項目介紹
本項目使用spring mybaits 前端使用jsp JavaScript css
功能
所有圖書:可以查詢所有的圖書資訊 進行檢視 借閱、編輯、下架
上新圖書:添加新的圖書資訊
下架圖書:檢視所有已經下架的圖書資訊,可以重新上架
圖書借閱記錄:查詢所有的借閱資訊。
讀者管理:對所有的借閱者進行管理
全部預期:檢視所有已經借出并且沒有歸還的圖書
項目技術
後端:spring SpringMVC,mybatis
前端:jsp、js、css等
開發工具:idea/eclipse
資料庫:mysql 5.7
部分功能
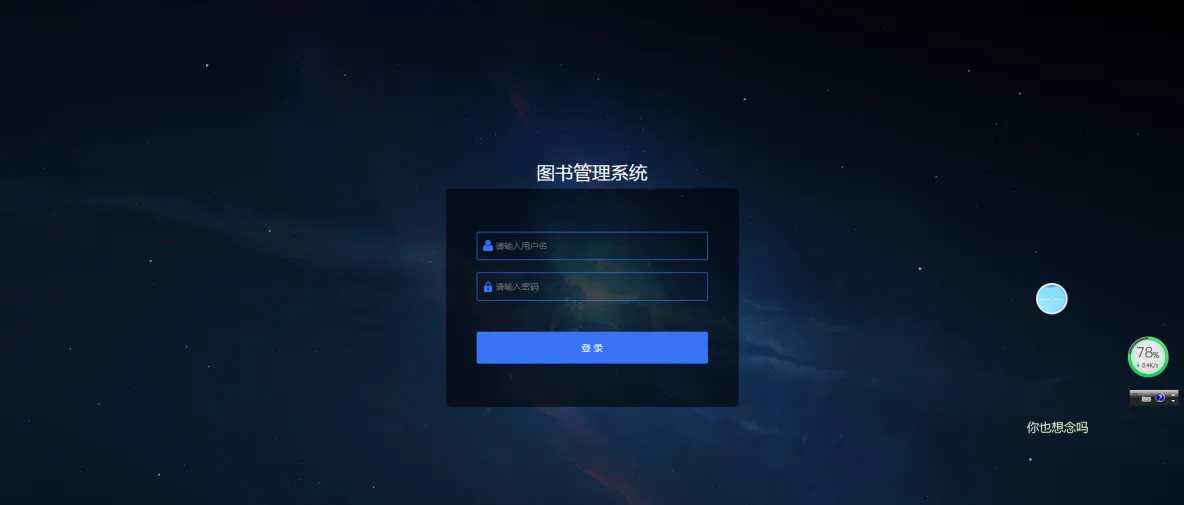
編輯
編輯
@Autowired
private MsBookService msBookService;
@Autowired
private MsCategoryService msCategoryService;
@Autowired
private BorrowBookController borrowBookController;
@RequestMapping(value = "/showBook")
public String showAllBookByPage(@RequestParam(value="currentPage",defaultValue="1",required=false)
int currentPage,String title,String author,Model model, HttpSession httpSession, HttpServletRequest request) {
logger.info("===BookController類的showAllBookByPage方法===");
model.addAttribute("pageMsg",msBookService.selectByPage(title, author, currentPage));
MsAdmin user = (MsAdmin) httpSession.getAttribute("msAdmin");
borrowBookController.checkIsPenalty(request, user.getId()); // 超期處理
return "showAllBook";
}
/******************查詢圖書***********************
*
*/
//跳轉頁面
@RequestMapping(value = "/searchBook")
public String toSearchBook() {
return "searchBook";
}
@RequestMapping(value = "/searchBookPage")
public String searchBook(@RequestParam(value="currentPage",defaultValue="1",required=false)
int currentPage,String title,String author,Model model, HttpServletRequest request) {
request.setAttribute("placeholder1", title); // 顯示查詢時輸入内容
request.setAttribute("placeholder2", author);
model.addAttribute("pageMsg",msBookService.selectByPage(title, author, currentPage));
return "searchBook";
}
/**
* ****************查詢圖書end*********************
*/
/**
* 檢視圖書詳細資訊
*/
@RequestMapping(value="/bookDetail")
public String showBookDetail(int id,Model model) {
MsBook book = msBookService.selectByID(id);
MsCategory cate = msCategoryService.selectByPrimaryKey(book.getCategoryId());
model.addAttribute("book",book);
model.addAttribute("cate", cate);
return "bookDetail";
}
/**
* 跳轉到添加圖書頁面
* @return
*/
@RequestMapping(value = "/toAddNewBook")
public String toAddNewBook() {
return "addNewBook";
}
/**
* 添加圖書
* @param book
* @param file
* @param request
* @return
*/
@RequestMapping(value="/addNewBook")
public String addNewBook(MsBook book,MultipartFile file,HttpServletRequest request,HttpSession httpSession) {
MsAdmin admin = (MsAdmin)httpSession.getAttribute("msAdmin");
System.out.println("頁面送出過來的表單:"+book);
//SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd");
book.setCreateTime(new Date());
book.setCreateAdmin(admin.getAdminName());
book.setUpdatePreAdmin(admin.getAdminName());
book.setDelFlg(1);
//String filePath = request.getSession().getServletContext().getRealPath("/bookImage");; //定義圖檔上傳後的路徑
//System.out.println("檔案上傳路徑:"+filePath);
String newFileName = fileOperate(file,Message.IMG_LOCAL_PATH);
book.setImage(newFileName);
System.out.println("添加資料後的book:"+book);
msBookService.insertBook(book);
