為了使在點選Button控件時有所響應,需要給Button添加點選監聽器(ClickListener),這裡我給它添加了一個點選後完成界面跳轉的效果:
首先建立一個空工程,會自動生成原Activity的代碼
然後建立一個新的Activity界面,也就是跳轉之後的界面,建立方法如下
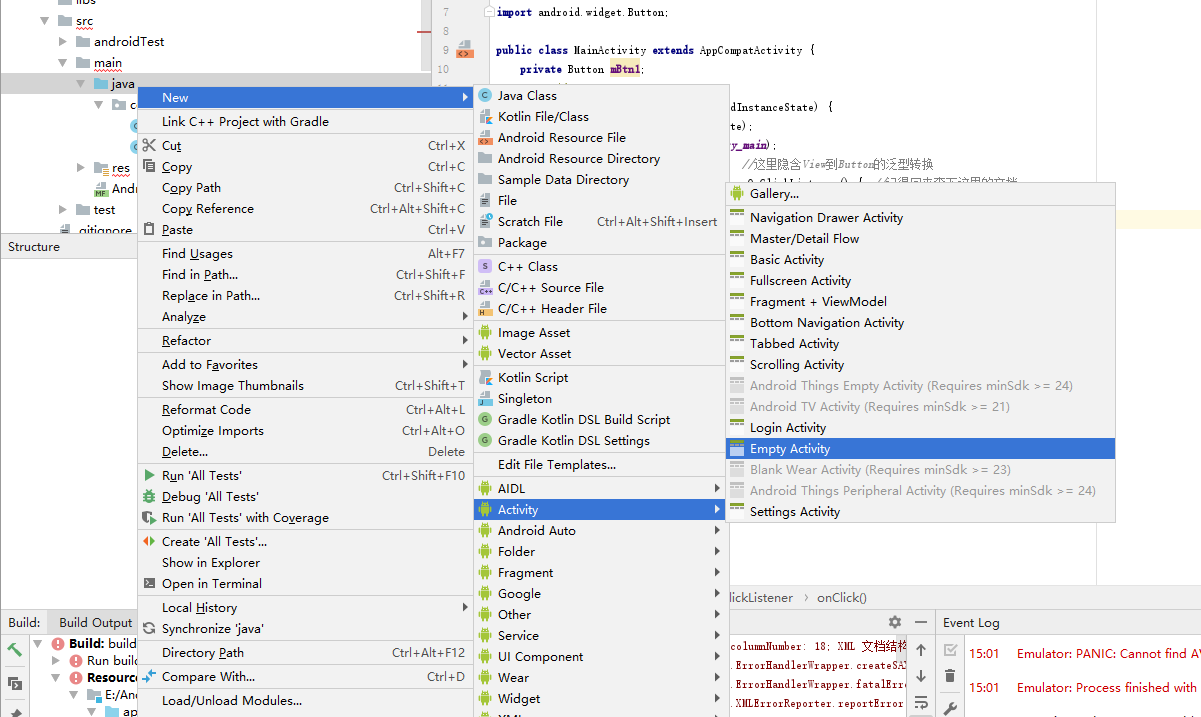
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".TextViewActivity">
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="@string/text_view_head"
android:textSize="30sp"
android:gravity="center"/>
<!--為了使得文字居中,需要設定gravity,也需要設定layoutwidth=match_parent-->
<!--text内容最好使用引用,否則會認為是"Hardcoded"而報警告-->
<ImageView
android:layout_width="wrap_content"
android:layout_height="200dp"
android:src="@drawable/dachuang" />
<TextView
android:layout_width="match_parent"
android:layout_height="200dp"
android:drawableRight="@drawable/dachuang"
android:text="@string/text_view_head"
android:textSize="30sp" />
<!--大圖檔不适合用TextView展示-->
<View
android:layout_width="match_parent"
android:layout_height="200dp"
android:background="#000000"/>
<!--View控件不含有text屬性-->
</LinearLayout>
在MainActivity的XML檔案中添加如下描述,也就是建立了一個Button的XML描述
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".MainActivity">
<Button
android:id="@+id/bt1"
android:layout_width="match_parent"
android:layout_height="80dp"
android:text="MyFirstButton"/>
<!--text預設大寫-->
</RelativeLayout>
然後在MainActivity中添加如下代碼
package com.example.demo2;
import androidx.appcompat.app.AppCompatActivity;
import android.content.Intent;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
public class MainActivity extends AppCompatActivity {
private Button mBtn1;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
mBtn1 = findViewById(R.id.bt1); //這裡隐含View到Button的泛型轉換
mBtn1.setOnClickListener(new View.OnClickListener() { //記得回來查下這裡的文檔
@Override
public void onClick(View v) {
//跳轉到TextView
Intent intent = new Intent(MainActivity.this, TextViewActivity.class);
startActivity(intent);
}
});
}
}