新增節點
'設定樹狀圖中節點
Dim node As TreeNode
'Method 1: straightforward adding of nodes
With Me.TreeView1.Nodes
'add text
.Add("AddByText ")
'since with..end with is used: read TreeView1.Nodes.Add ....
'every add method returns the newly created node. You can use
'this concept set the result to a variable or to directly add
'a childnode:
.Add("AddByText2 ").Nodes.Add("ChildOfAddByText ")
'this, you can take as far as you want
.Add("AddByText3 ").Nodes.Add("ChildOfAddByText ").Nodes.Add("Another child ")
'--
node = .Add("AddByText, Attach To Variable ")
node.Nodes.Add("Child one ")
node.Nodes.Add("Child two ")
' --
With .Add("AddByText Use WithTo Add ChildNodes ").Nodes
.Add("Child 1 ")
.Add("Child 2 ")
.Add("Child 3 ").Nodes.Add("Subchild 1 ")
End With
End With
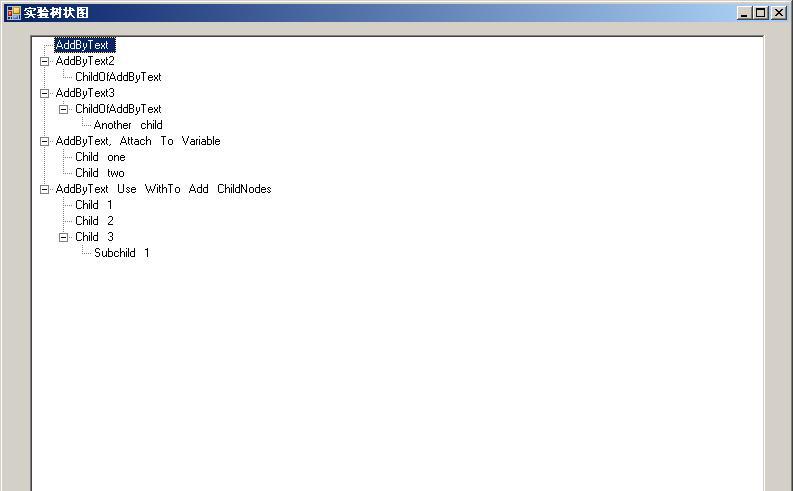
複制節點
'設定樹狀圖中節點
Dim node As TreeNode
'Method 2: adding by node
'Like virtually every .Net method you can directly assign an object:
Me.TreeView1.Nodes.Add(New TreeNode("AddByNode1 "))
Me.TreeView1.Nodes.Add("AddByNode2 ").Nodes.Add("test1")
'check out the overloading possibilities of using New()
'Another advantage of this method is that you can add a complete branch.
'(node is already declared as TreeNode above)
node = New TreeNode("MainNodeToAdd ")
node.Nodes.Add("Child 1 ")
node.Nodes.Add("Child 2 ")
'you can for instance add this newly created node to all main branches:
Dim enumNode As TreeNode
For Each enumNode In TreeView1.Nodes
enumNode.Nodes.Add(node.Clone) ' <- the clone() method is needed
Next
說明:
紅色字型部分,是将某個節點複制到指定節點之下.
注意,由于for循環的對象是TreeView1.Nodes,而節點"test1"的隸屬關系是"AddByNode2".Nodes
是以節點"test1"沒有被複制
追加節點
''Adding will always add the the node at the end of the collection.
''Of course you can also insert at a specified location:
Me.TreeView1.Nodes.Insert(2, New TreeNode("I am inserted at the 3th position "))
說明:
insert()函數中,第一個參數是從0 開始
是以上面方法三中第一個參數設定是2,而結果顯示該節點插入位置為第三項
删除節點及其下所有内容
'設定樹狀圖中節點
Dim node As TreeNode
''removing is done much in the same way:
TreeView1.Nodes.Add("I need to be removed ").Nodes.Add("and all children too ")
TreeView1.Nodes(0).Name = "test"
node = TreeView1.Nodes("test")
node.Remove()
說明:
注意,使用TreeView1.Nodes()定位節點的時候,有2種方法,
第一種,參數是Index,從0開始
第二種,參數是name,而不是text.
上例中如果寫作TreeView1.Nodes("I need to be removed "),則會系統報錯,Nodes傳回值為Nothing
删除前:
删除後:
删除節點其下的所有内容(該節點保留)
'設定樹狀圖中節點
Dim node As TreeNode
''removing is done much in the same way:
TreeView1.Nodes.Add("I need to be removed ").Nodes.Add("and all children too ")
TreeView1.Nodes(0).Name = "test"
node = TreeView1.Nodes("test")
node.Nodes.Clear()
說明:
clear()方法清除的是指定節點下所屬的内容,而該指定節點不受影響
删除前:
删除後:
展開/關閉節點
'the behaviour of the treenode can be controlled completely in code.
'you can make it expand
TreeView1.Nodes(0).Expand()
''and retract again
TreeView1.Nodes(0).Collapse()