加載一個線上網頁
下例是一個最簡單的WebView的使用,給定一個url網址,用WebView加載渲染出來。
import React, { Component } from 'react';
import { WebView } from 'react-native';
export default class WebViewSimpleDemo extends Component {
render() {
return (
<WebView
source={{uri: 'http://blog.csdn.net/column/details/17061.html'}}
/>
);
}
}
效果圖:
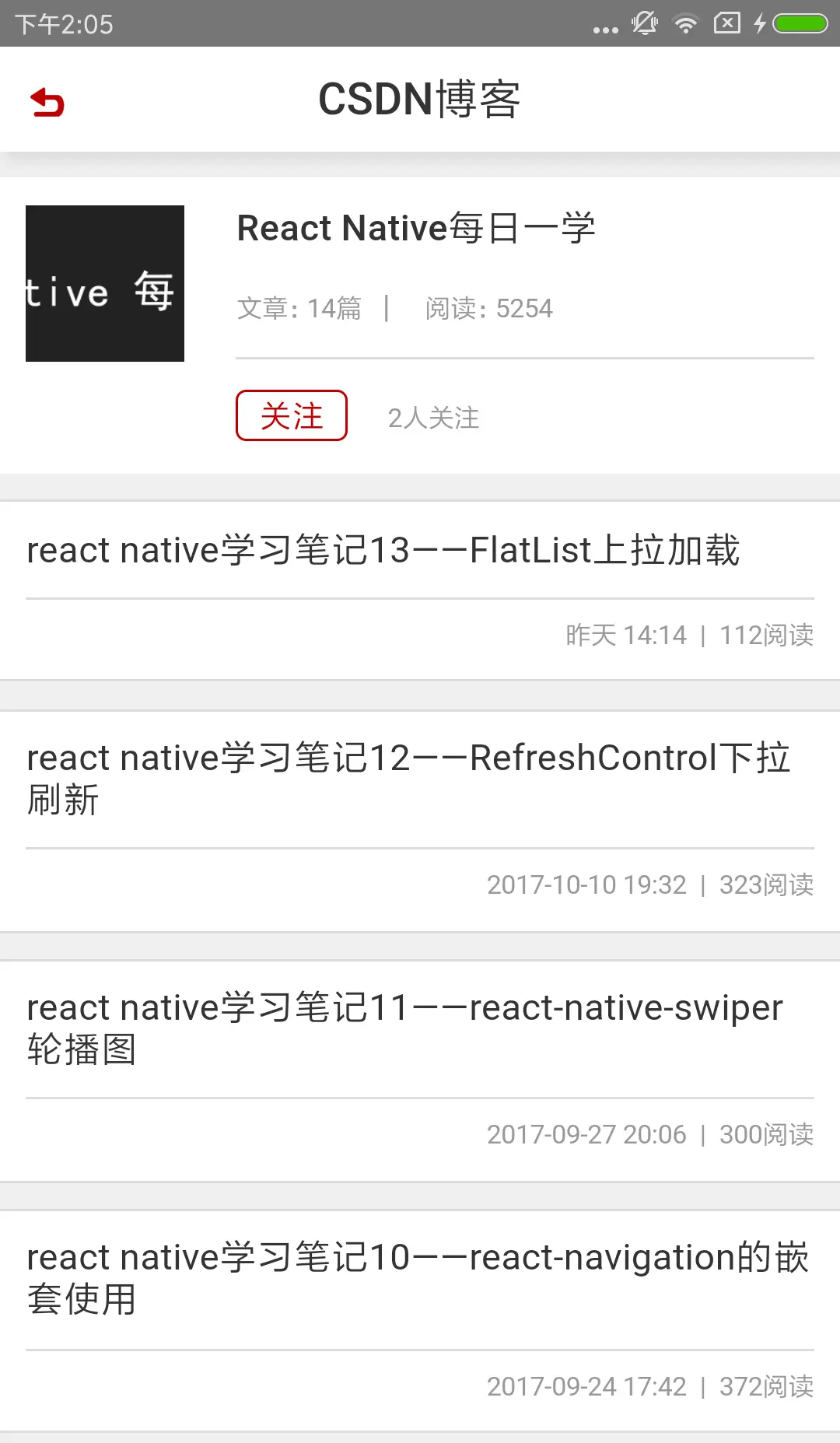
通常項目中使用WebView并不是簡單的加載一個靜态網頁,例如顯示一個加載頁,提供一個統一的加載失敗頁,webview如何與rn雙向通訊等。為了能完成上述需求,我們還需要學習WebView的基本屬性和方法。
基本屬性
屬性 | 類型 | 描述 |
---|---|---|
source | {uri: string, method: string, headers: object, body: string}, {html: string, baseUrl: string}, number | 在WebView中載入一段靜态的html代碼或是一個url(還可以附帶一些 header 選項) |
automaticallyAdjustContentInsets | bool | 設定是否自動調整内容。 |
contentInset | {top:number,left:number, bottom:number,right:number} | 設定網頁内嵌邊距。 |
startInLoadingState | bool | 在第一次加載時是否顯示loading視圖。預設true。 |
bounces(iOS) | bool | 指定滑動到邊緣後是否有回彈效果。預設true。 |
scalesPageToFit(iOS) | bool | 設定是否要把網頁縮放到适應視圖的大小,以及是否允許使用者改變縮放比例。 |
scrollEnabled(iOS) | bool | 設定是否開啟頁面滾動。 |
domStorageEnabled(Android) | bool | 是否開啟DOM存儲。 |
javaScriptEnabled(Android) | bool | 是否開啟 JavaScript,在 iOS 中的 WebView 是預設開啟的。 |
基本方法
方法名 | 描述 |
---|---|
injectJavaScript | 給WebView注入JS代碼,注入後會立即執行。 |
onNavigationStateChange | 當導航狀态發生變化的時候調用。 |
onLoadStart | 當網頁開始加載的時候調用。 |
onError | 當網頁加載失敗的時候調用。 |
onLoad | 當網頁加載結束的時候調用。 |
onLoadEnd | 當網頁加載結束調用,不管是成功還是失敗。 |
renderLoading | WebView元件正在渲染頁面時觸發的函數,隻有 startInLoadingState 為 true 時該函數才起作用。 |
renderError | 監聽渲染頁面出錯的函數。 |
onShouldStartLoadWithRequest(iOS) | 該方法允許攔截 WebView 加載的 URL 位址,進行自定義處理。該方法通過傳回 true 或者 false 來決定是否繼續加載該攔截到請求。 |
根據上面介紹的基本屬性方法,我們在加載WebView中增加加載中和加載失敗的頁面顯示。
<WebView
source={{uri: 'http://blog.csdn.net/column/details/17061.html'}}
renderError={() => {
console.log('renderError')
return <View><Text>renderError</Text></View>
}}
renderLoading={() => {
return <View><Text>自定義Loading...</Text></View>
}}
/>
renderError加載錯誤時會回調該函數,顯示該函數傳回的View。使用此方法我們可以自定義加載錯誤時的提示資訊。