return "redirect:newBookList";
}
/**
* 查詢最近上架的圖書
*/
@RequestMapping(value="/newBookList")
public String newBookList(Model model) {
model.addAttribute("newBookList", msBookService.selectNewBook());
return "newBookList";
}
/**
* 下架圖書
* @param id
* @return
*/
@RequestMapping(value = "/deleteBook")
public String deleteBook(int id) {
msBookService.deleteByPrimaryKey(id);
return "redirect:showBook";
}
/**
* 下架新上架的圖書
* @param id
* @return
*/
@RequestMapping(value = "/deleteBookNewList")
public String deleteBookNewList(int id) {
msBookService.deleteByPrimaryKey(id);
return "redirect:newBookList";
}
/**
* 下架查詢到的圖書
* @param id
* @return
*/
@RequestMapping(value = "/deleteSearchBook")
public String deleteSearchBook(int id) {
msBookService.deleteByPrimaryKey(id);
return "redirect:searchBook";
}
/**
* 已下架圖書清單
*/
@RequestMapping(value = "/deleteBookList")
public String deleteBookList(Model model) {
model.addAttribute("bookList", msBookService.selectBookDel());
return "delBookList";
}
/**
* 跳轉到修改書籍資訊
*/
@RequestMapping(value = "/toUpdateBook")
public String updateBookPage(int id,Model model) {
MsBook book = msBookService.selectByID(id);
MsCategory cate = msCategoryService.selectByPrimaryKey(book.getCategoryId());
model.addAttribute("book", book);
model.addAttribute("cate", cate);
return "editBook";
}
/**
* 修改圖書資訊
* @param book
* @param file
* @param httpSession
* @return
*/
@RequestMapping(value = "/updateBook")
public String updateBook(MsBook book,MultipartFile file,HttpSession httpSession) {
System.out.println("上傳過來的圖書資訊:"+book);
MsBook oldBook = msBookService.selectByID(book.getId());
book.setPublishTime(oldBook.getPublishTime());
MsAdmin admin = (MsAdmin)httpSession.getAttribute("msAdmin");
book.setUpdatePreAdmin(admin.getAdminName());
String newFileName = fileOperate(file,Message.IMG_LOCAL_PATH);
book.setImage(newFileName);
System.out.println("添加完成的圖書資訊:"+book);
msBookService.updateByPrimaryKeySelective(book);
return "redirect:showBook";
}
/**
* 重新上架圖書
*/
@RequestMapping("/updateBackBook")
public String updateBackBook(int id) {
msBookService.updateBackBook(id);
return "redirect:deleteBookList";
}
/**
* 徹底删除圖書
*/
@RequestMapping("/deleteBookReal")
public String deleteBookReal(int id) {
msBookService.deleteBookReal(id);
return "redirect:deleteBookList";
}
/**
* 封裝操作檔案方法,
* @param file
* @param filePath
* @return
*/
private String fileOperate(MultipartFile file,String filePath) {
System.out.println("進入檔案操作方法");
String originalFileName = file.getOriginalFilename();//擷取原始圖檔的擴充名
System.out.println("圖檔原始名稱:"+originalFileName);
String newFileName = UUID.randomUUID()+originalFileName; //新的檔案名稱
System.out.println("新的檔案名稱:"+newFileName);
File targetFile = new File(filePath,newFileName); //建立新檔案
try {
file.transferTo(targetFile); //把本地檔案上傳到檔案位置 , transferTo()是springmvc封裝的方法,用于圖檔上傳時,把記憶體中圖檔寫入磁盤
System.out.println("檔案上傳成功!");
} catch (IllegalStateException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
return newFileName;
}