序列化就是将一個對象的狀态持久化,把目前對象的資訊儲存到檔案中,在需要時反序列化擷取該對象的資訊。
下面是序列化與反序列化的執行個體。
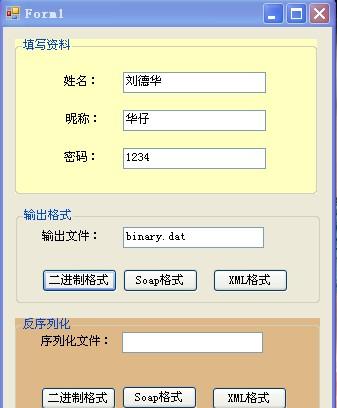
輸入如圖所示的資訊,點選輸出格式内的二進制格式按鈕,将輸入目前對象資訊的二進制格式檔案(預設儲存在目前工程的bin目錄下),在進行反序列化時,在反序列化框的TextBox内輸入binar.dat也就是已經輸出的檔案,點選二進制格式的反序列化按鈕即可獲得以上序列化後儲存的對象的資訊。
其他兩種格式的也類似。至于這三種序列化方式的差別及優缺點在下一篇日志中會用執行個體來描述的,現在先來了解如何将對象序列化吧。
下面是上圖對應得背景代碼:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using System.Runtime.Serialization;
using System.Runtime.Serialization.Formatters.Binary;
using System.IO;
using System.Xml.Serialization;
using System.Runtime.Serialization.Formatters.Soap;
namespace serialize
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void btnBinary_Click(object sender, EventArgs e)
{
//建立IFormatter接口引用,來自于BinaryFormatter類對象
IFormatter Fmt = new BinaryFormatter();
//定義成功資訊
string Success = "二進制輸出成功";
//調用UseIFormatter方法,并傳遞Fmt和Success參數
UseIFormatter(Fmt, Success);
}
private void UseIFormatter(IFormatter Fmt, string Success)
{
try
{
PersonName personName = new PersonName(this.txtName.Text, this.txtNicheng.Text, this.txtPassword.Text);
using (Stream fs = new FileStream(this.txtFileName.Text, FileMode.Create, FileAccess.Write, FileShare.None))
{
Fmt.Serialize(fs, personName);
}
MessageBox.Show(Success);
}
catch
{
}
}
[Serializable]
public class PersonName
{
[NonSerialized]
public string name;
[NonSerialized]
public string nickName;
public PersonOther po = new PersonOther();
public PersonName() { }
public PersonName(string name,string nickName,string pwd)
{
this.name = name;
this.nickName = nickName;
this.po.Password = pwd;
}
}
[Serializable]
public class PersonOther
{
string pwd;
public PersonOther() { }
public string Password
{
get
{
return pwd;
}
set
{
pwd = value;
}
}
}
private void btnXML_Click(object sender, EventArgs e)
{
PersonName pn = new PersonName(this.txtName.Text, this.txtNicheng.Text, this.txtPassword.Text);
XmlSerializer Xs = new XmlSerializer(typeof(PersonName), new Type[] { typeof(PersonOther) });
//建立Stream類型引用fs,并傳遞fn作路徑參數
using (Stream fs = new FileStream(this.txtFileName.Text, FileMode.Create, FileAccess.Write, FileShare.None))
{
Xs.Serialize(fs, pn);
}
MessageBox.Show("xml格式輸出成功。");
}
private void btnSoap_Click(object sender, EventArgs e)
{
//建立IFormatter接口引用,來自于SoapFormatter類對象
IFormatter Fmt = new SoapFormatter();
//定義成功資訊
string Success = "Soap輸出成功";
//調用UseIFormatter方法,并傳遞Fmt和Success參數
UseIFormatter(Fmt, Success);
}
private void btnDesBinary_Click(object sender, EventArgs e)//二進制反序列化的按鈕事件
{
using(FileStream fs=new FileStream(textBox1.Text,FileMode.Open))
{
IFormatter fmt = new BinaryFormatter();
PersonName person=(PersonName)fmt.Deserialize(fs);
txtName.Text = person.name;
txtPassword.Text = person.po.Password;
txtNicheng.Text = person.nickName;
}
}
private void btnDesSoap_Click(object sender, EventArgs e)//Soap的反序列化按鈕事件
{
using (FileStream fs = new FileStream(textBox1.Text.Trim(), FileMode.Open))
{
IFormatter fmt = new SoapFormatter();
PersonName person = (PersonName)fmt.Deserialize(fs);
txtName.Text = person.name;
txtNicheng.Text = person.nickName;
txtPassword.Text = person.po.Password;
}
}
private void btnDesXml_Click(object sender, EventArgs e)
{
XmlSerializer desXml = new XmlSerializer(typeof(PersonName), new Type[] { typeof(PersonOther) });
using (Stream sm = new FileStream(this.textBox1.Text.Trim(), FileMode.Open))
{
PersonName person=(PersonName) desXml.Deserialize(sm);
this.txtName.Text = person.name;
txtNicheng.Text = person.nickName;
txtPassword.Text= person.po.Password;
}
}
}
}
http://hi.baidu.com/zhaochenbo/blog/item/bfd044a7d300fb8ed0435826.html