類似的renderLoading可以自定義加載顯示的view。
加載本地靜态html檔案
import React, { Component } from 'react';
import { WebView } from 'react-native';
export default class WebViewSimpleDemo extends Component {
render() {
return (
<WebView
source={require('./helloworld.html')}
/>
);
}
}
helloworld.html
<!DOCTYPE html>
<html>
<head>
<title>Hello World</title>
<meta http-equiv="content-type" content="text/html; charset=utf-8">
<meta name="viewport" content="width=320, user-scalable=no">
<style type="text/css">
body {
margin: ;
padding: ;
font: % arial, sans-serif;
background: #ccc;
}
h1 {
padding: px;
margin: ;
text-align: center;
color: #f33;
}
</style>
</head>
<body>
<h1>hello world!</h1>
</body>
</html>
效果圖:
React Native與Html通信
React Native向html發資料——postMessage
React Native向html發送資料可以通過postMessage方法實作。
-
React Native端
設定WebView的ref屬性,将元件view作為參數指派給了this.webview
<WebView
ref={(webview) => this.webview = webview}
source={require('./messaging.html')}
/>
然後可通過postMessage方法從React Native向html發送資料
this.webview.postMessage('我是React Native發送過來的資料');
-
html端
html端接收React Native消息的處理:
var messagesReceivedFromReactNative = ;
document.addEventListener('message', function(e) {
messagesReceivedFromReactNative += ;
document.getElementsByTagName('p')[].innerHTML =
'從React Native接收的消息: ' + messagesReceivedFromReactNative;
document.getElementsByTagName('p')[].innerHTML = e.data;
});
React Native接收html發過來的資料——onMessage
React Native接收html發過來的資料可以通過onMessage方法實作。
-
html端
html端通過調用window.postMessage給React Native發送資料。
document.getElementsByTagName('button')[].addEventListener('click', function() {
window.postMessage('這是html發送到RN的消息');
});
-
React Native端
當有html發送資料過來時會觸發WebView的onMessage,在onMessage中處理接收到的資料。
<WebView
ref={(webview) => this.webview = webview}
source={require('./messaging.html')}
onMessage={this._onMessage}
/>
//接收HTML發出的資料
_onMessage = (e) => {
Alert.alert(e.nativeEvent.data);
}
效果圖
React Native與Html通信的完整代碼在Github