到,走到失敗結果";
}
}
三、測試方法
啟動discSystem-3
啟動ribbon項目
通路http://localhost:12348/demo
啟動discSystem-4項目
------------------------------------------------------------------------------------------------------------------------------------------------------
一、Feign+Hystrix
Feign是自帶斷路器的,在D版本的Spring Cloud中,它沒有預設打開。需要在配置檔案中配置打開它,在配置檔案加以下代碼:
feign:
hystrix:
enabled: true
SchedualCilentName類添加fallback = SchedualServiceHiHystric.class SchedualServiceHiHystric 為該接口實作類
import org.springframework.cloud.openfeign.FeignClient;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
/**
* 定義一個Feign接口類
* @author 于志強
*
* 2018年11月28日 上午11:43:20
*/
@FeignClient(value = "clientName",fallback = SchedualServiceHiHystric.class)
public interface SchedualCilentName {
// value值必須與discSystem-4、discSystem-4-1中方法名一緻
@RequestMapping(value = "/test",method = RequestMethod.GET)
String sayHiFromClientOne();
}
建立SchedualServiceHiHystric類(SchedualServiceHiHystric需要實作SchedualServiceHi 接口,并注入到Ioc容器中)
import org.springframework.stereotype.Component;
@Component
public class SchedualServiceHiHystric implements SchedualCilentName {
@Override
public String sayHiFromClientOne() {
return "sorry";
}
}
不需要修改pom檔案
二、測試方法
啟動discSystem-3
啟動service-feign項目
通路http://localhost:12349/demo
啟動discSystem-4項目