原作者:尚矽谷-佟剛
forEach标簽
ForEachTag.java
package com.atweihai.javaweb.mytag;
import java.io.IOException;
import java.util.Collection;
import javax.servlet.jsp.JspException;
import javax.servlet.jsp.tagext.SimpleTagSupport;
public class ForEachTag extends SimpleTagSupport{
private Collection<?> item;
private String var;
public void setItem(Collection<?> item) {
this.item = item;
}
public void setVar(String var) {
this.var = var;
}
@Override
public void doTag() throws JspException, IOException {
//循環周遊集合
if(item!=null&&item.size()>){
for(Object obj:item){
//放入PageContext對象中,鍵:var 值:每次周遊的對象
getJspContext().setAttribute(var,obj);
//标簽體解析後輸出
getJspBody().invoke(null);
}
}
}
}
mytag.tld
<tag>
<name>forEach</name>
<tag-class>com.atweihai.javaweb.mytag.ForEachTag</tag-class>
<!-- tagdependent:标簽體内容直接被寫入BodyContent,由自定義标簽類來進行處理,而不被JSP容器解釋
JSP:接受所有JSP文法,如定制的或内部的tag、scripts、靜态HTML、腳本元素、JSP指令和動作
empty:空标記,即起始标記和結束标記之間沒有内容
scriptless:标簽體可以包含EL表達式和JSP動作元素,但不能包含JSP的腳本元素和表達式元素
-->
<body-content>scriptless</body-content>
<attribute>
<name>item</name>
<required>true</required>
<rtexprvalue>true</rtexprvalue>
</attribute>
<attribute>
<name>var</name>
<required>true</required>
<rtexprvalue>false</rtexprvalue>
</attribute>
</tag>
mytag.jsp
<%@taglib prefix="atweihai" uri="http://com.atweihai/jsp/mytag/core" %>
<%
List<Customer> customers=new ArrayList<Customer>();
customers.add(new Customer(,"AA",));
customers.add(new Customer(,"BB",));
customers.add(new Customer(,"CC",));
customers.add(new Customer(,"DD",));
customers.add(new Customer(,"EE",));
customers.add(new Customer(,"FF",));
request.setAttribute("customers", customers);
%>
<atweihai:forEach item="${requestScope.customers }" var="cust">
${cust.id }--${cust.name }--${cust.age }<br>
</atweihai:forEach>
頁面運作效果
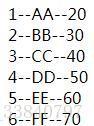
choose when otherwise 标簽
ChooseTag.java
package com.atweihai.javaweb.mytag;
import java.io.IOException;
import javax.servlet.jsp.JspException;
import javax.servlet.jsp.tagext.SimpleTagSupport;
public class ChooseTag extends SimpleTagSupport {
private boolean flag=true;
public void setFlag(boolean flag) {
this.flag = flag;
}
public boolean isFlag() {
return flag;
}
@Override
public void doTag() throws JspException, IOException {
getJspBody().invoke(null);
}
}
WhenTag.java
package com.atweihai.javaweb.mytag;
import java.io.IOException;
import javax.servlet.jsp.JspException;
import javax.servlet.jsp.tagext.SimpleTagSupport;
public class WhenTag extends SimpleTagSupport {
private boolean test;
public void setTest(boolean test) {
this.test = test;
}
@Override
public void doTag() throws JspException, IOException {
if(test){
ChooseTag parent=(ChooseTag) getParent();
boolean flag =parent.isFlag();
if(flag){
getJspBody().invoke(null);
parent.setFlag(false);
}
}
}
}
OtherwiseTag.java
package com.atweihai.javaweb.mytag;
import java.io.IOException;
import javax.servlet.jsp.JspException;
import javax.servlet.jsp.tagext.SimpleTagSupport;
public class OtherwiseTag extends SimpleTagSupport {
@Override
public void doTag() throws JspException, IOException {
ChooseTag parent= (ChooseTag) getParent();
boolean flag=parent.isFlag();
if(flag){
getJspBody().invoke(null);
}
}
}
mytag.tld
<tag>
<name>choose</name>
<tag-class>com.atweihai.javaweb.mytag.ChooseTag</tag-class>
<body-content>scriptless</body-content>
</tag>
<tag>
<name>when</name>
<tag-class>com.atweihai.javaweb.mytag.WhenTag</tag-class>
<body-content>scriptless</body-content>
<attribute>
<name>test</name>
<required>true</required>
<rtexprvalue>true</rtexprvalue>
</attribute>
</tag>
<tag>
<name>otherwise</name>
<tag-class>com.atweihai.javaweb.mytag.OtherwiseTag</tag-class>
<body-content>scriptless</body-content>
</tag>
mytag.jsp
<%@ taglib prefix="atweihai" uri="http://com.atweihai/jsp/mytag/core" %>
<atweihai:choose>
<atweihai:when test="${param.age > 24}">大學畢業</atweihai:when>
<atweihai:when test="${param.age > 20}">高中畢業</atweihai:when>
<atweihai:otherwise>高中以下</atweihai:otherwise>
</atweihai:choose>
頁面運作結果
EL自定義函數 substring(str,beginIndex,length)
substring(“abcdefghijklmn”,3,4) ===>defg
SubString.java
package com.atweihai.javaweb.mytag;
public class SubString {
public static String substring(String str,int beginIndex,int length){
return str.substring(beginIndex, beginIndex+length);
}
}
mytag.tld
<function>
<name>substring</name>
<function-class>com.atweihai.javaweb.mytag.SubString</function-class>
<function-signature>java.lang.String substring (java.lang.String,int,int)</function-signature>
</function>
mytag.jsp
<%@ taglib prefix="atweihai" uri="http://com.atweihai/jsp/mytag/core" %>
${atweihai:substring("abcdefghijklmn",,) }
頁面運作結果