#include <stdio.h>
#include <stdlib.h>
#include <sys/types.h>
#include <sys/wait.h>
#include <unistd.h>
#define DELAY 4
void child_code(int delay)
{
printf("child %d here. will sleep for %d seconds\n", getpid(), delay);
sleep(delay);
printf("child done. about to exit\n");
exit(17);
}
void parent_code(int childpid)
int wait_rv=0; /* return value from wait() */
wait_rv = wait(NULL);
printf("done waiting for %d. Wait returned: %d\n",
childpid, wait_rv);
int main()
int newpid;
printf("before: mypid is %d\n", getpid());
if ( (newpid = fork()) == -1 )
perror("fork");
else if ( newpid == 0 )
child_code(DELAY);
else
parent_code(newpid);
return 0;
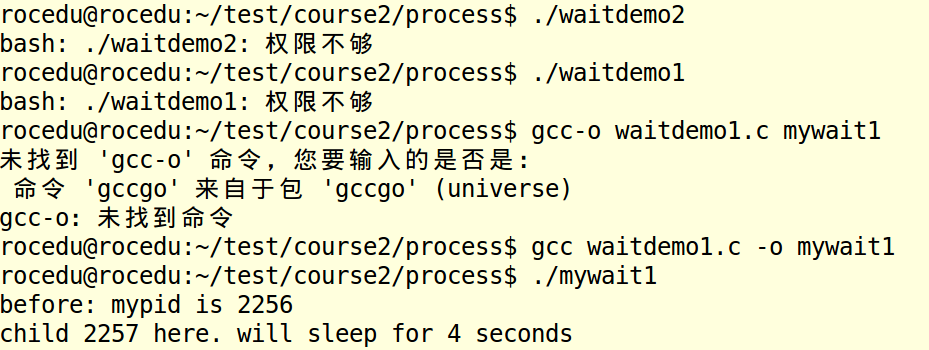
- waitdome2
#define DELAY 10
exit(27);
int wait_rv;
int child_status;
int high_8, low_7, bit_7;
wait_rv = wait(&child_status);
printf("done waiting for %d. Wait returned: %d\n", childpid, wait_rv);
high_8 = child_status >> 8; /* 1111 1111 0000 0000 */
low_7 = child_status & 0x7F; /* 0000 0000 0111 1111 */
bit_7 = child_status & 0x80; /* 0000 0000 1000 0000 */
printf("status: exit=%d, sig=%d, core=%d\n", high_8, low_7, bit_7);
printf("before: mypid is %d\n", getpid()); //程序數?
- testsystem
#include <stdlib.h> //功能貌似是一次執行兩個指令
int main ( int argc, char *argv[] )
system(argv[1]);
system(argv[2]);
return EXIT_SUCCESS;
} /* ---------- end of function main ---------- */
- testpp
#include <stdio.h>
#include <stdlib.h>
char **pp;
pp[0] = malloc(20); //向記憶體申請通路空間,運作出錯就是因為配置設定空間出問題了
- testpid
#include <unistd.h>
#include <sys/types.h>
printf("my pid: %d \n", getpid());
printf("my parent's pid: %d \n", getppid());
- testbuf1
printf("hello");
fflush(stdout); //清空stdout的緩沖區
while(1);
- testbuf2
printf("hello\n"); //和上一個代碼相比少了清緩沖
- testbuf3
fprintf(stdout, "1234", 5);
fprintf(stderr, "abcd", 4);
- psh1
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAXARGS 20
#define ARGLEN 100
int execute( char *arglist[] )
execvp(arglist[0], arglist);
perror("execvp failed");
exit(1);
char * makestring( char *buf )
char *cp;
buf[strlen(buf)-1] = '\0';
cp = malloc( strlen(buf)+1 );
if ( cp == NULL ){
fprintf(stderr,"no memory\n");
exit(1);
}
strcpy(cp, buf);
return cp;
char *arglist[MAXARGS+1];
int numargs;
char argbuf[ARGLEN];
numargs = 0;
while ( numargs < MAXARGS )
{
printf("Arg[%d]? ", numargs);
if ( fgets(argbuf, ARGLEN, stdin) && *argbuf != '\n' )
arglist[numargs++] = makestring(argbuf);
else
{
if ( numargs > 0 ){
arglist[numargs]=NULL;
execute( arglist );
numargs = 0;
}
}
return 0;